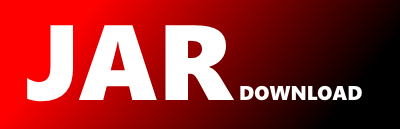
com.github.mvysny.kaributesting.v10.ComboBox.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of karibu-testing-v10 Show documentation
Show all versions of karibu-testing-v10 Show documentation
Karibu Testing, support for browserless Vaadin testing in Kotlin
package com.github.mvysny.kaributesting.v10
import com.vaadin.flow.component.combobox.ComboBox
import com.vaadin.flow.component.select.Select
import com.vaadin.flow.data.provider.DataCommunicator
import com.vaadin.flow.function.SerializableConsumer
/**
* Emulates an user inputting something into the combo box, filtering items. You can use [getSuggestionItems]
* to retrieve those items and to verify that the filter on your data provider works properly.
*
* WARNING: Only works with Vaadin 12 or higher
*/
fun ComboBox.setUserInput(userInput: String?) {
check(ComboBox::class.java.declaredFields.any { it.name == "filterSlot" }) { "This function only works with Vaadin 12 or higher" }
val comboBoxFilterSlot = ComboBox::class.java.getDeclaredField("filterSlot").apply { isAccessible = true }
@Suppress("UNCHECKED_CAST")
(comboBoxFilterSlot.get(this) as SerializableConsumer).accept(userInput)
}
/**
* Fetches items currently displayed in the suggestion box.
*
* WARNING: Only works with Vaadin 12 or higher
*/
@Suppress("UNCHECKED_CAST")
fun ComboBox.getSuggestionItems(): List {
check(ComboBox::class.java.declaredFields.any { it.name == "dataCommunicator" }) { "This function only works with Vaadin 12 or higher" }
val field = ComboBox::class.java.getDeclaredField("dataCommunicator").apply { isAccessible = true }
val dataCommunicator = field.get(this) as DataCommunicator
return dataCommunicator.fetch(0, Int.MAX_VALUE)
}
/**
* Fetches captions of items currently displayed in the suggestion box.
*
* WARNING: Only works with Vaadin 12 or higher
*/
fun ComboBox.getSuggestions(): List {
val items = getSuggestionItems()
return items.map { itemLabelGenerator.apply(it) }
}
/**
* Fetches items currently displayed in the suggestion box.
*/
@Suppress("UNCHECKED_CAST")
fun Select.getSuggestionItems(): List = dataProvider._findAll()
/**
* Fetches captions of items currently displayed in the suggestion box.
*/
fun Select.getSuggestions(): List {
val items = getSuggestionItems()
return when (itemLabelGenerator) {
null -> items.map { it.toString() }
else -> items.map { itemLabelGenerator.apply(it) }
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy