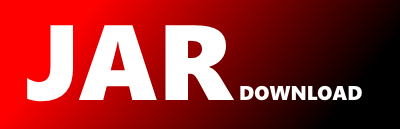
com.github.mvysny.kaributesting.v10.ContextMenu.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of karibu-testing-v10 Show documentation
Show all versions of karibu-testing-v10 Show documentation
Karibu Testing, support for browserless Vaadin testing in Kotlin
package com.github.mvysny.kaributesting.v10
import com.vaadin.flow.component.ClickEvent
import com.vaadin.flow.component.Component
import com.vaadin.flow.component.contextmenu.ContextMenu
import com.vaadin.flow.component.contextmenu.ContextMenuBase
import com.vaadin.flow.component.contextmenu.MenuItem
import com.vaadin.flow.component.contextmenu.MenuItemBase
import com.vaadin.flow.component.grid.Grid
import com.vaadin.flow.component.grid.contextmenu.GridContextMenu
import com.vaadin.flow.component.grid.contextmenu.GridMenuItem
import com.vaadin.flow.dom.DomEvent
import elemental.json.impl.JreJsonFactory
import kotlin.test.expect
import kotlin.test.fail
/**
* Tries to find a menu item with given caption and click it.
* @throws AssertionError if no such menu item exists, or the menu item is not enabled or visible, or it's nested in
* a menu item which is invisible or disabled, or it's attached to a component that's invisible.
*/
fun ContextMenu._clickItemWithCaption(caption: String) {
val parentMap = getParentMap()
val item = parentMap.keys.firstOrNull { it.getText() == caption } ?: fail("No menu item with caption $caption in ${toPrettyTree()}")
(item as MenuItem)._click()
}
/**
* Tries to find a menu item with given caption and click it, passing in given [gridItem].
* @throws AssertionError if no such menu item exists, or the menu item is not enabled or visible, or it's nested in
* a menu item which is invisible or disabled, or it's attached to a component that's invisible.
*/
fun GridContextMenu._clickItemWithCaption(caption: String, gridItem: T?) {
val parentMap = getParentMap()
val item = parentMap.keys.firstOrNull { it.getText() == caption } ?: fail("No menu item with caption $caption in ${toPrettyTree()}")
@Suppress("UNCHECKED_CAST")
(item as GridMenuItem)._click(gridItem)
}
private fun ContextMenuBase<*, *, *>.getParentMap(): Map, Component> {
val result = mutableMapOf, Component>()
fun fillInParentFor(item: MenuItemBase<*, *, *>, parent: Component) {
result[item] = parent
item.getSubMenu().getItems().forEach { fillInParentFor(it, item) }
}
getItems().forEach { fillInParentFor(it, this) }
return result
}
/**
* Tries to click given menu item.
* @throws AssertionError if no such menu item exists, or the menu item is not enabled or visible, or it's nested in
* a menu item which is invisible or disabled, or it's attached to a component that's invisible.
*/
fun MenuItem._click() {
val parentMap = contextMenu.getParentMap()
checkMenuItemVisible(this, parentMap)
checkMenuItemEnabled(this, parentMap)
_fireEvent(ClickEvent
© 2015 - 2025 Weber Informatics LLC | Privacy Policy