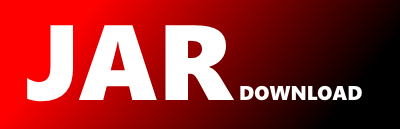
com.github.mvysny.kaributesting.v10.PrettyPrintTree.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of karibu-testing-v10 Show documentation
Show all versions of karibu-testing-v10 Show documentation
Karibu Testing, support for browserless Vaadin testing in Kotlin
package com.github.mvysny.kaributesting.v10
import com.vaadin.flow.component.Component
import com.vaadin.flow.component.HasValue
import com.vaadin.flow.component.contextmenu.MenuItemBase
import com.vaadin.flow.component.grid.Grid
import java.util.*
import kotlin.streams.toList
/**
* If true, [PrettyPrintTree] will use `\--` instead of `└──` which tend to render on some terminals as `???`.
*/
var prettyPrintUseAscii: Boolean = false
/**
* Utility class to create a pretty-printed ASCII tree of arbitrary nodes that can be printed to the console.
* You can build the tree out of any tree structure, just fill in this node [name] and its [children].
*
* To create a pretty tree dump of a Vaadin component, just use [ofVaadin].
*/
class PrettyPrintTree(val name: String, val children: MutableList) {
private val pipe = if (!prettyPrintUseAscii) '│' else '|'
private val branchTail = if (!prettyPrintUseAscii) "└── " else "\\-- "
private val branch = if (!prettyPrintUseAscii) "├── " else "|-- "
fun print(): String {
val sb = StringBuilder()
print(sb, "", true)
return sb.toString()
}
private fun print(sb: StringBuilder, prefix: String, isTail: Boolean) {
sb.append(prefix + (if (isTail) branchTail else branch) + name + "\n")
for (i in 0 until children.size - 1) {
children[i].print(sb, prefix + if (isTail) " " else "$pipe ", false)
}
if (children.size > 0) {
children[children.size - 1]
.print(sb, prefix + if (isTail) " " else "$pipe ", true)
}
}
companion object {
fun ofVaadin(root: Component): PrettyPrintTree {
val result = PrettyPrintTree(root.toPrettyString(), mutableListOf())
for (child in root.children) {
result.children.add(ofVaadin(child))
}
if (root is MenuItemBase<*, *, *>) {
for (item in root.getSubMenu().getItems()) {
result.children.add(ofVaadin(item))
}
}
return result
}
}
}
fun Component.toPrettyTree(): String = PrettyPrintTree.ofVaadin(this).print()
/**
* Returns the most basic properties of the component, formatted as a concise string:
* * The component class
* * The [Component.getId]
* * Whether the component is [Component.isVisible]
* * Whether it is a [HasValue] that is read-only
* * the styles
* * The [Component.label] and text
* * The [HasValue.getValue]
*/
@Suppress("UNCHECKED_CAST")
fun Component.toPrettyString(): String {
val list = LinkedList()
if (id.isPresent) {
list.add("#${id.get()}")
}
if (!_isVisible) {
list.add("INVIS")
}
if (this is HasValue<*, *> && (this as HasValue, Any?>).isReadOnly) {
list.add("RO")
}
if (!isEnabled) {
list.add("DISABLED")
}
if (element.style.names.toList().isNotEmpty()) {
list.add(element.style.names.toList().joinToString(" ") { "$it:${element.style[it]}" })
}
if (label.isNotBlank()) {
list.add("label='$label'")
}
if (!_text.isNullOrBlank()) {
list.add("text='$_text'")
}
if (this is HasValue<*, *>) {
list.add("value='${(this as HasValue, Any?>).value}'")
}
if (this is Grid.Column<*> && this.header2.isNotBlank()) {
list.add("header='${this.header2}'")
}
if (!placeholder.isNullOrBlank()) {
list.add("placeholder='$placeholder'")
}
var name = javaClass.simpleName
if (name.isEmpty()) {
// anonymous classes
name = javaClass.name
}
return name + list
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy