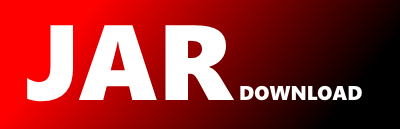
org.supercsv.ext.builder.BooleanCellProcessorBuilder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of super-csv-annotation Show documentation
Show all versions of super-csv-annotation Show documentation
CSVのJavaライブラリであるSuperCSVに、アノテーション機能を追加したライブラリです。
Please wait ...
© 2015 - 2025 Weber Informatics LLC | Privacy Policy