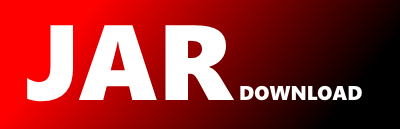
com.github.naoghuman.lib.preferences.api.ILibPreferences Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of lib-preferences Show documentation
Show all versions of lib-preferences Show documentation
Lib-Preferences is a library for easy storing simple data in a Preferences.properties file in a JavaFx & Maven desktop application. See https://github.com/Naoghuman/lib-preferences for more details.
/*
* Copyright (C) 2014 PRo
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program. If not, see .
*/
package com.github.naoghuman.lib.preferences.api;
import java.util.prefs.Preferences;
/**
* The Interface
for the class {@link com.github.naoghuman.lib.preferences.LibPreferences}.
* Over the facade {@link com.github.naoghuman.lib.preferences.api.PreferencesFacade} you can
* access the methods in this Interface
.
*
* @author PRo
* @see com.github.naoghuman.lib.preferences.LibPreferences
* @see com.github.naoghuman.lib.preferences.api.PreferencesFacade
*/
@Deprecated
public interface ILibPreferences {
/**
* Key constant for accessing the system preferfence file.
*/
@Deprecated
public static final String SYSTEM_PREFERENCES__FILE = "SYSTEM_PREFERENCES__FILE"; // NOI18N
/**
* Default value contant for the system preferfence file.
*/
@Deprecated
public static final String SYSTEM_PREFERENCES__FILE_DEFAULT_VALUE = "Preferences.properties"; // NOI18N
/**
* Get a {@link java.lang.Boolean} which is associated with the key in application context.
*
* @param key The key which value is searched for.
* @param def The default value if the key isn't stored.
* @return The Boolean
which is associated with the key or the default value.
*/
@Deprecated
public Boolean getBoolean(String key, Boolean def);
/**
* Get a {@link java.lang.Boolean} which is associated with the key in module context.
*
* @param clazz Defined the module context.
* @param key The key which value is searched for.
* @param def The default value if the key isn't stored.
* @return The Boolean
which is associated with the key or the default value.
*/
@Deprecated
public Boolean getBoolean(Class clazz, String key, Boolean def);
/**
* Stores a {@link java.lang.Boolean} which is associated with the key in application context.
*
* @param key The key for the saved Boolean
.
* @param value The value which will associated with the key.
*/
@Deprecated
public void putBoolean(String key, Boolean value);
/**
* Stores a {@link java.lang.Boolean} which is associated with the key in module context.
*
* @param clazz Defined the module context.
* @param key The key for the to saved Boolean
.
* @param value The value which will associated with the key.
*/
@Deprecated
public void putBoolean(Class clazz, String key, Boolean value);
/**
* Get a {@link java.lang.String} which is associated with the key in application context.
*
* @param key The key which value is searched for.
* @param def The default value if the key isn't stored.
* @return The String
which is associated with the key or the default value.
*/
@Deprecated
public String get(String key, String def);
/**
* Get a {@link java.lang.String} which is associated with the key in module context.
*
* @param clazz Defined the module context.
* @param key The key which value is searched for.
* @param def The default value if the key isn't stored.
* @return The String
which is associated with the key or the default value.
*/
@Deprecated
public String get(Class clazz, String key, String def);
/**
* Stores a {@link java.lang.String} which is associated with the key in application context.
*
* @param key The key for the saved String
.
* @param value The value which will associated with the key.
*/
@Deprecated
public void put(String key, String value);
/**
* Stores a {@link java.lang.String} which is associated with the key in module context.
*
* @param clazz Defined the module context.
* @param key The key for the to saved String
.
* @param value The value which will associated with the key.
*/
@Deprecated
public void put(Class clazz, String key, String value);
/**
* Get a {@link java.lang.Double} which is associated with the key in application context.
*
* @param key The key which value is searched for.
* @param def The default value if the key isn't stored.
* @return The Double
which is associated with the key or the default value.
*/
@Deprecated
public Double getDouble( String key, Double def);
/**
* Get a {@link java.lang.Double} which is associated with the key in module context.
*
* @param clazz Defined the module context.
* @param key The key which value is searched for.
* @param def The default value if the key isn't stored.
* @return The Double
which is associated with the key or the default value.
*/
@Deprecated
public Double getDouble(Class clazz, String key, Double def);
/**
* Stores a {@link java.lang.Double} which is associated with the key in application context.
*
* @param key The key for the saved Double
.
* @param value The value which will associated with the key.
*/
@Deprecated
public void putDouble(String key, Double value);
/**
* Stores a {@link java.lang.Double} which is associated with the key in module context.
*
* @param clazz Defined the module context.
* @param key The key for the to saved Double
.
* @param value The value which will associated with the key.
*/
@Deprecated
public void putDouble(Class clazz, String key, Double value);
/**
* Get a {@link java.lang.Integer} which is associated with the key in application context.
*
* @param key The key which value is searched for.
* @param def The default value if the key isn't stored.
* @return The Integer
which is associated with the key or the default value.
*/
@Deprecated
public Integer getInt(String key, Integer def);
/**
* Get a {@link java.lang.Integer} which is associated with the key in module context.
*
* @param clazz Defined the module context.
* @param key The key which value is searched for.
* @param def The default value if the key isn't stored.
* @return The Integer
which is associated with the key or the default value.
*/
@Deprecated
public Integer getInt(Class clazz, String key, Integer def);
/**
* Stores a {@link java.lang.Integer} which is associated with the key in application context.
*
* @param key The key for the saved Integer
.
* @param value The value which will associated with the key.
*/
@Deprecated
public void putInt(String key, Integer value);
/**
* Stores a {@link java.lang.Integer} which is associated with the key in module context.
*
* @param clazz Defined the module context.
* @param key The key for the to saved Integer
.
* @param value The value which will associated with the key.
*/
@Deprecated
public void putInt(Class clazz, String key, Integer value);
/**
* Get a {@link java.lang.Long} which is associated with the key in application context.
*
* @param key The key which value is searched for.
* @param def The default value if the key isn't stored.
* @return The Long
which is associated with the key or the default value.
*/
@Deprecated
public Long getLong(String key, Long def);
/**
* Get a {@link java.lang.Long} which is associated with the key in module context.
*
* @param clazz Defined the module context.
* @param key The key which value is searched for.
* @param def The default value if the key isn't stored.
* @return The Long
which is associated with the key or the default value.
*/
@Deprecated
public Long getLong(Class clazz, String key, Long def);
/**
* Stores a {@link java.lang.Long} which is associated with the key in application context.
*
* @param key The key for the saved Long
.
* @param value The value which will associated with the key.
*/
@Deprecated
public void putLong(String key, Long value);
/**
* Stores a {@link java.lang.Long} which is associated with the key in module context.
*
* @param clazz Defined the module context.
* @param key The key for the to saved Long
.
* @param value The value which will associated with the key.
*/
@Deprecated
public void putLong(Class clazz, String key, Long value);
/**
* Initialize the Preferences.properties
file.
* That means that the Preferences.properties
file will created
* under System.getProperty("user.dir") + File.separator
* + "Preferences.properties"
.
*
* @param drop Should an existing file dropped at frist?
*/
@Deprecated
public void init(boolean drop);
/**
* Allowed access to the Preferences
in application context. You can
* for example add a {@link java.util.prefs.PreferenceChangeListener PreferenceChangeListener}
* to listen for changes in specific preferences.
*
* @return The Preferences
in application context.
*/
@Deprecated
public Preferences forApplication();
/**
* Allowed access to the Preferences
in module context. You can
* for example add a {@link java.util.prefs.PreferenceChangeListener PreferenceChangeListener}
* to listen for changes in specific preferences.
*
* @param clazz The class in which defined the module.
* @return The Preferences
in module context.
*/
@Deprecated
public Preferences forModule(Class clazz);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy