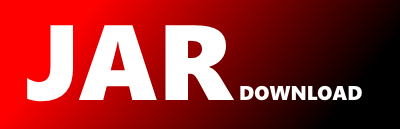
com.github.napp.database.impl.DAO Maven / Gradle / Ivy
/**
*
*/
package com.github.napp.database.impl;
import java.sql.Connection;
import java.sql.SQLException;
import com.github.napp.database.IConnectionProvider;
import com.github.napp.database.IDAO;
import com.github.napp.database.IDeleteQuery;
import com.github.napp.database.IEntity;
import com.github.napp.database.IFreeQuery;
import com.github.napp.database.IInsertQuery;
import com.github.napp.database.ISelectQuery;
import com.github.napp.database.IUpdateQuery;
import com.github.napp.util.NullTool;
/**
* @author Alexandru Bledea
* @since Sep 21, 2013
*/
public abstract class DAO implements IDAO {
private final EntityMapper entityMapper;
private final String tableName;
private final IConnectionProvider connectionProvider;
/**
* @param clazz
* @param tableName
* @throws SQLException
*/
public DAO(Class clazz, String tableName) throws SQLException {
this(clazz, tableName, null);
}
/**
* @param entityClass
* @param tableName
* @param connectionProvider
* @throws SQLException
*/
public DAO(Class entityClass, final String tableName, IConnectionProvider connectionProvider) throws SQLException {
NullTool.forbidNull(entityClass, tableName);
this.tableName = tableName;
this.connectionProvider = connectionProvider;
entityMapper = new EntityMapper(this, entityClass);
}
/* (non-Javadoc)
* @see com.github.napp.database.DAO#select()
*/
@Override
public final ISelectQuery select() {
return new SelectQuery(this);
}
/* (non-Javadoc)
* @see com.github.napp.database.IDAO#insert()
*/
@Override
public final IInsertQuery insert() {
return new InsertQuery(this);
}
/* (non-Javadoc)
* @see com.github.napp.database.IDAO#update()
*/
@Override
public IUpdateQuery update() {
return update((Integer) null);
}
/* (non-Javadoc)
* @see com.github.napp.database.IDAO#update(com.github.napp.database.IEntity)
*/
@Override
public IUpdateQuery update(I entity) {
if (entity == null) {
throw new IllegalArgumentException("Entity is null");
}
return update(entity.getId());
}
/* (non-Javadoc)
* @see com.github.napp.database.IDAO#update(java.lang.Integer)
*/
@Override
public IUpdateQuery update(Integer id) {
UpdateQuery updateQuery = new UpdateQuery(this);
if (id != null) {
updateQuery.addEquals("id", id);
}
return updateQuery;
}
/* (non-Javadoc)
* @see com.github.napp.database.DAO#delete()
*/
@Override
public final IDeleteQuery delete() {
return new DeleteQuery(this);
}
/* (non-Javadoc)
* @see com.github.napp.database.IDAO#delete(com.github.napp.database.IEntity)
*/
@Override
public void delete(I entity) {
NullTool.forbidNull(entity, "No entity provided!");
delete(entity.getId());
};
/* (non-Javadoc)
* @see com.github.napp.database.IDAO#delete(int)
*/
@Override
public void delete(int id) {
IDeleteQuery delete = delete();
delete.addEquals("id", id);
delete.execute();
}
/* (non-Javadoc)
* @see com.github.napp.database.DAO#freeQuery()
*/
@Override
public final IFreeQuery freeQuery() {
return new FreeQuery(this);
}
/**
* @param property
*/
final void validateProperty(String property) {
entityMapper.validate(property);
}
/**
* @return
*/
protected final Connection openConnection() {
NullTool.forbidNull(connectionProvider);
Connection connection = connectionProvider.getConnection();
NullTool.forbidNull(connection);
return connection;
}
/**
* @param con
*/
protected final void closeConnection(Connection con) {
NullTool.forbidNull(connectionProvider);
connectionProvider.close(con);
}
/**
* @return
*/
protected final String getTableName() {
return tableName;
}
/**
* @return the entityMapper
*/
protected final EntityMapper getEntityMapper() {
return entityMapper;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy