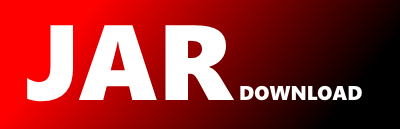
com.github.ndrlslz.service.ServiceCallAdapterFactory Maven / Gradle / Ivy
package com.github.ndrlslz.service;
import com.github.ndrlslz.exception.HttpException;
import retrofit2.*;
import java.io.IOException;
import java.lang.annotation.Annotation;
import java.lang.reflect.ParameterizedType;
import java.lang.reflect.Type;
public class ServiceCallAdapterFactory extends CallAdapter.Factory {
public static ServiceCallAdapterFactory create() {
return new ServiceCallAdapterFactory();
}
private ServiceCallAdapterFactory() {
}
@Override
public CallAdapter> get(Type returnType, Annotation[] annotations, Retrofit retrofit) {
if (getRawType(returnType) != ServiceCall.class) {
return null;
}
if (!(returnType instanceof ParameterizedType)) {
throw new IllegalStateException("ServiceCall return type must be parameterized"
+ " as ServiceCall or ServiceCall extends Foo>");
}
Type innerType = getParameterUpperBound(0, (ParameterizedType) returnType);
if (getRawType(innerType) != Response.class) {
// Generic type is not Response. Use it for body-only adapter.
return new BodyCallAdapter(innerType);
}
// Generic type is Response. Extract T and create the Response version of the adapter.
if (!(innerType instanceof ParameterizedType)) {
throw new IllegalStateException("Response must be parameterized"
+ " as Response or Response extends Foo>");
}
Type responseType = getParameterUpperBound(0, (ParameterizedType) innerType);
return new ResponseCallAdapter(responseType);
}
private static class BodyCallAdapter implements CallAdapter> {
private final Type responseType;
BodyCallAdapter(Type responseType) {
this.responseType = responseType;
}
@Override
public Type responseType() {
return responseType;
}
@Override
public ServiceCall adapt(final Call call) {
return new ServiceCall() {
@Override
public R execute() throws IOException {
return call.execute().body();
}
@Override
public void enqueue(final ServiceCallback callback) {
call.enqueue(new Callback() {
@Override
public void onResponse(Call call, Response response) {
callback.onResponse(response.body());
}
@Override
public void onFailure(Call call, Throwable t) {
callback.onFailure((HttpException) t);
}
});
}
@Override
public void cancel() {
call.cancel();
}
};
}
}
private static class ResponseCallAdapter implements CallAdapter> {
private final Type responseType;
ResponseCallAdapter(Type responseType) {
this.responseType = responseType;
}
@Override
public Type responseType() {
return responseType;
}
@Override
public ServiceCall> adapt(final Call call) {
return new ServiceCall>() {
@Override
public Response execute() throws IOException {
return call.execute();
}
@Override
public void enqueue(final ServiceCallback> callback) {
call.enqueue(new Callback() {
@Override
public void onResponse(Call call, Response response) {
callback.onResponse(response);
}
@Override
public void onFailure(Call call, Throwable t) {
callback.onFailure((HttpException) t);
}
});
}
@Override
public void cancel() {
call.cancel();
}
};
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy