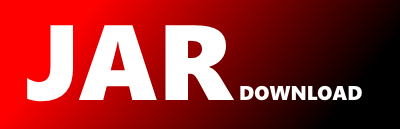
com.github.ngoanh2n.YamlData Maven / Gradle / Ivy
package com.github.ngoanh2n;
import com.fasterxml.jackson.databind.ObjectMapper;
import org.yaml.snakeyaml.Yaml;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.lang.reflect.ParameterizedType;
import java.lang.reflect.Type;
import java.lang.reflect.TypeVariable;
import java.nio.charset.Charset;
import java.util.*;
/**
* Reads Yaml file to Map, list of Map, Model, list of Models.
* Class of Model must be `public` and has `setter` methods.
*
* @author Ho Huu Ngoan ([email protected])
*/
@SuppressWarnings("unchecked")
public abstract class YamlData {
private InputStream _inputStream;
private Class _modelClazz;
/**
* Constructs and gets current Java Bean class
*/
public YamlData() {
_modelClazz = getModelClazz();
}
//-------------------------------------------------------------------------------//
/**
* Reads Yaml file as {@linkplain Map}.
*
* @param name is the name of file.
* e.g. src/test/resources/com/foo/File.yml
* @return {@linkplain Map} if the file exists;
* {@linkplain RuntimeError } if the file doesn't exist or read multiple object as single object.
*/
public static Map toMapFromFile(String name) {
return toMapFromFile(name, Charset.defaultCharset());
}
/**
* Reads Yaml file as {@linkplain Map}.
*
* @param name is the name of file.
* e.g. src/test/resources/com/foo/File.yml
* @param cs is a Charset
* @return {@linkplain Map} if the file exists;
* {@linkplain RuntimeError } if the file doesn't exist or read multiple object as single object.
*/
public static Map toMapFromFile(String name, Charset cs) {
InputStream is = getInputStream(name);
return toMapFromInputStream(is, cs);
}
/**
* Reads Yaml file as {@linkplain Map}.
*
* @param name is the name of resource.
* e.g. com/foo/File.yml
* @return {@linkplain Map} if the file exists;
* {@linkplain RuntimeError } if the file doesn't exist or read multiple object as single object.
*/
public static Map toMapFromResource(String name) {
return toMapFromResource(name, Charset.defaultCharset());
}
/**
* Reads Yaml file as {@linkplain Map}.
*
* @param name is the name of resource.
* e.g. com/foo/File.yml
* @param cs is a Charset
* @return {@linkplain Map} if the file exists;
* {@linkplain RuntimeError } if the file doesn't exist or read multiple object as single object.
*/
public static Map toMapFromResource(String name, Charset cs) {
InputStream is = Resource.getInputStream(name);
return toMapFromInputStream(is, cs);
}
/**
* Reads Yaml file as {@linkplain List} of {@linkplain Map}.
*
* @param name is the name of file.
* e.g. com/foo/File.yml
* @return {@linkplain List} of {@linkplain Map} if the file exists;
* {@linkplain RuntimeError } otherwise.
*/
public static List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy