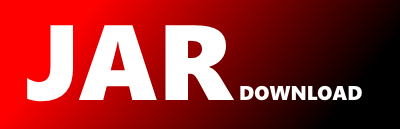
com.github.nicosensei.elasticindexbatch.IndexBatch Maven / Gradle / Ivy
package com.github.nicosensei.elasticindexbatch;
import java.util.HashMap;
import java.util.Map;
import org.elasticsearch.client.transport.TransportClient;
import org.elasticsearch.common.settings.ImmutableSettings;
import org.elasticsearch.common.transport.InetSocketTransportAddress;
import com.github.nicosensei.textbatch.Tool;
import com.github.nicosensei.textbatch.ToolException;
import com.github.nicosensei.textbatch.job.InputFileException;
import com.github.nicosensei.textbatch.job.InputLine;
import com.github.nicosensei.textbatch.job.JobController;
/**
* @author ngiraud
*
*/
public abstract class IndexBatch<
I extends InputLine, D extends IndexableDocument, J extends IndexBatchJob>
extends JobController {
private static final String ES_PING_TIMEOUT = Tool.getInstance().getProperty(
IndexBatch.class, "esPingTimeout");
private static final String[] ES_NODES_ADRESSES = Tool.getInstance().getProperty(
IndexBatch.class, "esNodesAdresses").split(",");
private static final String ES_CLUSTER_NAME = Tool.getInstance().getProperty(
IndexBatch.class, "esClusterName");
protected static final int SKIP_LIMIT = Tool.getInstance().getIntProperty(
IndexBatch.class, "skipLimit", 1000);
private TransportClient client;
@Override
protected abstract J jobFactory() throws ToolException;
@Override
protected IndexBatchState jobProgressFactory() throws ToolException {
IndexBatchState p = new IndexBatchState(getInputFilePath());
getInputFile().addCooldownListener(p);
return p;
}
@Override
public IndexBatchState getProgress() {
return (IndexBatchState) super.getProgress();
}
/**
* @return the client
*/
protected final TransportClient getClient() {
return client;
}
/**
* @return the indexName
*/
protected abstract String getIndexName();
@Override
protected abstract BatchInputReader inputFileReaderFactory()
throws InputFileException;
@Override
public BatchInputReader getInputFile() {
return (BatchInputReader) super.getInputFile();
}
protected abstract String getInputFilePath();
protected abstract void specificInit(String[] args) throws ToolException;
@Override
protected final void init(String[] args) throws ToolException {
specificInit(args);
Tool tool = Tool.getInstance();
Map clientSettings = new HashMap<>();
clientSettings.put("cluster.name", ES_CLUSTER_NAME);
if (ES_PING_TIMEOUT != null && !ES_PING_TIMEOUT.isEmpty()) {
clientSettings.put("client.transport.ping_timeout", ES_PING_TIMEOUT);
}
tool.logInfo("Cluster name is '" + ES_CLUSTER_NAME + "'");
this.client = new TransportClient(ImmutableSettings.settingsBuilder().put(clientSettings));
for (String esta : ES_NODES_ADRESSES) {
String[] parts = esta.split(":");
this.client.addTransportAddress(new InetSocketTransportAddress(
parts[0], Integer.parseInt(parts[1])));
tool.logInfo("Registered node address " + esta);
}
tool.logInfo("Target index name is " + getIndexName());
}
@Override
protected void onComplete() throws ToolException {
Tool tool = Tool.getInstance();
tool.logInfo("Will shut down ElasticSearch client...");
this.client.close();
tool.logInfo("... done!");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy