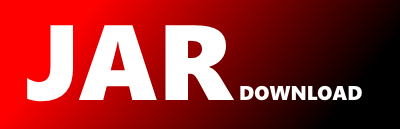
de.lessvoid.nifty.examples.defaultcontrols.ControlsDemoScreenController Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of nifty-examples Show documentation
Show all versions of nifty-examples Show documentation
Lots of Nifty example code! You can find the source for nearly all demos/tutorials in here.
package de.lessvoid.nifty.examples.defaultcontrols;
import de.lessvoid.nifty.EndNotify;
import de.lessvoid.nifty.Nifty;
import de.lessvoid.nifty.NiftyEventSubscriber;
import de.lessvoid.nifty.controls.*;
import de.lessvoid.nifty.controls.ConsoleCommands.ConsoleCommand;
import de.lessvoid.nifty.effects.Effect;
import de.lessvoid.nifty.effects.EffectEventId;
import de.lessvoid.nifty.effects.impl.Move;
import de.lessvoid.nifty.elements.Element;
import de.lessvoid.nifty.elements.events.NiftyMousePrimaryClickedEvent;
import de.lessvoid.nifty.examples.resolution.ResolutionControl;
import de.lessvoid.nifty.input.NiftyInputEvent;
import de.lessvoid.nifty.input.NiftyStandardInputEvent;
import de.lessvoid.nifty.screen.KeyInputHandler;
import de.lessvoid.nifty.screen.Screen;
import de.lessvoid.nifty.screen.ScreenController;
import de.lessvoid.nifty.tools.Color;
import javax.annotation.Nonnull;
import javax.annotation.Nullable;
import java.util.*;
import java.util.logging.Logger;
public class ControlsDemoScreenController implements ScreenController, KeyInputHandler {
private static final Logger logger = Logger.getLogger(ControlsDemoScreenController.class.getName());
private static final Color HELP_COLOR = new Color("#aaaf");
private Nifty nifty;
private Screen screen;
@Nullable
private Element consolePopup;
@Nullable
private Element creditsPopup;
@Nullable
private Console console;
@Nullable
private ConsoleCommands consoleCommands;
private final ResolutionControl resControl;
// This simply maps the IDs of the MenuButton elements to the corresponding Dialog elements we'd
// like to show with the given MenuButton. This map will make our life a little bit easier when
// switching between the menus.
@Nonnull
private final Map buttonToDialogMap = new HashMap();
// We keep all the button IDs in this list so that we can later decide if an index is before or
// after the current button.
@Nonnull
private final List buttonIdList = new ArrayList();
// This keeps the current menu button
@Nullable
private String currentMenuButtonId;
public ControlsDemoScreenController(final ResolutionControl resolutionControl, @Nullable final String ... mapping) {
resControl = resolutionControl;
if (mapping == null || mapping.length == 0 || mapping.length % 2 != 0) {
logger.warning("expecting pairs of values that map menuButton IDs to dialog IDs");
} else {
for (int i=0; i moveEffects = element.findElementById("#effectPanel").getEffects(effectEventId, Move.class);
if (!moveEffects.isEmpty()) {
moveEffects.get(0).getParameters().put("direction", direction);
}
}
@NiftyEventSubscriber(pattern="menuButton.*")
public void onMenuButtonListBoxClick(@Nonnull final String id, final NiftyMousePrimaryClickedEvent clickedEvent) {
if ("menuButtonCredits".equals(id)) {
showCredits();
return;
}
changeDialogTo(id);
}
private void showCredits() {
nifty.showPopup(screen, creditsPopup.getId(), null);
}
@NiftyEventSubscriber(id="creditsBack")
public void onCreditsBackClick(final String id, final ButtonClickedEvent event) {
nifty.closePopup(creditsPopup.getId());
}
@NiftyEventSubscriber(id="resetScreenButton")
public void onTestButtonClick(final String id, final ButtonClickedEvent clickedEvent) {
screen.findElementById(buttonToDialogMap.get(currentMenuButtonId)).hide(new EndNotify() {
@Override
public void perform() {
nifty.gotoScreen("demo");
}
});
}
private void changeDialogTo(@Nonnull final String id) {
if (!id.equals(currentMenuButtonId)) {
int currentIndex = buttonIdList.indexOf(currentMenuButtonId);
int nextIndex = buttonIdList.indexOf(id);
Element nextElement = screen.findElementById(buttonToDialogMap.get(id));
modifyMoveEffect(EffectEventId.onShow, nextElement, currentIndex < nextIndex ? "right" : "left");
nextElement.show();
Element currentElement = screen.findElementById(buttonToDialogMap.get(currentMenuButtonId));
modifyMoveEffect(EffectEventId.onHide, currentElement, currentIndex < nextIndex ? "left" : "right");
currentElement.hide();
screen.findElementById(currentMenuButtonId).stopEffect(EffectEventId.onCustom);
screen.findElementById(id).startEffect(EffectEventId.onCustom, null, "selected");
currentMenuButtonId = id;
}
}
@NiftyEventSubscriber(id="console")
public void onConsoleEvent(final String id, @Nonnull final ConsoleExecuteCommandEvent executeCommandEvent) {
System.out.println(executeCommandEvent.getCommandLine());
}
@NiftyEventSubscriber(id="resolutions")
public void onResolution(final String id, @Nonnull final DropDownSelectionChangedEvent event) {
screen.findElementById("whiteOverlay").startEffect(EffectEventId.onCustom, new EndNotify() {
@Override
public void perform() {
resControl.setResolution(event.getSelection());
nifty.enableAutoScaling(1024, 768);
nifty.resolutionChanged();
}
}, "onResolutionStart");
}
@NiftyEventSubscriber(id="scale-resolution")
public void onSliderChanged(final String id, @Nonnull final SliderChangedEvent sliderChangedEvent) {
nifty.enableAutoScaling(1024, 768, sliderChangedEvent.getValue(), sliderChangedEvent.getValue());
}
/**
* Get all LWJGL DisplayModes into the DropDown
* @param screen
*/
private void fillResolutionDropDown(@Nonnull final Screen screen) {
DropDown dropDown = screen.findNiftyControl("resolutions", DropDown.class);
final Collection resolutions = resControl.getResolutions();
for (T mode : resolutions) {
dropDown.addItem(mode);
}
dropDown.selectItem(resControl.getCurrentResolution());
}
private class ShowCommand implements ConsoleCommand {
@Override
public void execute(@Nonnull final String[] args) {
if (args.length != 2) {
console.outputError("command argument error");
return;
}
// this really is a hack to get from the command argument, like: "ListBox" to the matching "menuButtonId"
String menuButtonId = "menuButton" + args[1];
if (!buttonToDialogMap.containsKey(menuButtonId)) {
console.outputError("'" + menuButtonId + "' is not a registered dialog.");
return;
}
// just a gimmick
if (menuButtonId.equals(currentMenuButtonId)) {
console.outputError("Hah! Already there! I'm smart... :>");
return;
}
// finally switch
changeDialogTo(menuButtonId);
}
}
private class NiftyCommand implements ConsoleCommand {
@Override
public void execute(@Nonnull final String[] args) {
if (args.length != 2) {
console.outputError("command argument error");
return;
}
String param = args[1];
if ("screen".equals(param)) {
String screenDebugOutput = nifty.getCurrentScreen().debugOutput();
console.output(screenDebugOutput);
System.out.println(screenDebugOutput);
} else {
console.outputError("unknown parameter [" + args[1] + "]");
}
}
}
private class HelpCommand implements ConsoleCommand {
@Override
public void execute(@Nonnull final String[] args) {
console.output("---------------------------", HELP_COLOR);
console.output("Supported commands", HELP_COLOR);
console.output("---------------------------", HELP_COLOR);
for (String command : consoleCommands.getRegisteredCommands()) {
console.output(command, HELP_COLOR);
}
}
}
private class ExitCommand implements ConsoleCommand {
@Override
public void execute(@Nonnull final String[] args) {
console.output("good bye");
nifty.closePopup(consolePopup.getId());
}
}
private class ClearCommand implements ConsoleCommand {
@Override
public void execute(@Nonnull final String[] args) {
console.clear();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy