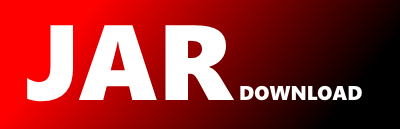
de.lessvoid.nifty.examples.defaultcontrols.treebox.TreeboxControlDialogController Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of nifty-examples Show documentation
Show all versions of nifty-examples Show documentation
Lots of Nifty example code! You can find the source for nearly all demos/tutorials in here.
package de.lessvoid.nifty.examples.defaultcontrols.treebox;
import de.lessvoid.nifty.Nifty;
import de.lessvoid.nifty.NiftyEventSubscriber;
import de.lessvoid.nifty.controls.*;
import de.lessvoid.nifty.elements.Element;
import de.lessvoid.nifty.input.NiftyInputEvent;
import de.lessvoid.nifty.screen.Screen;
import javax.annotation.Nonnull;
/**
* The TreeboxControlDialogController registers a new control with Nifty
* that represents the whole Dialog. This gives us later an appropriate
* ControlBuilder to actual construct the Dialog (as a control).
* @author ractoc
*/
public class TreeboxControlDialogController implements Controller {
private Nifty nifty;
@Override
public void bind(
@Nonnull final Nifty nifty,
@Nonnull final Screen screen,
@Nonnull final Element element,
@Nonnull final Parameters parameter) {
TreeBox treebox = screen.findNiftyControl("tree-box", TreeBox.class);
this.nifty = nifty;
treebox.setTree(setupTree());
}
@Override
public void init(@Nonnull final Parameters parameter) {
}
@Override
public void onStartScreen() {
}
@Override
public void onFocus(final boolean getFocus) {
}
@Override
public boolean inputEvent(@Nonnull final NiftyInputEvent inputEvent) {
return false;
}
@Override
public void onEndScreen() {
}
@Nonnull
private TreeItem setupTree() {
TreeItem treeRoot = new TreeItem();
TreeItem branch1 = new TreeItem("branch 1");
branch1.setExpanded(true);
TreeItem branch11 = new TreeItem("branch 1 1");
TreeItem branch12 = new TreeItem("branch 1 2");
branch1.addTreeItem(branch11);
branch1.addTreeItem(branch12);
TreeItem branch2 = new TreeItem("branch 2");
TreeItem branch21 = new TreeItem("branch 2 1");
TreeItem branch211 = new TreeItem("branch 2 1 1");
branch2.addTreeItem(branch21);
branch21.addTreeItem(branch211);
treeRoot.addTreeItem(branch1);
treeRoot.addTreeItem(branch2);
return treeRoot;
}
@NiftyEventSubscriber(id="tree-box")
public void treeItemSelected(final String id, @Nonnull final TreeItemSelectionChangedEvent event) {
final TextField text = nifty.getCurrentScreen().findNiftyControl("selectedItemText", TextField.class);
if (!event.getSelection().isEmpty()) {
text.setText(event.getSelection().get(0).getValue());
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy