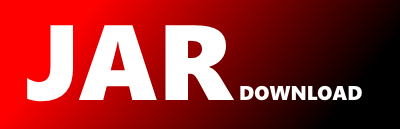
com.example.mylibrary1.LibClass Maven / Gradle / Ivy
/*
* LibClass.java
* mylibrary
*
* Copyright (C) 2020, Gleb Nikitenko.
*
* Permission is hereby granted, free of charge, to any person obtaining a copy
* of this software and associated documentation files (the "Software"), to deal
* in the Software without restriction, including without limitation the rights
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
* copies of the Software, and to permit persons to whom the Software is
* furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included in all
* copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
* SOFTWARE.
*/
package com.example.mylibrary1;
public final class LibClass {
public LibClass() {
System.out.println("LibClass.LibClass " + System.currentTimeMillis());
System.out.println("LibClass.LibClass " + System.currentTimeMillis());
/*final EffectContext ec = EffectContext.createWithCurrentGlContext();
final Effect effect = ec.getFactory().createEffect(EffectFactory.EFFECT_AUTOFIX);
effect.apply();
effect.release();
ec.release();*/
}
public static void m2(String a) {
for (int i = 0; i < 5; i++) {
System.out.println("LibClass.m2 " + a);
}
}
public static void countingSort(int[] values, int min, int max) {
final int range = max - min + 1;
final int[] count = new int[range];
final int[] output = new int[values.length];
// число вхождений
for (int i = 0; i < values.length; i++) count[values[i] - min]++;
// сумма вхождений
for (int i = 1; i < count.length; i++) count[i] += count[i - 1];
//в соответствии с суммами
for (int i = values.length - 1; i >= 0; i--) {
output[count[values[i] - min] - 1] = values[i];
count[values[i] - min]--;
}
// for (int i = 0; i < values.length; i++) values[i] = output[i];
}
public static void insertionSort(int[] array) {
for (int i = 1; i < array.length; i++) {
int current = array[i];
int j = i - 1;
while (j >= 0 && current < array[j]) {
array[j + 1] = array[j];
j--;
}
// в этой точке мы вышли, так что j так же -1
// или в первом элементе, где текущий >= a[j]
array[j + 1] = current;
}
}
public static void selectionSort(int[] array) {
for (int i = 0; i < array.length; i++) {
int min = array[i];
int minId = i;
for (int j = i + 1; j < array.length; j++) {
if (array[j] < min) {
min = array[j];
minId = j;
}
}
// замена
int temp = array[i];
array[i] = min;
array[minId] = temp;
}
}
private void bubbleSort(int[] arr)// o(n^2)
{
int n = arr.length;
for (int i = 0; i < n - 1; i++)
for (int j = 0; j < n - i - 1; j++)
if (arr[j] > arr[j + 1]) {
// swap arr[j+1] and arr[i]
int temp = arr[j];
arr[j] = arr[j + 1];
arr[j + 1] = temp;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy