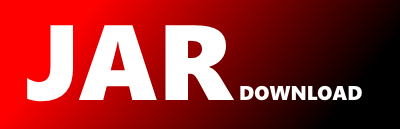
djdbc.Transaction Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of djdbc Show documentation
Show all versions of djdbc Show documentation
Declarative abstraction over JDBC
The newest version!
package djdbc;
import java.sql.SQLException;
/**
* A composable transaction.
* It is abstracted away from the notion of database connections or
* any other kind of resources.
* All you deal with is the structure of the transaction and that's it.
* It also abstracts away from the concurrent transaction conflicts,
* also known as serialization errors,
* by automatically retrying the transaction when they arise.
*
* @param The input parameters
* @param The result
*/
public interface Transaction {
/**
* The isolation level of this transaction.
*
* If this transaction encapsulates other transactions,
* it is recommended to use
* {@link TransactionIsolation#max(TransactionIsolation, TransactionIsolation...)}
* to merge the isolation levels of all nested transactions
* by picking the highest.
*/
TransactionIsolation getIsolation();
/**
* The body of the transaction.
* The implementation of this method must not contain any
* effects except for those that the {@code context} parameter provides.
* The reason is that the code in this method may get executed multiple times
* in case concurrent transaction conflicts arise
* (e.g., SQL State "40001" of PostgreSQL).
*/
result run(TransactionContext context, params params) throws SQLException;
/**
* A helper class for construction of transactions from
* an iterable of void statements.
*/
final class VoidStatements implements Transaction {
private final TransactionIsolation isolation;
private final Iterable> statements;
public VoidStatements(TransactionIsolation isolation, Iterable> statements) {
this.isolation = isolation;
this.statements = statements;
}
@Override
public TransactionIsolation getIsolation() {
return isolation;
}
@Override
public Void run(TransactionContext context, Void aVoid) throws SQLException {
for (Statement statement : statements) {
context.execute(statement);
}
return null;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy