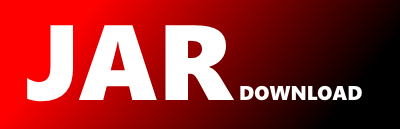
com.github.nill14.parsers.dependency.IDependencyGraph Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of graph Show documentation
Show all versions of graph Show documentation
Implementation of directed acyclic graph along with some graph algorithms and dependency management.
The newest version!
package com.github.nill14.parsers.dependency;
import java.util.List;
import java.util.Map;
import java.util.Set;
import java.util.concurrent.ExecutionException;
import java.util.concurrent.ExecutorService;
import com.github.nill14.parsers.graph.DirectedGraph;
import com.github.nill14.parsers.graph.GraphEdge;
public interface IDependencyGraph {
/**
* In the graph a dependency relation is modeled by precursors direction
* That is, when sorted topologically, the most left element doesn't depend on anything.
* The foremost right element depends on it's precursors
* @return directed acyclic graph
*/
DirectedGraph> getGraph();
/**
*
* @return a set of modules
*/
Set getModules();
/**
*
* @param module The module having dependencies
* @return A set of direct dependencies of M, not considering transitive.
*/
Set getDirectDependencies(M module);
/**
*
* @param module The module having dependencies
* @return A set of all dependencies of M, including transitive.
*/
Set getAllDependencies(M module);
/**
*
* @return a topologically sorted list of modules
*/
List getTopologicalOrder();
/**
* Returns module ratings based on longest path algorithm and module priority.
* The modules sorted by the module rating descendingly are guaranteed
* to be in topological order.
*
* @return module rankings
*/
Map getModuleRankings();
/**
*
* @param executor an executor to be used for executing the closure
* @param moduleConsumer a processing closure
* @throws ExecutionException when the closure throws an exception
*/
void walkGraph(ExecutorService executor, IConsumer moduleConsumer) throws ExecutionException;
/**
* Synchronous version of {@link #walkGraph(ExecutorService, IConsumer)}
* The order is guaranteed to be the same as {@link #getTopologicalOrder()}
*
* @param moduleConsumer a processing closure
* @throws ExecutionException when the closure throws an exception
*/
void iterateTopoOrder(IConsumer moduleConsumer) throws ExecutionException;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy