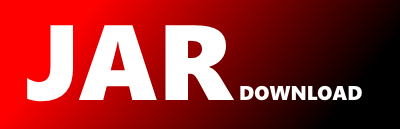
com.github.nill14.parsers.dependency.impl.DependencyDescriptor Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of graph Show documentation
Show all versions of graph Show documentation
Implementation of directed acyclic graph along with some graph algorithms and dependency management.
The newest version!
package com.github.nill14.parsers.dependency.impl;
import java.util.Set;
import com.github.nill14.parsers.dependency.IDependencyDescriptor;
import com.github.nill14.parsers.dependency.IDependencyDescriptorBuilder;
import com.google.common.collect.ImmutableSet;
public final class DependencyDescriptor implements IDependencyDescriptor {
private final ImmutableSet dependencies;
private final ImmutableSet optDependencies;
private final ImmutableSet optProviders;
private final K self;
private final int priority;
private DependencyDescriptor(Builder builder) {
dependencies = builder.dependencies.build();
optDependencies = builder.optDependencies.build();
optProviders = builder.providers.build();
self = builder.self;
priority = builder.priority;
if (priority < 0 || priority > 100000) {
throw new IllegalArgumentException("Priority not in range 0..100000: "+ priority);
}
}
@Override
public Set getRequiredDependencies() {
return dependencies;
}
@Override
public Set getOptionalDependencies() {
return optDependencies;
}
@Override
public Set getOptionalProviders() {
return optProviders;
}
@Override
public int getExecutionPriority() {
return priority;
}
public static IDependencyDescriptorBuilder builder(K self) {
return new Builder<>(self);
}
@Override
public String toString() {
return self.toString();
}
public static class Builder implements IDependencyDescriptorBuilder {
private final ImmutableSet.Builder dependencies = ImmutableSet.builder();
private final ImmutableSet.Builder optDependencies = ImmutableSet.builder();
private final ImmutableSet.Builder providers = ImmutableSet.builder();
private final K self;
private int priority = 0;
public Builder(K name) {
this.self = name;
providers.add(name);
}
@Override
public IDependencyDescriptorBuilder uses(K dependency) {
dependencies.add(dependency);
return this;
}
@Override
public IDependencyDescriptorBuilder usesOptionally(K dependency) {
optDependencies.add(dependency);
return this;
}
@Override
public IDependencyDescriptorBuilder provides(K provider) {
providers.add(provider);
return this;
}
@Override
public IDependencyDescriptorBuilder executionPriority(int priority) {
this.priority = priority;
return this;
}
@Override
public IDependencyDescriptor build() {
return new DependencyDescriptor<>(this);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy