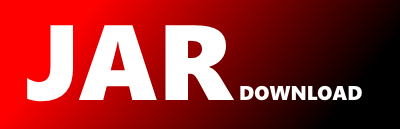
com.github.nill14.parsers.dependency.impl.ModuleRankingsPrinter Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of graph Show documentation
Show all versions of graph Show documentation
Implementation of directed acyclic graph along with some graph algorithms and dependency management.
The newest version!
package com.github.nill14.parsers.dependency.impl;
import java.io.PrintStream;
import java.util.Collection;
import java.util.List;
import java.util.Map;
import org.slf4j.Logger;
import com.github.nill14.parsers.dependency.IDependencyGraph;
import com.google.common.collect.Lists;
public class ModuleRankingsPrinter {
private final IDependencyGraph dependencyGraph;
private final Map moduleRankings;
private final List topologicalOrder;
public ModuleRankingsPrinter(IDependencyGraph dependencyGraph) {
this.dependencyGraph = dependencyGraph;
moduleRankings = dependencyGraph.getModuleRankings();
topologicalOrder = dependencyGraph.getTopologicalOrder();
}
private String printLine(M vertex, boolean executionBit, int ranking) {
if (executionBit) {
return String.format("* %s (%d)", vertex, ranking);
} else {
return String.format(" %s (%d)", vertex, ranking);
}
}
public Collection getLines() {
Collection result = Lists.newArrayList();
for (M node : topologicalOrder) {
boolean executionBit = dependencyGraph.getDirectDependencies(node).isEmpty();
int ranking = moduleRankings.get(node);
String line = printLine(node, executionBit, ranking);
result.add(line);
}
return result;
}
/**
* Outputs module rankings to System.out
*/
public void toConsole() {
toPrintStream(System.out);
}
/**
* Outputs module rankings to a PrintStream
*/
public void toPrintStream(PrintStream p) {
p.println("Module Rankings");
for (String line : getLines()) {
p.println(line);
}
}
/**
* Outputs module rankings to {@link Logger#info(String)}
*/
public void toInfoLog(Logger log) {
if (log.isInfoEnabled()) {
log.info("Module Rankings");
for (String line : getLines()) {
log.info(line);
}
}
}
/**
* Outputs module rankings to {@link Logger#debug(String)}
*/
public void toDebugLog(Logger log) {
if (log.isDebugEnabled()) {
log.debug("Module Rankings");
for (String line : getLines()) {
log.debug(line);
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy