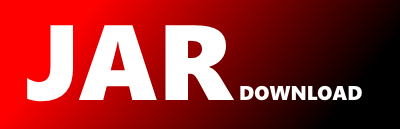
com.github.nill14.utils.init.impl.AbstractPropertyResolver Maven / Gradle / Ivy
package com.github.nill14.utils.init.impl;
import java.lang.annotation.Annotation;
import java.util.Collection;
import javax.inject.Provider;
import com.github.nill14.utils.init.api.IBeanDescriptor;
import com.github.nill14.utils.init.api.IParameterType;
import com.github.nill14.utils.init.api.IPojoInitializer;
import com.github.nill14.utils.init.api.IPropertyResolver;
import com.github.nill14.utils.init.inject.PojoInjectionDescriptor;
import com.google.common.base.Preconditions;
import com.google.common.collect.ImmutableList;
import com.google.common.collect.ImmutableSet;
import com.google.common.collect.ImmutableSortedSet;
@SuppressWarnings("serial")
public abstract class AbstractPropertyResolver implements IPropertyResolver {
@SuppressWarnings("unchecked")
@Override
public Object resolve(Object pojo, IParameterType type) {
if (type.isParametrized()) {
Class> rawType = type.getRawType();
Class> paramClass = type.getFirstParamClass();
if (java.util.Optional.class.isAssignableFrom(rawType)) {
return java.util.Optional.ofNullable(doResolve(pojo, type, paramClass));
}
if (com.google.common.base.Optional.class.isAssignableFrom(rawType)) {
return com.google.common.base.Optional.fromNullable(doResolve(pojo, type, paramClass));
}
if (Iterable.class.isAssignableFrom(rawType)) {
return doResolveCollection(pojo, rawType, paramClass);
}
}
Object result = doResolve(pojo, type, type.getRawType());
if (result != null) {
return result;
}
//Wire mode is implicit now
// if (type.getAnnotation(Wire.class).isPresent()) {
// try {
IBeanDescriptor> typeDescriptor = new PojoInjectionDescriptor<>(type);
if (typeDescriptor.canBeInstantiated()) {
@SuppressWarnings({ "rawtypes" })
Provider factory = new PojoFactory(typeDescriptor, this);
IPojoInitializer
© 2015 - 2025 Weber Informatics LLC | Privacy Policy