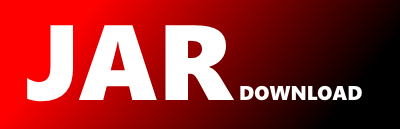
com.github.nill14.utils.init.inject.ReflectionUtils Maven / Gradle / Ivy
package com.github.nill14.utils.init.inject;
import java.lang.annotation.Annotation;
import java.lang.reflect.Method;
import java.util.List;
import java.util.Optional;
import java.util.stream.Stream;
import javax.inject.Provider;
import com.github.nill14.utils.init.api.BindingKey;
import com.github.nill14.utils.init.api.IScope;
import com.github.nill14.utils.init.binding.Binder;
import com.github.nill14.utils.init.binding.impl.BindingImpl;
import com.github.nill14.utils.init.binding.target.ProvidesMethodBindingTarget;
import com.github.nill14.utils.init.meta.AnnotationScanner;
import com.github.nill14.utils.init.meta.Provides;
import com.github.nill14.utils.init.scope.PrototypeScope;
import com.google.common.collect.Lists;
import com.google.common.reflect.TypeToken;
import com.google.common.reflect.TypeToken.TypeSet;
public enum ReflectionUtils {
;
@SuppressWarnings({ "unchecked" })
public static TypeToken getProviderReturnTypeToken(Class extends Provider extends T>> providerClass) {
try {
return (TypeToken) TypeToken.of(providerClass.getMethod("get").getGenericReturnType());
} catch (ReflectiveOperationException e) {
throw new RuntimeException(e);
}
}
@SuppressWarnings({ "unchecked" })
public static TypeToken getProviderReturnTypeToken(Provider extends T> provider) {
return getProviderReturnTypeToken((Class extends Provider extends T>>) provider.getClass());
}
@SuppressWarnings({ "unchecked" })
public static TypeToken getProviderReturnTypeToken(TypeToken extends Provider extends T>> providerType) {
return getProviderReturnTypeToken((Class extends Provider extends T>>)providerType.getRawType());
}
public static boolean isClassPresent(String name) {
try {
Class.forName(name);
return true;
} catch(ClassNotFoundException e) {
return false;
}
}
@SuppressWarnings({ "rawtypes", "unchecked" })
public static List> scanProvidesBindings(Binder binder, Object module) {
List> result = Lists.newArrayList();
Class> moduleClass = module.getClass();
for (Method m : moduleClass.getDeclaredMethods()) {
if (m.isAnnotationPresent(Provides.class)) {
TypeToken typeToken = TypeToken.of(m.getGenericReturnType());
ProvidesMethodBindingTarget
© 2015 - 2025 Weber Informatics LLC | Privacy Policy