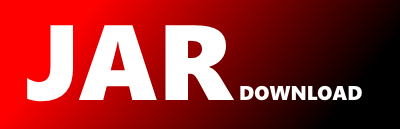
com.github.nill14.utils.init.meta.Annotations Maven / Gradle / Ivy
The newest version!
/**
* Copyright (C) 2006 Google Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.github.nill14.utils.init.meta;
import java.lang.annotation.Annotation;
import java.lang.reflect.Method;
import javax.inject.Named;
import com.github.nill14.utils.init.meta.impl.AnnotationInvocationHandler;
import com.google.common.base.Preconditions;
import com.google.common.cache.CacheBuilder;
import com.google.common.cache.CacheLoader;
import com.google.common.cache.LoadingCache;
import com.google.common.collect.ImmutableMap;
import com.google.common.reflect.Reflection;
public class Annotations {
private Annotations() {}
public static Named named(String name) {
return new NamedImpl(name);
}
/**
* Generates an annotation of the expected annotation type.
* All annotation type properties are expected to have a default value.
*
* @param annotationType The annotation class
* @return annotation object for the annotation class
*/
@SuppressWarnings("unchecked")
public static T annotation(Class annotationType) {
Preconditions.checkState(isAllDefaultMethods(annotationType),
"%s is not all default methods", annotationType);
return (T) cache0.getUnchecked(annotationType);
}
/**
* Generates an annotation of the expected annotation type.
*
* @param annotationType The annotation class
* @param value The result of the annotation's value() method
* @return annotation object for the annotation class
*/
public static T withValue(Class annotationType, String value) {
return withValue0(annotationType, value);
}
/**
* Generates an annotation of the expected annotation type.
*
* @param annotationType The annotation class
* @param value The result of the annotation's value() method
* @return annotation object for the annotation class
*
*/
public static T withValue(Class annotationType, int value) {
return withValue0(annotationType, value);
}
/**
* Generates an annotation of the expected annotation type.
*
* @param annotationType The annotation class
* @param value The result of the annotation's value() method
* @return annotation object for the annotation class
*/
public static T withValue(Class annotationType, Class> value) {
return withValue0(annotationType, value);
}
public static > T withValue(Class annotationType, E value) {
return withValue0(annotationType, value);
}
/**
* Generates an annotation of the expected annotation type.
*
* @param annotationType The annotation class
* @param value The result of the annotation's value() method
* @return annotation object for the annotation class
*/
public static T withValue(Class annotationType, float value) {
return withValue0(annotationType, value);
}
/**
* Generates an annotation of the expected annotation type.
*
* @param annotationType The annotation class
* @param value The result of the annotation's value() method
* @return annotation object for the annotation class
*/
public static T withValue(Class annotationType, double value) {
return withValue0(annotationType, value);
}
/**
* Generates an annotation of the expected annotation type.
*
* @param annotationType The annotation class
* @param value The result of the annotation's value() method
* @return annotation object for the annotation class
*/
public static T withValue(Class annotationType, long value) {
return withValue0(annotationType, value);
}
/**
* Generates an Annotation with value for the annotation class.
* All other values are defaults.
*/
@SuppressWarnings("unchecked")
private static T withValue0(Class annotationType, Object value) {
Preconditions.checkState(isAllDefaultMethodsExceptValue(annotationType),
"%s is not all default methods", annotationType);
return (T) cache1.getUnchecked(new KeyWithValues<>(annotationType, definedValue(value)));
}
private static ImmutableMap definedValue(Object value) {
return ImmutableMap.of("value", value);
}
private static boolean isAllDefaultMethodsExceptValue(Class extends Annotation> annotationType) {
for (Method m : annotationType.getDeclaredMethods()) {
if (m.getDefaultValue() == null && !"value".equals(m.getName())) {
return false;
}
}
return true;
}
private static boolean isAllDefaultMethods(Class extends Annotation> annotationType) {
for (Method m : annotationType.getDeclaredMethods()) {
if (m.getDefaultValue() == null) {
return false;
}
}
return true;
}
private static final LoadingCache, Annotation> cache0 = CacheBuilder.newBuilder()
.weakKeys()
.build(new CacheLoader, Annotation>() {
@Override
public Annotation load(Class extends Annotation> annotationType) {
return Reflection.newProxy(annotationType, new AnnotationInvocationHandler<>(annotationType));
}
});
private static final LoadingCache, Annotation> cache1 = CacheBuilder.newBuilder()
.weakKeys()
.build(new CacheLoader, Annotation>() {
@Override
public Annotation load(KeyWithValues extends Annotation> key) {
return Reflection.newProxy(key.annotationType,
new AnnotationInvocationHandler<>(key.annotationType, key.values));
}
});
private static final class KeyWithValues {
final Class annotationType;
final ImmutableMap values;
public KeyWithValues(Class annotationType, ImmutableMap values) {
this.annotationType = annotationType;
this.values = values;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy