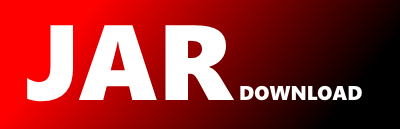
com.github.nmorel.gwtjackson.rest.api.RestRequestBuilder Maven / Gradle / Ivy
/*
* Copyright 2015 Nicolas Morel
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.github.nmorel.gwtjackson.rest.api;
import java.util.ArrayList;
import java.util.Collection;
import java.util.LinkedHashMap;
import java.util.List;
import java.util.Map;
import java.util.Map.Entry;
import com.github.nmorel.gwtjackson.client.ObjectReader;
import com.github.nmorel.gwtjackson.client.ObjectWriter;
import com.google.gwt.http.client.Request;
import com.google.gwt.http.client.RequestBuilder;
import com.google.gwt.http.client.RequestBuilder.Method;
import com.google.gwt.http.client.RequestException;
import com.google.gwt.http.client.URL;
/**
* @author Nicolas Morel
*/
public class RestRequestBuilder {
private static String defaultApplicationPath = "";
public static void setDefaultApplicationPath( String defaultApplicationPath ) {
if ( null == defaultApplicationPath ) {
throw new IllegalArgumentException( "Application path cannot be null" );
}
RestRequestBuilder.defaultApplicationPath = defaultApplicationPath;
}
/**
* HTTP method to use when opening a JavaScript XmlHttpRequest object.
*/
private Method method;
/**
* Application path to concatenate before the url
*/
private String applicationPath = defaultApplicationPath;
/**
* URL to use when opening a JavaScript XmlHttpRequest object.
*/
private String url;
/**
* User to use when opening a JavaScript XmlHttpRequest object.
*/
private String user;
/**
* Password to use when opening a JavaScript XmlHttpRequest object.
*/
private String password;
/**
* Whether to include credentials for a Cross Origin Request.
*/
private Boolean includeCredentials;
/**
* Timeout in milliseconds before the request timeouts and fails.
*/
private Integer timeoutMillis;
/**
* Map of header name to value that will be added to the JavaScript
* XmlHttpRequest object before sending a request.
*/
private Map headers;
private Map> queryParams;
private Map pathParams;
private B body;
private ObjectWriter bodyConverter;
private ObjectReader responseConverter;
private RestCallback callback;
public RestRequestBuilder() {
}
public RestRequestBuilder method( Method method ) {
this.method = method;
return this;
}
public RestRequestBuilder applicationPath( String applicationPath ) {
this.applicationPath = applicationPath;
return this;
}
public RestRequestBuilder url( String url ) {
this.url = url;
return this;
}
public RestRequestBuilder user( String user ) {
this.user = user;
return this;
}
public RestRequestBuilder password( String password ) {
this.password = password;
return this;
}
public RestRequestBuilder includeCredentials( boolean includeCredentials ) {
this.includeCredentials = includeCredentials;
return this;
}
public RestRequestBuilder timeout( int timeoutMillis ) {
this.timeoutMillis = timeoutMillis;
return this;
}
public RestRequestBuilder addHeader( String name, String value ) {
if ( null == headers ) {
headers = new LinkedHashMap();
}
headers.put( name, value );
return this;
}
public Map getHeaders() {
return headers;
}
/**
* Special case where you want to add a query param without value like ?key1&key2=value
*
* @param name Name of the parameter
*
* @return this builder
*/
public RestRequestBuilder addQueryParam( String name ) {
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy