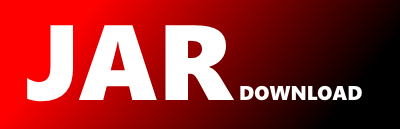
com.github.nmorel.gwtjackson.client.JsonSerializationContext Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gwt-jackson Show documentation
Show all versions of gwt-jackson Show documentation
gwt-jackson is a GWT JSON serializer/deserializer mechanism based on Jackson annotations
package com.github.nmorel.gwtjackson.client;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.IdentityHashMap;
import java.util.List;
import java.util.Map;
import java.util.logging.Level;
import java.util.logging.Logger;
import com.fasterxml.jackson.annotation.ObjectIdGenerator;
import com.github.nmorel.gwtjackson.client.exception.JsonSerializationException;
import com.github.nmorel.gwtjackson.client.ser.bean.AbstractBeanJsonSerializer;
import com.github.nmorel.gwtjackson.client.ser.bean.ObjectIdSerializer;
import com.github.nmorel.gwtjackson.client.stream.JsonWriter;
import com.google.gwt.logging.client.LogConfiguration;
/**
* Context for the serialization process.
*
* @author Nicolas Morel
*/
public class JsonSerializationContext extends JsonMappingContext {
public static class Builder {
private boolean useEqualityForObjectId = false;
private boolean serializeNulls = true;
private boolean writeDatesAsTimestamps = true;
private boolean writeDateKeysAsTimestamps = false;
private boolean indent = false;
/**
* Determines whether Object Identity is compared using
* true JVM-level identity of Object (false); or, equals()
method.
* Latter is sometimes useful when dealing with Database-bound objects with
* ORM libraries (like Hibernate).
*
* Option is disabled by default; meaning that strict identity is used, not
* equals()
*/
public Builder useEqualityForObjectId( boolean useEqualityForObjectId ) {
this.useEqualityForObjectId = useEqualityForObjectId;
return this;
}
/**
* Sets whether object members are serialized when their value is null.
* This has no impact on array elements. The default is true.
*/
public Builder serializeNulls( boolean serializeNulls ) {
this.serializeNulls = serializeNulls;
return this;
}
/**
* Determines whether {@link java.util.Date} and {@link java.sql.Timestamp} values are to be serialized as numeric timestamps
* (true; the default), or as textual representation.
* If textual representation is used, the actual format is
* {@link com.google.gwt.i18n.client.DateTimeFormat.PredefinedFormat#ISO_8601}
* Option is enabled by default.
*/
public Builder writeDatesAsTimestamps( boolean writeDatesAsTimestamps ) {
this.writeDatesAsTimestamps = writeDatesAsTimestamps;
return this;
}
/**
* Feature that determines whether {@link java.util.Date}s and {@link java.sql.Timestamp}s used as {@link java.util.Map} keys are
* serialized as timestamps or as textual values.
* If textual representation is used, the actual format is
* {@link com.google.gwt.i18n.client.DateTimeFormat.PredefinedFormat#ISO_8601}
* Option is disabled by default.
*/
public Builder writeDateKeysAsTimestamps( boolean writeDateKeysAsTimestamps ) {
this.writeDateKeysAsTimestamps = writeDateKeysAsTimestamps;
return this;
}
/**
* Feature that allows enabling (or disabling) indentation
* for the underlying writer.
* Feature is disabled by default.
*/
public Builder indent( boolean indent ) {
this.indent = indent;
return this;
}
public JsonSerializationContext build() {
return new JsonSerializationContext( useEqualityForObjectId, serializeNulls, writeDatesAsTimestamps,
writeDateKeysAsTimestamps, indent );
}
}
private static final Logger logger = Logger.getLogger( "JsonSerialization" );
private Map
© 2015 - 2025 Weber Informatics LLC | Privacy Policy