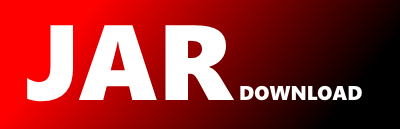
com.github.nmorel.gwtjackson.rebind.property.PropertyInfoBuilder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gwt-jackson Show documentation
Show all versions of gwt-jackson Show documentation
gwt-jackson is a GWT JSON serializer/deserializer mechanism based on Jackson annotations
/*
* Copyright 2014 Nicolas Morel
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.github.nmorel.gwtjackson.rebind.property;
import com.fasterxml.jackson.annotation.JsonFormat;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.github.nmorel.gwtjackson.rebind.bean.BeanIdentityInfo;
import com.github.nmorel.gwtjackson.rebind.bean.BeanTypeInfo;
import com.google.gwt.core.ext.typeinfo.JType;
import com.google.gwt.thirdparty.guava.common.base.Optional;
/**
* @author Nicolas Morel.
*/
final class PropertyInfoBuilder {
private final String propertyName;
private final JType type;
private boolean ignored = false;
private boolean required = false;
private boolean rawValue = false;
private boolean value = false;
private boolean anyGetter = false;
private boolean anySetter = false;
private boolean unwrapped = false;
private Optional managedReference = Optional.absent();
private Optional backReference = Optional.absent();
private Optional extends FieldAccessor> getterAccessor = Optional.absent();
private Optional extends FieldAccessor> setterAccessor = Optional.absent();
private Optional identityInfo = Optional.absent();
private Optional typeInfo = Optional.absent();
private Optional format = Optional.absent();
private Optional include = Optional.absent();
private Optional ignoreUnknown = Optional.absent();
private Optional ignoredProperties = Optional.absent();
PropertyInfoBuilder( String propertyName, JType type ) {
this.propertyName = propertyName;
this.type = type;
}
String getPropertyName() {
return propertyName;
}
JType getType() {
return type;
}
boolean isIgnored() {
return ignored;
}
void setIgnored( boolean ignored ) {
this.ignored = ignored;
}
boolean isRequired() {
return required;
}
void setRequired( boolean required ) {
this.required = required;
}
boolean isRawValue() {
return rawValue;
}
void setRawValue( boolean rawValue ) {
this.rawValue = rawValue;
}
boolean isValue() {
return value;
}
void setValue( boolean value ) {
this.value = value;
}
boolean isAnyGetter() {
return anyGetter;
}
void setAnyGetter( boolean anyGetter ) {
this.anyGetter = anyGetter;
}
boolean isAnySetter() {
return anySetter;
}
void setAnySetter( boolean anySetter ) {
this.anySetter = anySetter;
}
boolean isUnwrapped() {
return unwrapped;
}
void setUnwrapped( boolean unwrapped ) {
this.unwrapped = unwrapped;
}
Optional getManagedReference() {
return managedReference;
}
void setManagedReference( Optional managedReference ) {
this.managedReference = managedReference;
}
Optional getBackReference() {
return backReference;
}
void setBackReference( Optional backReference ) {
this.backReference = backReference;
}
Optional extends FieldAccessor> getGetterAccessor() {
return getterAccessor;
}
void setGetterAccessor( Optional extends FieldAccessor> getterAccessor ) {
this.getterAccessor = getterAccessor;
}
Optional extends FieldAccessor> getSetterAccessor() {
return setterAccessor;
}
void setSetterAccessor( Optional extends FieldAccessor> setterAccessor ) {
this.setterAccessor = setterAccessor;
}
Optional getIdentityInfo() {
return identityInfo;
}
void setIdentityInfo( Optional identityInfo ) {
this.identityInfo = identityInfo;
}
Optional getTypeInfo() {
return typeInfo;
}
void setTypeInfo( Optional typeInfo ) {
this.typeInfo = typeInfo;
}
Optional getFormat() {
return format;
}
void setFormat( Optional format ) {
this.format = format;
}
Optional getInclude() {
return include;
}
void setInclude( Optional include ) {
this.include = include;
}
Optional getIgnoreUnknown() {
return ignoreUnknown;
}
void setIgnoreUnknown( Optional ignoreUnknown ) {
this.ignoreUnknown = ignoreUnknown;
}
Optional getIgnoredProperties() {
return ignoredProperties;
}
void setIgnoredProperties( Optional ignoredProperties ) {
this.ignoredProperties = ignoredProperties;
}
PropertyInfo build() {
return new PropertyInfo( propertyName, type, ignored, required, rawValue, value, anyGetter, anySetter, unwrapped,
managedReference, backReference, getterAccessor, setterAccessor, identityInfo, typeInfo, format, include, ignoreUnknown,
ignoredProperties );
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy