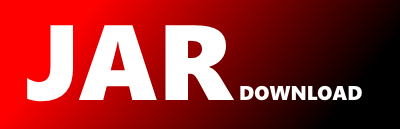
com.github.nmorel.gwtjackson.rebind.property.PropertyInfo Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gwt-jackson Show documentation
Show all versions of gwt-jackson Show documentation
gwt-jackson is a GWT JSON serializer/deserializer mechanism based on Jackson annotations
/*
* Copyright 2013 Nicolas Morel
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.github.nmorel.gwtjackson.rebind.property;
import com.fasterxml.jackson.annotation.JsonFormat;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.github.nmorel.gwtjackson.rebind.bean.BeanIdentityInfo;
import com.github.nmorel.gwtjackson.rebind.bean.BeanTypeInfo;
import com.google.gwt.core.ext.typeinfo.JType;
import com.google.gwt.thirdparty.guava.common.base.Optional;
/**
* PropertyInfo class.
*
* @author Nicolas Morel
* @version $Id: $
*/
public final class PropertyInfo {
private final String propertyName;
private final JType type;
private final boolean ignored;
private final boolean required;
private final boolean rawValue;
private final boolean value;
private final boolean anyGetter;
private final boolean anySetter;
private final boolean unwrapped;
private final Optional managedReference;
private final Optional backReference;
private final Optional extends FieldAccessor> getterAccessor;
private final Optional extends FieldAccessor> setterAccessor;
private final Optional identityInfo;
private final Optional typeInfo;
private final Optional format;
private final Optional include;
private final Optional ignoreUnknown;
private final Optional ignoredProperties;
PropertyInfo( String propertyName, JType type, boolean ignored, boolean required, boolean rawValue, boolean value, boolean
anyGetter, boolean anySetter, boolean unwrapped, Optional managedReference, Optional backReference,
Optional extends
FieldAccessor> getterAccessor, Optional extends FieldAccessor> setterAccessor, Optional
identityInfo,
Optional typeInfo, Optional format, Optional include, Optional
ignoreUnknown, Optional ignoredProperties ) {
this.propertyName = propertyName;
this.type = type;
this.ignored = ignored;
this.required = required;
this.rawValue = rawValue;
this.value = value;
this.anyGetter = anyGetter;
this.anySetter = anySetter;
this.unwrapped = unwrapped;
this.managedReference = managedReference;
this.backReference = backReference;
this.getterAccessor = getterAccessor;
this.setterAccessor = setterAccessor;
this.identityInfo = identityInfo;
this.typeInfo = typeInfo;
this.format = format;
this.include = include;
this.ignoreUnknown = ignoreUnknown;
this.ignoredProperties = ignoredProperties;
}
/**
* Getter for the field propertyName
.
*
* @return a {@link java.lang.String} object.
*/
public String getPropertyName() {
return propertyName;
}
/**
* Getter for the field type
.
*
* @return a {@link com.google.gwt.core.ext.typeinfo.JType} object.
*/
public JType getType() {
return type;
}
/**
* isIgnored
*
* @return a boolean.
*/
public boolean isIgnored() {
return ignored;
}
/**
* isRequired
*
* @return a boolean.
*/
public boolean isRequired() {
return required;
}
/**
* isRawValue
*
* @return a boolean.
*/
public boolean isRawValue() {
return rawValue;
}
/**
* isValue
*
* @return a boolean.
*/
public boolean isValue() {
return value;
}
/**
* isAnyGetter
*
* @return a boolean.
*/
public boolean isAnyGetter() {
return anyGetter;
}
/**
* isAnySetter
*
* @return a boolean.
*/
public boolean isAnySetter() {
return anySetter;
}
/**
* isUnwrapped
*
* @return a boolean.
*/
public boolean isUnwrapped() {
return unwrapped;
}
/**
* Getter for the field managedReference
.
*
* @return a {@link com.google.gwt.thirdparty.guava.common.base.Optional} object.
*/
public Optional getManagedReference() {
return managedReference;
}
/**
* Getter for the field backReference
.
*
* @return a {@link com.google.gwt.thirdparty.guava.common.base.Optional} object.
*/
public Optional getBackReference() {
return backReference;
}
/**
* Getter for the field getterAccessor
.
*
* @return a {@link com.google.gwt.thirdparty.guava.common.base.Optional} object.
*/
public Optional extends FieldAccessor> getGetterAccessor() {
return getterAccessor;
}
/**
* Getter for the field setterAccessor
.
*
* @return a {@link com.google.gwt.thirdparty.guava.common.base.Optional} object.
*/
public Optional extends FieldAccessor> getSetterAccessor() {
return setterAccessor;
}
/**
* Getter for the field identityInfo
.
*
* @return a {@link com.google.gwt.thirdparty.guava.common.base.Optional} object.
*/
public Optional getIdentityInfo() {
return identityInfo;
}
/**
* Getter for the field typeInfo
.
*
* @return a {@link com.google.gwt.thirdparty.guava.common.base.Optional} object.
*/
public Optional getTypeInfo() {
return typeInfo;
}
/**
* Getter for the field format
.
*
* @return a {@link com.google.gwt.thirdparty.guava.common.base.Optional} object.
*/
public Optional getFormat() {
return format;
}
/**
* Getter for the field include
.
*
* @return a {@link com.google.gwt.thirdparty.guava.common.base.Optional} object.
*/
public Optional getInclude() {
return include;
}
/**
* Getter for the field ignoreUnknown
.
*
* @return a {@link com.google.gwt.thirdparty.guava.common.base.Optional} object.
*/
public Optional getIgnoreUnknown() {
return ignoreUnknown;
}
/**
* Getter for the field ignoredProperties
.
*
* @return a {@link com.google.gwt.thirdparty.guava.common.base.Optional} object.
*/
public Optional getIgnoredProperties() {
return ignoredProperties;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy