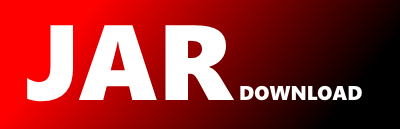
com.github.nomou.mybatis.builder.spi.Parameter Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of mybatis-smart Show documentation
Show all versions of mybatis-smart Show documentation
Mybatis-smart - a light mybatis mapper method implementation proxy
package com.github.nomou.mybatis.builder.spi;
import com.github.nomou.mybatis.util.Collections2;
import org.apache.ibatis.annotations.Param;
import org.apache.ibatis.reflection.Jdk;
import org.apache.ibatis.reflection.ParamNameUtil;
import java.lang.annotation.Annotation;
import java.lang.reflect.Method;
import java.lang.reflect.ParameterizedType;
import java.lang.reflect.Type;
import java.util.Collections;
import java.util.Map;
/**
* 方法参数.
*/
@SuppressWarnings("unused")
public class Parameter {
private final int parameterIndex;
private final String parameterName;
private final Type parameterType;
private final Class> parameterClass;
private final Method declaringMethod;
private final Map, Annotation> declaredAnnotations;
/**
* Package constructor.
*
* @param declaringMethod 定义方法
* @param parameterIndex 参数索引
* @param parameterType 参数类型
* @param useActualParameterName 是否使用实际参数名称(JDK8+适用)
* @see Parameters
*/
Parameter(final Method declaringMethod, final int parameterIndex,
final Type parameterType, final boolean useActualParameterName) {
Class> theClass;
if (null != parameterType) {
if (parameterType instanceof Class>) {
theClass = (Class>) parameterType;
} else if (parameterType instanceof ParameterizedType) {
theClass = (Class>) ((ParameterizedType) parameterType).getRawType();
} else {
throw new IllegalArgumentException("parameter type must be Class or ParameterizedType");
}
} else {
theClass = declaringMethod.getParameterTypes()[parameterIndex];
}
final Annotation[] annotations = declaringMethod.getParameterAnnotations()[parameterIndex];
final Map, Annotation> parameterAnnotations = Collections2.newHashMap((int) (annotations.length / .75f) + 1);
for (final Annotation anno : annotations) {
parameterAnnotations.put(anno.annotationType(), anno);
}
String parameterName = null;
final Param param = (Param) parameterAnnotations.get(Param.class);
if (null != param) {
parameterName = param.value();
}
if (null == parameterName && useActualParameterName && Jdk.parameterExists) {
parameterName = ParamNameUtil.getParamNames(declaringMethod).get(parameterIndex);
}
this.parameterIndex = parameterIndex;
this.parameterName = parameterName;
this.parameterType = parameterType;
this.parameterClass = theClass;
this.declaringMethod = declaringMethod;
this.declaredAnnotations = Collections.unmodifiableMap(parameterAnnotations);
}
/**
* 获取参数索引.
*
* @return 参数索引
*/
public int getIndex() {
return parameterIndex;
}
/**
* 获取参数名称.
*
* 注意这里的名称是有{@link Param}标注或者JDK8+且创建时指定了'useActualParameterName=true'时通过JDK8 API获取的名称.
*
* @return 如果可以获取返回参数名称, 否则返回null
*/
public String getName() {
return parameterName;
}
/**
* 获取参数类型.
*
* @return 参数类型
*/
public Class> getType() {
return parameterClass;
}
/**
* 获取参数的泛型类型.
*
* @return 参数的泛型类型
*/
public Type getGenericType() {
return null != parameterType ? parameterType : declaringMethod.getGenericParameterTypes()[parameterIndex];
}
/**
* 获取参数注解.
*
* @param annotationClass 注解Class
* @param 注解类型
* @return 如果存在对应类型注解返回, 否则返回null
*/
@SuppressWarnings("unchecked")
public T getAnnotation(final Class annotationClass) {
return (T) declaredAnnotations.get(annotationClass);
}
/**
* 获取定义当前参数的方法.
*
* @return 定义方法
*/
public Method getDeclaringMethod() {
return this.declaringMethod;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy