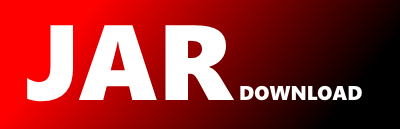
etc.example.mybatis-mapper-template.xml Maven / Gradle / Ivy
<?xml version="1.0" encoding="UTF-8" ?> <!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd" > <mapper namespace="support2"> <!-- ============================== 动态过滤,排序支持 ============================== --> <!-- 动态过滤条件集合 默认过滤条件集合为 _filters 如果需要遍历其他 filters 对象 <bind name="_oldFilters" value="_filters" /> <bind name="_filters" value="secondFilter" /> 设置要遍历 secondFilters <include refid="support2.dynamicFilters" /> --> <sql id="dynamicFilters"> <if test="_filters != null"> <foreach item="filter" collection="_filters" separator=" AND "> <include refid="support2.foreachFilter"/> </foreach> </if> </sql> <!-- 动态过滤条件 过滤条件属性 filter 属性前缀为 空 如果需要遍历其他, 可通过 bind 实现, 参考 dynamicFilters 注释 如果需要为每个过滤属性追加前缀: <bind name="_filterPrefix" value="'ROOT.'" /> <include refid="support2.dynamicFilters" /> <bind name="_filterPrefix" value="''" /> 还原前缀 --> <sql id="foreachFilter"> <foreach item="value" collection="filter" open="(" close=")" separator=" OR "> <!-- 忽略大小写时记得建立大/小写索引 --> <!-- 大写索引的创建 CREATE INDEX WS_NAME_IDX ON TB_WEBSITE(UPPER(NAME)); --> <!-- LIKE 索引失效问题求解决 --> <choose> <!-- WARN: mybatis 3.3.0 更新了 OGNL 产生抽象枚举比较错误 已经提交: https://github.com/jkuhnert/ognl/issues/15 mybaits 3.2.8 没有问题 临时解决方案 Operator.name() == 'NE' --> <when test="filter.operator.name() == 'LK'"> UPPER(${_filterPrefix}${filter.property}) ${filter.operator} UPPER(#{value}) </when> <when test="filter.operator.name() == 'BW'"> UPPER(${_filterPrefix}${filter.property}) ${filter.operator} UPPER(#{value}) </when> <when test="filter.operator.name() == 'BN'"> UPPER(${_filterPrefix}${filter.property}) ${filter.operator} UPPER(#{value}) </when> <when test="filter.operator.name() == 'EW'"> UPPER(${_filterPrefix}${filter.property}) ${filter.operator} UPPER(#{value}) </when> <when test="filter.operator.name() == 'EN'"> UPPER(${_filterPrefix}${filter.property}) ${filter.operator} UPPER(#{value}) </when> <when test="filter.operator.name() == 'CN'"> UPPER(${_filterPrefix}${filter.property}) ${filter.operator} UPPER(#{value}) </when> <when test="filter.operator.name() == 'NC'"> UPPER(${_filterPrefix}${filter.property}) ${filter.operator} UPPER(#{value}) </when> <when test="filter.operator.name() == 'NU'"> ${_filterPrefix}${filter.property} IS NULL </when> <when test="filter.operator.name() == 'NN'"> ${_filterPrefix}${filter.property} IS NOT NULL </when> <!-- <when test="filter.operator == @org.ponly.webbase.domain.Filters$Operator@LK"> UPPER(${_filterPrefix}${filter.property}) ${filter.operator} UPPER(#{value}) </when> <when test="filter.operator == @org.ponly.webbase.domain.Filters$Operator@BW"> UPPER(${_filterPrefix}${filter.property}) ${filter.operator} UPPER(#{value}) </when> <when test="filter.operator == @org.ponly.webbase.domain.Filters$Operator@BN"> UPPER(${_filterPrefix}${filter.property}) ${filter.operator} UPPER(#{value}) </when> <when test="filter.operator == @org.ponly.webbase.domain.Filters$Operator@EW"> UPPER(${_filterPrefix}${filter.property}) ${filter.operator} UPPER(#{value}) </when> <when test="filter.operator == @org.ponly.webbase.domain.Filters$Operator@EN"> UPPER(${_filterPrefix}${filter.property}) ${filter.operator} UPPER(#{value}) </when> <when test="filter.operator == @org.ponly.webbase.domain.Filters$Operator@CN"> UPPER(${_filterPrefix}${filter.property}) ${filter.operator} UPPER(#{value}) </when> <when test="filter.operator == @org.ponly.webbase.domain.Filters$Operator@NC"> UPPER(${_filterPrefix}${filter.property}) ${filter.operator} UPPER(#{value}) </when> <when test="filter.operator == @org.ponly.webbase.domain.Filters$Operator@NU"> ${_filterPrefix}${filter.property} IS NULL </when> <when test="filter.operator == @org.ponly.webbase.domain.Filters$Operator@NN"> ${_filterPrefix}${filter.property} IS NOT NULL </when> --> <otherwise> ${_filterPrefix}${filter.property} ${filter.operator} #{value} </otherwise> </choose> </foreach> </sql> <!-- 动态排序 默认排序属性为 _sort 默认排序字段前缀为空 如果需要 <bind name="_oldSort" value="_sort" /> <bind name="_sort" value="secondSort" /> <bind name="_sortPrefix" value="'PREFIX'" /> <include refid="support2.dynamicSort" /> <bind name="_sortPrefix" value="''" /> --> <sql id="dynamicSort"> <if test="_sort != null"> <foreach item="order" collection="_sort" separator=","> ${_sortPrefix}${order.property} ${order.direction} </foreach> </if> </sql> <!-- ========================= UUID 支持 ============================ --> <sql id="uuid"> <choose> <when test="_databaseId == 'mysql'"> <include refid="support2.__mysqlUUID"/> </when> <otherwise> <include refid="support2.__oracleGUID"/> </otherwise> </choose> </sql> <sql id="__mysqlUUID">SELECT UUID()</sql> <sql id="__oracleGUID">SELECT SYS_GUID() FROM DUAL</sql> <!-- ================================ 分页支持 ==================================== --> <!-- 分页头, 仅为兼容,请不要再使用 --> <sql id="pagingHeader"> <if test="_offset != null and _maxResults != null"> SELECT * FROM ( </if> </sql> <!-- 分页尾, 仅为兼容,请不要再使用 --> <sql id="pagingFooter"> <if test="_offset != null and _maxResults != null"> ) R LIMIT #{_offset}, #{_maxResults} </if> </sql> <!-- id相同时,可以通过 databaseId 自动匹配, 但是id 相同 IDE 报错不爽 --> <sql id="ph"> <if test="_offset != null and _maxResults != null"> <choose> <when test="_databaseId == 'mysql'"> <include refid="support2.__mysqlPagingHeader"/> </when> <otherwise> <include refid="support2.__oraclePagingHeader"/> </otherwise> </choose> </if> </sql> <!-- 分页尾 --> <sql id="pf"> <if test="_offset != null and _maxResults != null"> <choose> <when test="_databaseId == 'mysql'"> <include refid="support2.__mysqlPagingFooter"/> </when> <otherwise> <include refid="support2.__oraclePagingFooter"/> </otherwise> </choose> </if> </sql> <!-- oracle paging wrapper --> <sql id="__oraclePagingHeader"> SELECT * FROM ( SELECT R.*, ROWNUM RN FROM ( </sql> <sql id="__oraclePagingFooter"> ) R WHERE ROWNUM <![CDATA[ <= ]]> #{_offset} + #{_maxResults} ) P WHERE P.RN <![CDATA[ > ]]> #{_offset} </sql> <!-- mysql paging wrapper --> <sql id="__mysqlPagingHeader"> SELECT * FROM ( </sql> <sql id="__mysqlPagingFooter"> ) R LIMIT #{_offset}, #{_maxResults} </sql> <!-- =================== ===================== --> <!-- 有问题, 实现不了, 暂时不用 --> <sql id="dynamicGroupedFilter"> <if test="_grouped_filter != null and (_grouped_filter.rules.size > 0 or _grouped_filter.groups.size > 0)"> ( <!-- 遍历所有规则 --> <if test="_grouped_filter.rules.size > 0"> <foreach item="rule" collection="_grouped_filter.rules" index="i"> <!-- separator 不能使用变量 --> <if test="0 lt i">${_grouped_filter.op}</if> <choose> <when test="rule.op == @Rule$Op@NU or rule.op == @Rule$Op@NN"> ${rule.property} ${rule.op} </when> <otherwise> ${rule.property} ${rule.op} #{rule.value} </otherwise> </choose> </foreach> <if test="_grouped_filter.groups != null and _grouped_filter.groups.size > 0">${_grouped_filter.op}</if> </if> <if test="_grouped_filter.groups != null and _grouped_filter.groups.size > 0"> <foreach item="_grouped_filter" collection="_grouped_filter.groups" separator=" ${_grouped_filter.op} "> <!-- mybatis 是先包含在执行, 因此这里递归包含会死循环 --> <!--<include refid="support2.dynamicGroupedFilter" />--> <if test="_grouped_filter.rules.size > 0"> ( <foreach item="rule" collection="_grouped_filter.rules" index="i"> <!-- separator 不能使用变量 --> <if test="0 lt i">${_grouped_filter.op}</if> <choose> <when test="rule.op == @Rule$Op@NU or rule.op == @Rule$Op@NN"> ${rule.property} ${rule.op} </when> <otherwise> ${rule.property} ${rule.op} #{rule.value} </otherwise> </choose> </foreach> ) </if> </foreach> </if> ) </if> </sql> </mapper>
© 2015 - 2025 Weber Informatics LLC | Privacy Policy