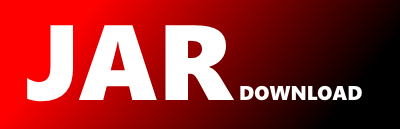
com.github.nomou.log4web.LogWatcher Maven / Gradle / Ivy
/*
* Copyright (c) 2005, 2014 vacoor
*
* Licensed under the Apache License, Version 2.0 (the "License");
*/
package com.github.nomou.log4web;
import com.github.nomou.log4web.cfg.ListenerConfig;
import com.github.nomou.log4web.cfg.LogWatcherConfig;
import com.github.nomou.log4web.history.CircularList;
import com.github.nomou.log4web.internal.jul.JulWatcher;
import com.github.nomou.log4web.internal.log4j.Log4jWatcher;
import com.github.nomou.log4web.internal.logback.LogbackWatcher;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.slf4j.impl.StaticLoggerBinder;
import java.io.PrintWriter;
import java.io.StringWriter;
import java.lang.reflect.Constructor;
import java.lang.reflect.InvocationTargetException;
import java.lang.reflect.UndeclaredThrowableException;
import java.util.ArrayList;
import java.util.Collection;
import java.util.List;
import java.util.Map;
import java.util.concurrent.atomic.AtomicBoolean;
/**
* Log watcher.
*
* @param the log event
* @author vacoor
*/
public abstract class LogWatcher {
/**
* The time of log.
*/
public static final String TIME_KEY = "time";
/**
* The level of log.
*/
public static final String LEVEL_KEY = "level";
/**
* The logger name of log.
*/
public static final String LOGGER_KEY = "logger";
/**
* The message of log.
*/
public static final String MESSAGE_KEY = "message";
/**
* The stack trace of log.
*/
public static final String TRACE_KEY = "trace";
/**
* Logger.
*/
private static final Logger LOGGER = LoggerFactory.getLogger(LogWatcher.class);
/**
* SLF4j-log4j factory.
*/
private static final String LOG4J_LOGGER_FACTORY = "org.slf4j.impl.Log4jLoggerFactory";
/**
* SLF4j-logback-classic factory.
*/
private static final String LOGBACK_CLASSIC_LOGGER_FACTORY = "ch.qos.logback.classic.util.ContextSelectorStaticBinder";
/**
* The history list for log.
*/
private CircularList history;
/**
* The last time for log.
*/
private long last = -1L;
/**
* Gets the watcher name.
*
* @return the watcher name
*/
public abstract String getName();
/**
* Gets the watcher threshold level.
*
* @return the watcher threshold level
*/
public abstract String getThreshold();
/**
* Sets the watcher threshold level.
*/
public abstract void setThreshold(final String level);
/**
* Gets the all levels of log for the current log implementation.
*
* @return the levels of log
*/
public abstract List getAllLevels();
/**
* Gets the configured loggers.
*
* @return the loggers info
*/
public abstract Collection getAllLoggers();
/**
* Sets level for the given category.
*
* @param category the category for logger
* @param level the level of log
*/
public abstract void setLogLevel(final String category, final String level);
/**
* Adds the log event to watcher.
*
* @param event the log event
* @param millis the happen time (ms)
*/
public void add(final E event, final long millis) {
this.history.add(event);
this.last = millis;
}
/**
* @return
*/
public long getLastEvent() {
return this.last;
}
public int getHistorySize() {
return this.history == null ? -1 : this.history.getBufferSize();
}
public List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy