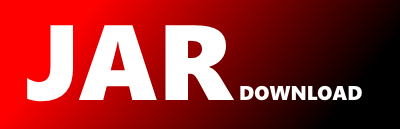
oriana.retry_scheduling.scala Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of oriana_2.11 Show documentation
Show all versions of oriana_2.11 Show documentation
Oriana is a small layer on top of slick that allows easier access to the database. It allows peudo-syntactic methods to inject a database context into arbitrary code, and simplifies deployment, updates and initialization.
The newest version!
package oriana
import com.typesafe.config.Config
import scala.collection.JavaConverters._
import scala.concurrent.duration.FiniteDuration
import scala.concurrent.duration._
/**
* Defines a retry schedule for failures of an [[DBTransaction]].
*/
trait RetrySchedule {
/**
* Determines the delay to execute the `retryCount`th retry of an operation
* @param retryCount retry count - how many times the operation has already been retried
* @return delay if another attempt should be made, None otherwise
*/
def retryDelay(retryCount: Int): Option[FiniteDuration]
}
/**
* Simple schedule that never attempts a retry
*/
object NoRetrySchedule extends RetrySchedule {
/**
* Always returns None
*
* @param retryCount ignored
* @return None
*/
override def retryDelay(retryCount: Int) = None
}
/**
* Attempts a fixed number of retries, with constant time delays
* @param retries retry time delays
*/
class FixedRetrySchedule(retries: FiniteDuration*) extends RetrySchedule {
private val schedule = retries.toArray
/**
* Returns the retry delay at position retryCount if present, None otherwise
* @param retryCount lookup location
* @return retry delay, if present at that index
*/
def retryDelay(retryCount: Int) = if (0 <= retryCount && schedule.length > retryCount) Some(schedule(retryCount)) else None
}
/**
* A configured schedule derives its delays from the config key "retry_db_delay_millis", where it expects to find a
* number list. The numbers are interpreted as millisecond delays.
* @param config (sub-)configuration searched for the "retry_db_delay_millis"
*/
class ConfiguredRetrySchedule(config: Config) extends FixedRetrySchedule({
config.getNumberList("retry_db_delay_millis").asScala.map(_.intValue.millis)
} : _*)
/**
* The default schedule retries five times, with escalating delays. The retries occur at 100, 200, 300, 400 and 500 ms
* intervals. This schedule is primarily beneficial to testing and demo environments, and should generally be replaced
* by a more considered schedule in applications.
*/
object DefaultSchedule extends FixedRetrySchedule({
for (i <- 1 to 5) yield i.millis * 100
} : _*)
© 2015 - 2025 Weber Informatics LLC | Privacy Policy