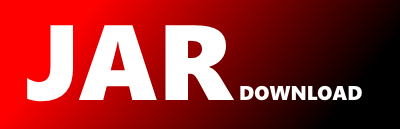
org.seasar.framework.convention.NamingConvention Maven / Gradle / Ivy
The newest version!
/*
* Copyright 2004-2015 the Seasar Foundation and the Others.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND,
* either express or implied. See the License for the specific language
* governing permissions and limitations under the License.
*/
package org.seasar.framework.convention;
/**
* 命名規約のためのインターフェースです。
*
* @author higa
* @author shot
*/
public interface NamingConvention {
/**
* view
のルートパスを返します。
*
* @return view root path
*/
String getViewRootPath();
/**
* view
のルートパスが/のみの場合に取り除きます。 例:"/" -> "", "/hoge" -> "/hoge"
*
* @return adjusted view root path
*/
String adjustViewRootPath();
/**
* view
の拡張子を返します。
*
* @return view extension
*/
String getViewExtension();
/**
* 実装クラスのsuffix
を返します。
*
* @return 実装クラスのsuffix
*/
String getImplementationSuffix();
/**
* Page
クラスのsuffix
を返します。
*
* @return Page
クラスのsuffix
*/
String getPageSuffix();
/**
* Action
クラスのsuffix
を返します。
*
* @return Action
クラスのsuffix
*/
String getActionSuffix();
/**
* Service
クラスのsuffix
を返します。
*
* @return Service
クラスのsuffix
*/
String getServiceSuffix();
/**
* Dxo
クラスのsuffix
を返します。
*
* @return Dxo
クラスのsuffix
*/
String getDxoSuffix();
/**
* Logic
クラスのsuffix
を返します。
*
* @return Logic
クラスのsuffix
*/
String getLogicSuffix();
/**
* Dao
クラスのsuffix
を返します。
*
* @return Dao
クラスのsuffix
*/
String getDaoSuffix();
/**
* Helper
クラスのsuffix
を返します。
*
* @return Helper
クラスのsuffix
*/
String getHelperSuffix();
/**
* Interceptor
クラスのsuffix
を返します。
*
* @return Interceptor
クラスのsuffix
*/
String getInterceptorSuffix();
/**
* Validator
クラスのsuffix
を返します。
*
* @return Validator
クラスのsuffix
*/
String getValidatorSuffix();
/**
* Converter
クラスのsuffix
を返します。
*
* @return Converter
クラスのsuffix
*/
String getConverterSuffix();
/**
* Dto
クラスのsuffix
を返します。
*
* @return Dto
クラスのsuffix
*/
String getDtoSuffix();
/**
* Connector
クラスのsuffix
を返します。
*
* @return Connector
クラスのsuffix
*/
String getConnectorSuffix();
/**
* サブアプリケーションのルートパッケージ名を返します。
*
* @return サブアプリケーションのルートパッケージ名
*/
String getSubApplicationRootPackageName();
/**
* 実装用のパッケージ名を返します。
*
* @return 実装用のパッケージ名
*/
String getImplementationPackageName();
/**
* Dxo
クラスのパッケージ名を返します。
*
* @return Dxo
クラスのパッケージ名
*/
String getDxoPackageName();
/**
* Logic
クラスのパッケージ名を返します。
*
* @return Logic
クラスのパッケージ名
*/
String getLogicPackageName();
/**
* Dao
クラスのパッケージ名を返します。
*
* @return Dao
クラスのパッケージ名
*/
String getDaoPackageName();
/**
* Entity
クラスのパッケージ名を返します。
*
* @return Entity
クラスのパッケージ名
*/
String getEntityPackageName();
/**
* Dto
クラスのパッケージ名を返します。
*
* @return Dto
クラスのパッケージ名
*/
String getDtoPackageName();
/**
* Service
クラスのパッケージ名を返します。
*
* @return Service
クラスのパッケージ名
*/
String getServicePackageName();
/**
* Interceptor
クラスのパッケージ名を返します。
*
* @return Interceptor
クラスのパッケージ名
*/
String getInterceptorPackageName();
/**
* Validator
クラスのパッケージ名を返します。
*
* @return Validator
クラスのパッケージ名
*/
String getValidatorPackageName();
/**
* Converter
クラスのパッケージ名を返します。
*
* @return Converter
クラスのパッケージ名
*/
String getConverterPackageName();
/**
* Helper
クラスのパッケージ名を返します。
*
* @return Helper
クラスのパッケージ名
*/
String getHelperPackageName();
/**
* Connector
クラスのパッケージ名を返します。
*
* @return Connector
クラスのパッケージ名
*/
String getConnectorPackageName();
/**
* ルートパッケージ名の配列を返します。
*
* @return ルートパッケージ名の配列
*/
String[] getRootPackageNames();
/**
* 無視するルートパッケージ名の配列を返します。
*
* @return 無視するルートパッケージ名の配列
*/
String[] getIgnorePackageNames();
/**
* suffix
をパッケージ名に変換します。
*
* @param suffix suffix
* @return パッケージ名
*/
String fromSuffixToPackageName(String suffix);
/**
* クラス名を短いコンポーネント名に変換します。 短いコンポーネント名とは、"サブアプリケーション名_"がついていないコンポーネント名です。
*
* @param className className
* @return 短いコンポーネント名
*/
String fromClassNameToShortComponentName(String className);
/**
* クラス名をコンポーネント名に変換します。
*
* @param className className
* @return コンポーネント名
*/
String fromClassNameToComponentName(String className);
/**
* コンポーネント名を{@link Class}に変換します。
*
* @param componentName componentName
* @return {@link Class}
*/
Class> fromComponentNameToClass(String componentName);
/**
* クラス名を実装クラス名に変換します。
*
* @param className className
* @return 実装クラス名
*/
String toImplementationClassName(String className);
/**
* クラス名をインターフェース名に変換します。
*
* @param className className
* @return インターフェース名
*/
String toInterfaceClassName(String className);
/**
* 規約に従っていないスキップすべきクラスかどうか返します。
*
* @param clazz clazz
* @return 規約に従っていないスキップすべきクラス
*/
boolean isSkipClass(Class> clazz);
/**
* 最終的に利用されるクラスに変換します。 通常は、実装クラスですが、DaoのようにInterceptorで実体化される場合、
* インターフェースの場合もあります。
*
* @param clazz clazz
* @return 最終的に利用されるクラス
*/
Class> toCompleteClass(Class> clazz);
/**
* コンポーネント名をクラス名の一部に変換します。 "_"は"."に"_"の後ろは大文字に変換されます。
* 例えば、コンポーネント名がhoge_fooの場合、hoge.Fooになります。
*
* @param componentName componentName
* @return クラス名の一部
*/
String fromComponentNameToPartOfClassName(String componentName);
/**
* コンポーネント名をsuffix
に変換します。 コンポーネント名の最後から探して最初の大文字までを抽出して、
* 先頭を小文字に変換したものが、 suffix
になります。
*
* @param componentName componentName
* @return suffix
*/
String fromComponentNameToSuffix(String componentName);
/**
* クラス名をsuffix
に変換します。
*
* @param className className
* @return suffix
*/
String fromClassNameToSuffix(String className);
/**
* View
のパスをページ名に変換します。
*
* @param path path
* @return ページ名
*/
String fromPathToPageName(String path);
/**
* View
のパスをアクション名に変換します。
*
* @param path path
* @return アクション名
*/
String fromPathToActionName(String path);
/**
* ページ名をView
のパスに変換します。
*
* @param pageName pageName
* @return View
のパス
*/
String fromPageNameToPath(String pageName);
/**
* ページの{@link Class}をView
のパスに変換します。
*
* @param pageClass pageClass
* @return View
のパス
*/
String fromPageClassToPath(Class> pageClass);
/**
* アクション名をView
のパスに変換します。
*
* @param actionName actionName
* @return View
のパス
*/
String fromActionNameToPath(String actionName);
/**
* アクション名をページ名に変換します。
*
* @param actionName actionName
* @return ページ名
*/
String fromActionNameToPageName(String actionName);
/**
* ターゲットのクラス名かどうかを返します。
*
* @param className className
* @param suffix suffix
* @return ターゲットのクラス名かどうか
*/
boolean isTargetClassName(String className, String suffix);
/**
* ターゲットのクラス名かどうかを返します。
*
* @param className className
* @return ターゲットのクラス名かどうか
*/
boolean isTargetClassName(String className);
/**
* HOT deployのターゲットのクラス名かどうかを返します。
*
* @param className className
* @return HOT deployのターゲットのクラス名かどうか
*/
boolean isHotdeployTargetClassName(String className);
/**
* 無視するクラス名かどうかを返します。
*
* @param className className
* @return 無視するクラス名かどうか
*/
boolean isIgnoreClassName(String className);
/**
* 妥当なView
のルートパスかどうかを返します。
*
* @param path path
* @return 妥当なView
のルートパスかどうか
*/
boolean isValidViewRootPath(String path);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy