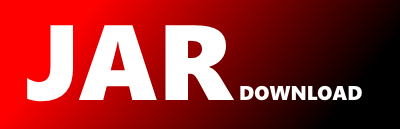
com.github.nstdio.eitheradapter.EitherCallAdapterFactory Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of retrofit-either-adapter Show documentation
Show all versions of retrofit-either-adapter Show documentation
A Retrofit2 Either call adapter.
package com.github.nstdio.eitheradapter;
import com.github.nstdio.eitheradapter.annotation.InvocationPolicy;
import okhttp3.ResponseBody;
import retrofit2.Call;
import retrofit2.CallAdapter;
import retrofit2.Converter;
import retrofit2.Retrofit;
import java.lang.annotation.Annotation;
import java.lang.reflect.ParameterizedType;
import java.lang.reflect.Type;
public final class EitherCallAdapterFactory extends CallAdapter.Factory {
public static final Annotation[] ANNOTATIONS = new Annotation[0];
private EitherCallAdapterFactory() {
}
public static EitherCallAdapterFactory create() {
return new EitherCallAdapterFactory();
}
public CallAdapter, ?> get(Type returnType, Annotation[] annotations, Retrofit retrofit) {
final Class> rawType = getRawType(returnType);
if (rawType != EitherCall.class) {
return null;
}
if (!(returnType instanceof ParameterizedType)) {
throw new IllegalStateException("EitherCall return type must be parameterized"
+ " as EitherCall or EitherCall extends Foo>");
}
final Type leftType = getParameterUpperBound(0, (ParameterizedType) returnType);
final Type rightType = getParameterUpperBound(1, (ParameterizedType) returnType);
final Converter left = retrofit.responseBodyConverter(leftType, ANNOTATIONS);
final Converter right = retrofit.responseBodyConverter(rightType, ANNOTATIONS);
final InvocationPolicy statusCode = annotated(annotations);
return new EitherCallAdapter(left, right, statusCode);
}
private InvocationPolicy annotated(Annotation[] annotations) {
for (Annotation annotation : annotations) {
if (annotation instanceof InvocationPolicy) {
return (InvocationPolicy) annotation;
}
}
return new InvocationPolicy() {
@Override
public Class extends Annotation> annotationType() {
return InvocationPolicy.class;
}
@Override
public int[] left() {
return new int[0];
}
@Override
public int[] right() {
return new int[0];
}
@Override
public StatusCodeRange[] leftRange() {
return new StatusCodeRange[]{StatusCodeRange.SUCCESS, StatusCodeRange.REDIRECT};
}
@Override
public StatusCodeRange[] rightRange() {
return new StatusCodeRange[]{StatusCodeRange.CLIENT_ERROR, StatusCodeRange.SERVER_ERROR};
}
};
}
private class EitherCallAdapter implements CallAdapter> {
private final Converter left;
private final Converter right;
private final InvocationPolicy statusCode;
private EitherCallAdapter(Converter left,
Converter right, InvocationPolicy statusCode) {
this.left = left;
this.right = right;
this.statusCode = statusCode;
}
public Type responseType() {
return ResponseBody.class;
}
@Override
public EitherCall adapt(Call call) {
return new EitherCall(call, left, right, statusCode);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy