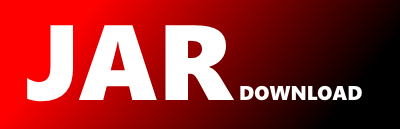
gedi.solutions.geode.io.function.GetAllRegionValuesFunction Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gedi-geode-extensions-core Show documentation
Show all versions of gedi-geode-extensions-core Show documentation
GemFire Enterprise Data Integration - common development extensions powered by Apache Geode
The newest version!
Please wait ...
© 2015 - 2025 Weber Informatics LLC | Privacy Policy