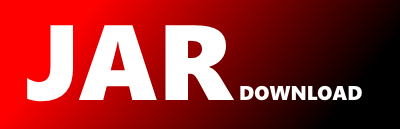
gedi.solutions.geode.lucene.GeodePagination Maven / Gradle / Ivy
package gedi.solutions.geode.lucene;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Map;
import java.util.TreeSet;
import org.apache.geode.cache.Region;
import gedi.solutions.geode.io.Querier;
import nyla.solutions.core.util.BeanComparator;
import nyla.solutions.core.util.Organizer;
public class GeodePagination
{
/**
*
* @param id the unique ID
* @param pageNumber the page number
* @param pageRegion the page region
* @return the collection
*/
public Collection> readKeys(String id, int pageNumber ,Region> pageRegion)
{
String pageKey = toPageKey(id, pageNumber);
return pageRegion.get(pageKey);
}// --------------------------------------------------------------
/**
* Store the pagination search result details
* @param the key class
* @param the value class
* @param id the unique id
* @param pageSize the page size
* @param pageKeysRegion the region where pages keys are stors
* @param results the collection os results
* @return the list of keys in the page region
*/
@SuppressWarnings({ "unchecked", "rawtypes" })
public List storePaginationMap(String id,int pageSize,
Region> pageKeysRegion,
List> results)
{
if(results == null || results.isEmpty())
return null;
//add to pages
List> pagesCollection = toKeyPages((List)results, pageSize);
int pageIndex = 1;
String key = null;
ArrayList keys = new ArrayList(pageSize);
for (Collection page : pagesCollection)
{
//store in region
key = toPageKey(id,pageIndex++);
pageKeysRegion.put(key, page);
keys.add(key);
}
keys.trimToSize();
return keys;
}// --------------------------------------------------------------
/***
*
* @param id the ID
* @param pageNumber the page number
* @return the id-pageNumber
*/
public static String toPageKey(String id,int pageNumber)
{
return new StringBuilder().append(id).append("-").append(pageNumber).toString();
}// --------------------------------------------------------------
/**
* @param the key class
* @param the value class
* @param mapEntries the map entries
* @param pageSize the page size
* @return the collection of key
*/
@SuppressWarnings({ "unchecked", "rawtypes" })
public static List> toKeyPages(List> mapEntries,int pageSize)
{
if(mapEntries == null || mapEntries.isEmpty())
return null;
int collectionSize = mapEntries.size();
if(pageSize <= 0 || collectionSize <= pageSize)
{
ArrayList list = new ArrayList(mapEntries.size());
for (Map.Entry entry : mapEntries)
{
if(entry == null)
continue;
list.add(entry.getKey());
}
if(list.isEmpty())
return null;
return Collections.singletonList(list);
}
int initialSize = collectionSize /pageSize;
ArrayList> list = new ArrayList(initialSize);
ArrayList current = new ArrayList();
for (Map.Entry entry : mapEntries)
{
current.add(entry.getKey());
if(current.size() >= pageSize)
{
current.trimToSize();
list.add((Collection)current);
current = new ArrayList();
}
}
if(!current.isEmpty())
list.add((Collection)current);
return (List>)list;
}//------------------------------------------------
public List storePagination(String sanId, int pageSize,
Map> pageKeysRegion,
Collection keys)
{
if(keys == null || keys.isEmpty())
return null;
//add to pages
List> pagesCollection = Organizer.toPages(keys, pageSize);
String key = null;
int pageIndex = 1;
ArrayList pageKeys = new ArrayList(10);
for (Collection page : pagesCollection)
{
//store in region
key = new StringBuilder().append(sanId).append("-").append(pageIndex++).toString();
pageKeysRegion.put(key, page);
pageKeys.add(key);
}
pageKeys.trimToSize();
return pageKeys;
}
/**
* Read Results from region by keys in pageRegion
* @param the key class
* @param the value class
* @param criteria the text page criteria
* @param pageNumber the page number to retrieve
* @param region the region where data is retrieved
* @param pageRegion the page region with key contains the pageKey and value the keys to the region
* @return region.getAll(pageKey)
*/
public Map readResultsByPage(TextPageCriteria criteria, int pageNumber, Region region, Region> pageRegion)
{
if(pageRegion == null )
return null;
Collection> regionKeys = pageRegion.get(criteria.toPageKey(pageNumber));
if(regionKeys == null|| regionKeys.isEmpty())
return null;
return region.getAll(regionKeys);
}
@SuppressWarnings("unchecked")
public Collection readResultsByPageValues(String criteriaId, String sortField, boolean desc,int pageNumber, Region region, Region> pageRegion)
{
if(pageRegion == null )
return null;
Collection regionKeys = (Collection)pageRegion.get(criteriaId+"-"+pageNumber);
if(regionKeys == null|| regionKeys.isEmpty())
return null;
if(sortField != null)
{
String field = sortField.replace("entry.", "");
BeanComparator c = new BeanComparator(field,desc);
TreeSet set = new TreeSet(c);
for (K key : regionKeys)
{
set.add(region.get(key));
}
return set;
}
else
{
ArrayList list = new ArrayList();
for (K key : regionKeys)
{
list.add(region.get(key));
}
return list;
}
}//------------------------------------------------
public Collection clearSearchResultsByPage(TextPageCriteria criteria, Region> pageRegion)
{
///TODO: Get previous page numbers faster (without a query)
Collection pageKeys = Querier.query("select * from /"+criteria.getPageRegionName()+".keySet() k where k like '"+criteria.getId()+"%'");
if(pageKeys == null || pageKeys.isEmpty())
return null;
pageRegion.removeAll(pageKeys);
return pageKeys;
}//------------------------------------------------
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy