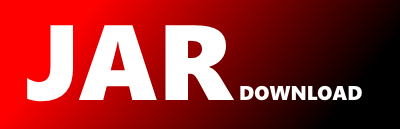
nyla.solutions.dao.ResultSetDataRow Maven / Gradle / Ivy
package nyla.solutions.dao;
import java.sql.Blob;
import java.sql.Clob;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import nyla.solutions.global.data.Arrayable;
import nyla.solutions.global.data.DataRow;
import nyla.solutions.global.util.Debugger;
/**
* Represents an entity row
* @author Gregory Green
*
*/
public class ResultSetDataRow extends DataRow implements Arrayable
© 2015 - 2025 Weber Informatics LLC | Privacy Policy