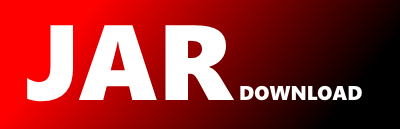
nyla.solutions.dao.patterns.command.ExecuteUpdateArrayableCommand Maven / Gradle / Ivy
package nyla.solutions.dao.patterns.command;
import java.sql.PreparedStatement;
import java.sql.SQLException;
import nyla.solutions.global.data.Arrayable;
import nyla.solutions.global.exception.SystemException;
/**
* Example
*
true
select 'Y' from ASAP_STRUCTURES where cdregno = ?
1
* @author Gregory Green
*
*/
public class ExecuteUpdateArrayableCommand extends AbstractDAOCommand>
{
/**
*
* @see solutions.global.patterns.command.Command#execute(java.lang.Object)
*/
public Integer execute(Arrayable
© 2015 - 2025 Weber Informatics LLC | Privacy Policy