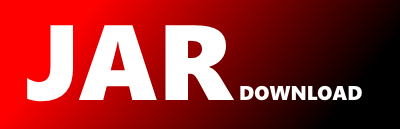
nyla.solutions.global.patterns.command.commas.ShellMgr Maven / Gradle / Ivy
package nyla.solutions.global.patterns.command.commas;
import java.lang.reflect.InvocationHandler;
import java.lang.reflect.Method;
import java.util.Collection;
import nyla.solutions.global.exception.NoDataFoundException;
import nyla.solutions.global.exception.RequiredException;
import nyla.solutions.global.patterns.command.Command;
import nyla.solutions.global.security.SecuredToken;
import nyla.solutions.global.util.Debugger;
public class ShellMgr implements Shell, InvocationHandler
{
/**
*
* @param commasName the shell name
*/
protected ShellMgr(String commasName, Shell shell)
{
this(commasName,shell,null);
}// -----------------------------------------------
/**
*
* @param commasName the shell name
*/
protected ShellMgr(String commasName, Shell shell, SecuredToken securedToken)
{
if (commasName == null)
throw new
RequiredException("commasName");
if(shell == null)
throw new RequiredException("shell");
this.name = commasName;
this.shell = shell;
this.securedToken = securedToken;
}// -----------------------------------------------
/**
* Implementation for the InvocationHandler to execute functions
*/
@Override
public Object invoke(Object proxy, Method method, Object[] args)
throws Throwable
{
String methodName = method.getName();
Debugger.println(this,"ShellMgr.invoke method="+methodName);
if(!(proxy instanceof Shell) && !"toString".equals(methodName))
{
return executeMethodCommand(args, methodName);
}
else if(methodName.matches("executeFunction|getFunction|listFunction|toString|getName"))
{
//special shell function
if("executeFunction".equals(methodName))
{
if(args == null || args.length != 2)
throw new RequiredException("Function name and request for method "+methodName);
String commandName = (String)args[0];
Object request = args[1];
return this.executeCommand(commandName, request);
}
else if("getFunction".equals(methodName))
{
if(args == null || args.length == 0)
throw new IllegalArgumentException("args required for method call "+methodName);
int argsLength = args.length;
switch(argsLength)
{
case 1: return this.getCommand((String)args[0]);
case 2: return this.getCommand((String)args[0],args[1] );
default: throw new IllegalArgumentException("Invalid number of arguments for getFunction method");
}
}
else if("listFunction".equals(methodName))
{
return this.getCommands();
}
else if("toString".equals(methodName))
{
return method.getDeclaringClass().getName()+"."+method.getName();
}
else if("getName".equals(methodName))
{
return this.name;
}
}
return executeMethodCommand(args, methodName);
}// -----------------------------------------------
/**
*
* @param args the method arguments
* @param methodName the method to execute
* @return the method's return
*/
private Object executeMethodCommand(Object[] args, String methodName)
{
Command
© 2015 - 2025 Weber Informatics LLC | Privacy Policy