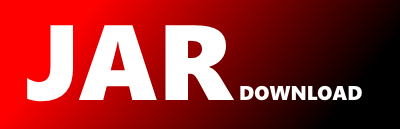
nyla.solutions.global.patterns.command.file.ReLookupCommand Maven / Gradle / Ivy
package nyla.solutions.global.patterns.command.file;
import java.io.File;
import java.util.Map;
import java.util.TreeMap;
import nyla.solutions.global.exception.RequiredException;
import nyla.solutions.global.operations.logging.Log;
import nyla.solutions.global.util.Config;
import nyla.solutions.global.util.Debugger;
import nyla.solutions.global.util.Text;
/**
* Uses complex regular expressions to the determine nested FileCommand to execute
* @author Gregory Green
*
*/
public class ReLookupCommand implements FileCommand
{
/**
*
* Constructor for ReLookupCommand initializes internal
*/
public ReLookupCommand()
{
}// --------------------------------------------
/**
* Executes a nested file command if the file name matches a regular expression in the lookup table.
* @param file the file to process
*
*/
public synchronized Void execute(File file)
{
log.debug("Entering the transform method");
String re = null;
//loop thru values
String fileName = file.getName();
FileCommand
© 2015 - 2025 Weber Informatics LLC | Privacy Policy