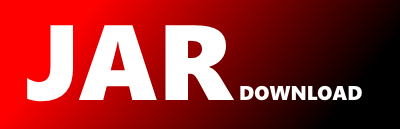
nyla.solutions.global.patterns.command.remote.partitioning.RmiAllRoutesAdvice Maven / Gradle / Ivy
package nyla.solutions.global.patterns.command.remote.partitioning;
import java.io.Serializable;
import java.net.URI;
import java.net.URISyntaxException;
import java.rmi.RemoteException;
import java.util.ArrayList;
import java.util.Collection;
import java.util.HashMap;
import java.util.HashSet;
import java.util.List;
import java.util.Map;
import java.util.Set;
import java.util.TreeSet;
import java.util.concurrent.Callable;
import javax.annotation.concurrent.NotThreadSafe;
import nyla.solutions.global.data.Envelope;
import nyla.solutions.global.exception.CommunicationException;
import nyla.solutions.global.exception.SetupException;
import nyla.solutions.global.net.rmi.RMI;
import nyla.solutions.global.patterns.SetUpable;
import nyla.solutions.global.patterns.aop.Advice;
import nyla.solutions.global.patterns.command.Command;
import nyla.solutions.global.patterns.command.commas.CatalogClassInfo;
import nyla.solutions.global.patterns.command.commas.CommandAttribute;
import nyla.solutions.global.patterns.command.commas.CommandFacts;
import nyla.solutions.global.patterns.command.commas.CommasConstants;
import nyla.solutions.global.patterns.command.commas.annotations.Aspect;
import nyla.solutions.global.patterns.command.remote.RemoteCommand;
import nyla.solutions.global.patterns.command.remote.RemoteCommandProcessor;
import nyla.solutions.global.patterns.iteration.Paging;
import nyla.solutions.global.patterns.workthread.ExecutorBoss;
import nyla.solutions.global.util.Debugger;
import nyla.solutions.global.util.Organizer;
/**
* Used int Partition Data Services design implementation
* for RMI.
*
* - get lookup Id from payload
- get routing key by lookup Id and commasProperties
- lookup by routingKey URL
- configure routing information in Envelope
- Execute RemoteCommas
- skip over execute
Advice reference
@COMMAS
public class RealSingleRouteCommand
{
@CMD(advice=RmiAllRoutesAdvice.ADVICE_NAME)
public Collection findUsersEveryWhere(Criteria criteria)
{}
}
* @author Gregory Green
*
*/
@Aspect(name=RmiAllRoutesAdvice.ADVICE_NAME)
@NotThreadSafe
public class RmiAllRoutesAdvice implements Advice, SetUpable
{
private enum RmiAllRoutesAdviceReturnType
{
collection,
set,
tree,
paging,
single
};
/**
* ADVICE_NAME = "RmiOneRouteAdvice"
*/
public static final String ADVICE_NAME = "RmiAllRoutesAdvice";
//initialize CommandFacts
public RmiAllRoutesAdvice()
{
}// --------------------------------------------------------
@Override
public synchronized void setUp()
{
if(this.registry != null)
return;
try
{
//this.factory = ServiceFactory.getInstance(this.getClass());
//this.registry = this.factory.create(PartitionCommasRemoteRegistry.class);
this.registry = PartitionCommasRemoteRegistry.getRegistry();
}
catch (RemoteException e)
{
throw new SetupException("Unable to get remote commas registry ",e);
}
}// --------------------------------------------------------
/**
* @return rmiOneRouteCommand
* @see nyla.solutions.global.patterns.aop.Advice#getBeforeCommand()
*/
@Override
public Command, ?> getBeforeCommand()
{
return new RmiAllRoutesCommand(this.commandFacts, this.returnType);
}// --------------------------------------------------------
/**
* No after processing
* @return null
* @see nyla.solutions.global.patterns.aop.Advice#getAfterCommand()
*/
@Override
public Command, ?> getAfterCommand()
{
return null;
}// --------------------------------------------------------
/**
*
* @see nyla.solutions.global.patterns.aop.Advice#getFacts()
* @return this.commandFacts;
*/
@Override
public CommandFacts getFacts()
{
return this.commandFacts;
}// --------------------------------------------------------
/**
* Add CommasProxyCommand.ADVISED_SKIP_METHOD_INVOKE_CMD_ATTRIB_NAME
* to command attributes
* @see nyla.solutions.global.patterns.aop.Advice#setFacts(nyla.solutions.global.patterns.command.commas.CommandFacts)
*/
@Override
public void setFacts(CommandFacts facts)
{
this.returnType = RmiAllRoutesAdviceReturnType.single;
CommandAttribute advisedSkipMethodInvoke = new CommandAttribute(
CommasConstants.ADVISED_SKIP_METHOD_INVOKE_CMD_ATTRIB_NAME,
Boolean.class.getName(), Boolean.TRUE.toString());
if(facts == null)
{
facts = new CommandFacts();
CommandAttribute[] commandAttributes = { advisedSkipMethodInvoke};
facts.setCommandAttributes(commandAttributes);
}
//process advisedSkipMethodInvoke
CommandAttribute[] commandAttributes = facts.getCommandAttributes();
if(commandAttributes == null)
{
CommandAttribute[] commandAttributesArray = { advisedSkipMethodInvoke};
facts.setCommandAttributes(commandAttributesArray);
}
else
{
//add advisedSkipMethodInvoke
commandAttributes= Organizer.add(advisedSkipMethodInvoke, commandAttributes);
facts.setCommandAttributes(commandAttributes);
}
this.commandFacts = facts;
//Determine return class type
CatalogClassInfo classInfo = this.commandFacts.getReturnClassInfo();
String className = classInfo.getBeanClassName();
if(Collection.class.getName().equals(className) ||
ArrayList.class.getName().equals(className) ||
List.class.getName().equals(className))
returnType = RmiAllRoutesAdviceReturnType.collection;
else if(Set.class.getName().equals(className))
returnType = RmiAllRoutesAdviceReturnType.set;
else if(Paging.class.getName().equals(className))
returnType = RmiAllRoutesAdviceReturnType.paging;
else if(TreeSet.class.getName().equals(className))
returnType = RmiAllRoutesAdviceReturnType.tree;
}// --------------------------------------------------------
/**
* Before process to make Remote RMI call and
* by pass local processing
* @author Gregory Green
*
*/
class RmiAllRoutesCommand implements Command>
{
RmiAllRoutesCommand(CommandFacts facts,RmiAllRoutesAdviceReturnType returnType)
{
this.commandFacts = facts;
this.returnType = returnType;
}// --------------------------------------------------------
@SuppressWarnings({ "unchecked", "rawtypes" })
@Override
public synchronized Serializable execute(Envelope env)
{
if(registry == null)
setUp();
//get lookup Id from payload
Collection locations = null;
try
{
//configure routing information in Envelope
Map
© 2015 - 2025 Weber Informatics LLC | Privacy Policy