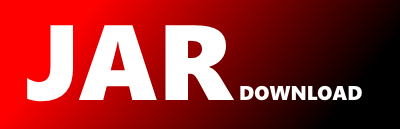
nyla.solutions.global.security.user.data.UserProfile Maven / Gradle / Ivy
package nyla.solutions.global.security.user.data;
import java.util.*;
import nyla.solutions.global.data.Copier;
import nyla.solutions.global.data.Criteria;
import nyla.solutions.global.util.Text;
/**
*
*
* UserProfile is a value object representation of the
* User entity and associated entities.
*
* @author Gregory Green
* @version 1.0
*/
public class UserProfile extends Criteria
implements User, Copier, Comparable
© 2015 - 2025 Weber Informatics LLC | Privacy Policy