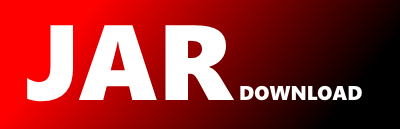
com.github.nylle.javafixture.specimen.SpecialSpecimen Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of javafixture Show documentation
Show all versions of javafixture Show documentation
JavaFixture is the attempt to bring Mark Seemann's AutoFixture for .NET to the Java world. Its purpose
is to generate full object graphs for use in test suites with a fluent API for customising the test objects
during generation.
package com.github.nylle.javafixture.specimen;
import com.github.nylle.javafixture.Context;
import com.github.nylle.javafixture.CustomizationContext;
import com.github.nylle.javafixture.ISpecimen;
import com.github.nylle.javafixture.PseudoRandom;
import com.github.nylle.javafixture.SpecimenType;
import java.io.File;
import java.lang.annotation.Annotation;
import java.math.BigDecimal;
import java.math.BigInteger;
import java.net.URI;
import java.net.URISyntaxException;
import java.util.Random;
import java.util.UUID;
public class SpecialSpecimen implements ISpecimen {
private static final int MAXIMUM_BITLENGTH_FOR_RANDOM_BIGINT = 1024;
private final SpecimenType type;
private final Context context;
public SpecialSpecimen(SpecimenType type, Context context) {
if (type == null) {
throw new IllegalArgumentException("type: null");
}
if (!type.isSpecialType()) {
throw new IllegalArgumentException("type: " + type.getName());
}
if (context == null) {
throw new IllegalArgumentException("context: null");
}
this.type = type;
this.context = context;
}
@Override
public T create(CustomizationContext customizationContext, Annotation[] annotations) {
if (type.asClass().equals(File.class)) {
return (T) new File(UUID.randomUUID().toString());
}
if (type.asClass().equals(BigInteger.class)) {
return (T) createBigInteger();
}
if (type.asClass().equals(BigDecimal.class)) {
return (T) createBigDecimal();
}
try {
return (T) new URI("https://localhost/" + UUID.randomUUID());
} catch (URISyntaxException e) {
return null;
}
}
private BigInteger createBigInteger() {
var rnd = new Random();
var result = new BigInteger(rnd.nextInt(MAXIMUM_BITLENGTH_FOR_RANDOM_BIGINT), new Random());
if (!context.getConfiguration().usePositiveNumbersOnly()) {
if (rnd.nextBoolean()) { // negate randomly
return result.negate();
}
}
return result;
}
private BigDecimal createBigDecimal() {
var bd = new BigDecimal(new PseudoRandom().nextLong(new Random().nextBoolean()));
if (context.getConfiguration().usePositiveNumbersOnly()) {
return bd.abs();
}
return bd;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy