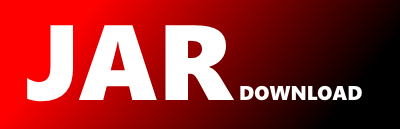
com.github.obase.mysql.impl.MysqlClientImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of obase-mysql Show documentation
Show all versions of obase-mysql Show documentation
A very simple/efficient Mysql Client Framework
The newest version!
package com.github.obase.mysql.impl;
import static com.github.obase.kit.StringKit.isNotEmpty;
import java.sql.Connection;
import java.sql.SQLException;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.regex.Pattern;
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
import org.springframework.core.io.Resource;
import org.springframework.core.io.support.PathMatchingResourcePatternResolver;
import org.springframework.core.io.support.ResourcePatternResolver;
import com.github.obase.MessageException;
import com.github.obase.Page;
import com.github.obase.kit.ClassKit;
import com.github.obase.mysql.JdbcMeta;
import com.github.obase.mysql.MysqlErrno;
import com.github.obase.mysql.asm.AsmKit;
import com.github.obase.mysql.data.ClassMetaInfo;
import com.github.obase.mysql.sql.SqlDdlKit;
import com.github.obase.mysql.sql.SqlMetaKit;
import com.github.obase.mysql.xml.ObaseMysqlObject;
import com.github.obase.mysql.xml.ObaseMysqlParser;
import com.github.obase.mysql.xml.Statement;
public class MysqlClientImpl extends MysqlClientOperation {
static final Log logger = LogFactory.getLog(MysqlClientOperation.class);
// =============================================
// 基础属性及设置
// =============================================
protected String packagesToScan; // multi-value separated by comma ","
protected String configLocations; // multi-value separated by comma ","
boolean updateTable; // update table or not
public void setPackagesToScan(String packagesToScan) {
this.packagesToScan = packagesToScan;
}
public void setConfigLocations(String configLocations) {
this.configLocations = configLocations;
}
public void setUpdateTable(boolean updateTable) {
this.updateTable = updateTable;
}
public Statement getStatement(String key) {
return statementCache.get(key);
}
public SPstmtMeta getSelectAllPstmtMeta(Class> key) {
return selectListCache.get(key);
}
public SPstmtMeta getSelectPstmtMeta(Class> key) {
return selectCache.get(key);
}
public SPstmtMeta getInsertPstmtMeta(Class> key) {
return insertCache.get(key);
}
public SPstmtMeta getInsertIgnorePstmtMeta(Class> key) {
return insertIgnoreCache.get(key);
}
public SPstmtMeta getReplacePstmtMeta(Class> key) {
return replaceCache.get(key);
}
public SPstmtMeta getMergePstmtMeta(Class> key) {
return mergeCache.get(key);
}
public SPstmtMeta getUpdatePstmtMeta(Class> key) {
return updateCache.get(key);
}
public SPstmtMeta getDeletePstmtMeta(Class> key) {
return deleteCache.get(key);
}
// =============================================
// 全局缓存属性, 由metainfo快速生成的缓存.部分如limit,count,
// insertIgnore, replace等合并到对应的SQL中
// =============================================
final Map statementCache = new HashMap(); // xml中statement缓存
final Map, SPstmtMeta> selectListCache = new HashMap, SPstmtMeta>(); // 全表查询,同时缓存limit,count
final Map, SPstmtMeta> selectCache = new HashMap, SPstmtMeta>(); // 记录查询
final Map, SPstmtMeta> insertCache = new HashMap, SPstmtMeta>(); // 插入
final Map, SPstmtMeta> insertIgnoreCache = new HashMap, SPstmtMeta>(); // 插入忽略
final Map, SPstmtMeta> replaceCache = new HashMap, SPstmtMeta>(); // 替换
final Map, SPstmtMeta> mergeCache = new HashMap, SPstmtMeta>(); // 合并非null,采用insert or update语法
final Map, SPstmtMeta> updateCache = new HashMap, SPstmtMeta>(); // 更新
final Map, SPstmtMeta> deleteCache = new HashMap, SPstmtMeta>(); // 删除
// 用于双活方案的快捷方法,从from复制所有cache
public void doInit(MysqlClientImpl from) {
this.statementCache.clear();
this.selectListCache.clear();
this.selectCache.clear();
this.insertCache.clear();
this.insertIgnoreCache.clear();
this.replaceCache.clear();
this.mergeCache.clear();
this.updateCache.clear();
this.deleteCache.clear();
this.statementCache.putAll(from.statementCache);
this.selectListCache.putAll(from.selectListCache);
this.selectCache.putAll(from.selectCache);
this.insertCache.putAll(from.insertCache);
this.insertIgnoreCache.putAll(from.insertIgnoreCache);
this.replaceCache.putAll(from.replaceCache);
this.mergeCache.putAll(from.mergeCache);
this.updateCache.putAll(from.updateCache);
this.deleteCache.putAll(from.deleteCache);
}
protected void doInit(Connection conn) throws Exception {
final Pattern separator = Pattern.compile("\\s*,\\s*");
final PathMatchingResourcePatternResolver resolver = new PathMatchingResourcePatternResolver();
Map metaMetaInfoMap = new HashMap(); // key is classname
Map tableMetaInfoMap = new HashMap(); // key is tablename
ClassMetaInfo classMetaInfo, tableMetaInfo;
StringBuilder sb = new StringBuilder(128);
String key;
if (isNotEmpty(packagesToScan)) {
String[] pkgs = separator.split(packagesToScan);
for (String pkg : pkgs) {
if (isNotEmpty(pkg)) {
sb.setLength(0);
String packageSearchPath = sb.append(ResourcePatternResolver.CLASSPATH_ALL_URL_PREFIX).append(ClassKit.getInternalNameFromClassName(pkg)).append("/**/*.class").toString();
Resource[] rss = resolver.getResources(packageSearchPath);
for (Resource rs : rss) {
classMetaInfo = AsmKit.getAnnotationClassMetaInfo(rs);
if (classMetaInfo.tableAnnotation != null || classMetaInfo.metaAnnotation != null) {
metaMetaInfoMap.put(ClassKit.getClassNameFromInternalName(classMetaInfo.internalName), classMetaInfo);
if (classMetaInfo.tableAnnotation != null) {
if (logger.isInfoEnabled()) {
logger.info(String.format("Load @Table: %s %s", classMetaInfo.tableName, classMetaInfo.columns));
}
if ((tableMetaInfo = tableMetaInfoMap.put(classMetaInfo.tableName, classMetaInfo)) != null) {
throw new MessageException(MysqlErrno.SOURCE, MysqlErrno.META_INFO_DUBLICATE_TABLE, "Duplicate table: " + classMetaInfo.tableName + ", please check class:" + classMetaInfo.internalName + "," + tableMetaInfo.internalName);
}
}
}
}
}
}
}
if (isNotEmpty(configLocations)) {
ObaseMysqlParser parser = new ObaseMysqlParser();
String[] locations = separator.split(configLocations);
for (String location : locations) {
if (isNotEmpty(location)) {
Resource[] rss = resolver.getResources(location);
for (Resource rs : rss) {
ObaseMysqlObject configMetaInfo = parser.parse(rs);
for (Class> clazz : configMetaInfo.tableClassList) {
String className = clazz.getCanonicalName();
if (!metaMetaInfoMap.containsKey(className)) {
classMetaInfo = AsmKit.getAnnotationClassMetaInfo(className);
if (classMetaInfo.tableAnnotation != null || classMetaInfo.metaAnnotation != null) {
metaMetaInfoMap.put(className, classMetaInfo);
if (classMetaInfo.tableAnnotation != null) {
if (logger.isInfoEnabled()) {
logger.info(String.format("Load @Table: %s %s", classMetaInfo.tableName, classMetaInfo.columns));
}
if ((tableMetaInfo = tableMetaInfoMap.put(classMetaInfo.tableName, classMetaInfo)) != null) {
throw new MessageException(MysqlErrno.SOURCE, MysqlErrno.META_INFO_DUBLICATE_TABLE, "Duplicate table: " + classMetaInfo.tableName + ", please check class:" + classMetaInfo.internalName + "," + tableMetaInfo.internalName);
}
}
}
}
}
for (Class> clazz : configMetaInfo.metaClassList) {
String className = clazz.getCanonicalName();
if (!metaMetaInfoMap.containsKey(className)) {
classMetaInfo = AsmKit.getClassMetaInfo(className);
classMetaInfo.tableAnnotation = null; // FIXBUG:的类不是
metaMetaInfoMap.put(className, classMetaInfo);
}
}
for (Statement stmt : configMetaInfo.statementList) {
// key = namespace + . + id
sb.setLength(0);
if (isNotEmpty(configMetaInfo.namespace)) {
// ignore if not setting namespace
sb.append(configMetaInfo.namespace).append('.');
}
key = sb.append(stmt.id).toString();
if (statementCache.put(key, stmt) != null) {
throw new MessageException(MysqlErrno.SOURCE, MysqlErrno.SQL_CONFIG_DUPLICATE, "Duplicate statement id: " + key);
}
}
}
}
}
}
Class> clazz;
for (Map.Entry entry : metaMetaInfoMap.entrySet()) {
clazz = ClassKit.forName(entry.getKey());
classMetaInfo = entry.getValue();
// 初始化JdbcMeta
JdbcMeta.set(clazz, AsmKit.newJdbcMeta(classMetaInfo), false);
// 初始化ORM相关的SQL
if (classMetaInfo.tableAnnotation != null) {
selectListCache.put(clazz, SqlMetaKit.genSelectAllPstmt(classMetaInfo));
selectCache.put(clazz, SqlMetaKit.genSelectPstmt(classMetaInfo));
insertCache.put(clazz, SqlMetaKit.genInsertPstmt(classMetaInfo));
insertIgnoreCache.put(clazz, SqlMetaKit.genInsertIgnorePstmt(classMetaInfo));
replaceCache.put(clazz, SqlMetaKit.genReplacePstmt(classMetaInfo));
mergeCache.put(clazz, SqlMetaKit.genMergePstmt(classMetaInfo));
updateCache.put(clazz, SqlMetaKit.genUpdatePstmt(classMetaInfo));
deleteCache.put(clazz, SqlMetaKit.genDeletePstmt(classMetaInfo));
}
}
if (updateTable) {
if (tableMetaInfoMap.size() > 0) {
SqlDdlKit.processUpdateTable(conn, tableMetaInfoMap);
}
}
if (logger.isInfoEnabled()) {
logger.info(String.format("Mysqlclient initialization successful, load %d tables, %d metas, and %d statements", tableMetaInfoMap.size(), metaMetaInfoMap.size(), statementCache.size()));
}
}
@Override
public List selectList(Class table) throws SQLException {
SPstmtMeta pstmt = selectListCache.get(table);
if (pstmt == null) {
throw new MessageException(MysqlErrno.SOURCE, MysqlErrno.META_INFO_NOT_FOUND, "Not found table: " + table);
}
return query(pstmt, table, null);
}
@Override
public T selectFirst(Class table) throws SQLException {
SPstmtMeta pstmt = selectListCache.get(table);
if (pstmt == null) {
throw new MessageException(MysqlErrno.SOURCE, MysqlErrno.META_INFO_NOT_FOUND, "Not found table: " + table);
}
return queryFirst(pstmt, table, null);
}
@Override
public List selectRange(Class table, int offset, int count) throws SQLException {
SPstmtMeta pstmt = selectListCache.get(table);
if (pstmt == null) {
throw new MessageException(MysqlErrno.SOURCE, MysqlErrno.META_INFO_NOT_FOUND, "Not found table: " + table);
}
return queryRange(pstmt, table, offset, count, null);
}
@Override
public void selectPage(Class table, Page page) throws SQLException {
SPstmtMeta pstmt = selectListCache.get(table);
if (pstmt == null) {
throw new MessageException(MysqlErrno.SOURCE, MysqlErrno.META_INFO_NOT_FOUND, "Not found table: " + table);
}
queryPage(pstmt, table, page, null);
}
@Override
public T select(Class table, Object object) throws SQLException {
SPstmtMeta pstmt = selectCache.get(table);
if (pstmt == null) {
throw new MessageException(MysqlErrno.SOURCE, MysqlErrno.META_INFO_NOT_FOUND, "Not found table: " + table);
}
return queryFirst(pstmt, table, object);
}
@Override
public boolean select2(Class table, Object object) throws SQLException {
SPstmtMeta pstmt = selectCache.get(table);
if (pstmt == null) {
throw new MessageException(MysqlErrno.SOURCE, MysqlErrno.META_INFO_NOT_FOUND, "Not found table: " + table);
}
return queryFirst2(pstmt, object);
}
@Override
public T selectByKey(Class table, Object keys) throws SQLException {
SPstmtMeta pstmt = selectCache.get(table);
if (pstmt == null) {
throw new MessageException(MysqlErrno.SOURCE, MysqlErrno.META_INFO_NOT_FOUND, "Not found table: " + table);
}
return queryFirst(pstmt, table, keys);
}
@Override
public T selectByKeys(Class table, Object... keys) throws SQLException {
SPstmtMeta pstmt = selectCache.get(table);
if (pstmt == null) {
throw new MessageException(MysqlErrno.SOURCE, MysqlErrno.META_INFO_NOT_FOUND, "Not found table: " + table);
}
return queryFirst(pstmt, table, keys);
}
@Override
public int insert(Class> table, Object object) throws SQLException {
SPstmtMeta pstmt = insertCache.get(table);
if (pstmt == null) {
throw new MessageException(MysqlErrno.SOURCE, MysqlErrno.META_INFO_NOT_FOUND, "Not found table: " + table);
}
return executeUpdate(pstmt, object);
}
@Override
public R insert(Class> table, Class generatedKeyType, Object object) throws SQLException {
SPstmtMeta pstmt = insertCache.get(table);
if (pstmt == null) {
throw new MessageException(MysqlErrno.SOURCE, MysqlErrno.META_INFO_NOT_FOUND, "Not found table: " + table);
}
return executeUpdate(pstmt, generatedKeyType, object);
}
@Override
public int insertIgnore(Class> table, Object object) throws SQLException {
SPstmtMeta pstmt = insertIgnoreCache.get(table);
if (pstmt == null) {
throw new MessageException(MysqlErrno.SOURCE, MysqlErrno.META_INFO_NOT_FOUND, "Not found table: " + table);
}
return executeUpdate(pstmt, object);
}
@Override
public R insertIgnore(Class> table, Class generatedKeyType, Object object) throws SQLException {
SPstmtMeta pstmt = insertIgnoreCache.get(table);
if (pstmt == null) {
throw new MessageException(MysqlErrno.SOURCE, MysqlErrno.META_INFO_NOT_FOUND, "Not found table: " + table);
}
return executeUpdate(pstmt, generatedKeyType, object);
}
@Override
public int replace(Class> table, Object object) throws SQLException {
SPstmtMeta pstmt = replaceCache.get(table);
if (pstmt == null) {
throw new MessageException(MysqlErrno.SOURCE, MysqlErrno.META_INFO_NOT_FOUND, "Not found table: " + table);
}
return executeUpdate(pstmt, object);
}
@Override
public R replace(Class> table, Class generatedKeyType, Object object) throws SQLException {
SPstmtMeta pstmt = replaceCache.get(table);
if (pstmt == null) {
throw new MessageException(MysqlErrno.SOURCE, MysqlErrno.META_INFO_NOT_FOUND, "Not found table: " + table);
}
return executeUpdate(pstmt, generatedKeyType, object);
}
@Override
public int merge(Class> table, Object object) throws SQLException {
SPstmtMeta pstmt = mergeCache.get(table);
if (pstmt == null) {
throw new MessageException(MysqlErrno.SOURCE, MysqlErrno.META_INFO_NOT_FOUND, "Not found table: " + table);
}
return executeUpdate(pstmt, object);
}
@Override
public R merge(Class> table, Class generatedKeyType, Object object) throws SQLException {
SPstmtMeta pstmt = mergeCache.get(table);
if (pstmt == null) {
throw new MessageException(MysqlErrno.SOURCE, MysqlErrno.META_INFO_NOT_FOUND, "Not found table: " + table);
}
return executeUpdate(pstmt, generatedKeyType, object);
}
@Override
public int update(Class> table, Object object) throws SQLException {
SPstmtMeta pstmt = updateCache.get(table);
if (pstmt == null) {
throw new MessageException(MysqlErrno.SOURCE, MysqlErrno.META_INFO_NOT_FOUND, "Not found table: " + table);
}
return executeUpdate(pstmt, object);
}
@Override
public int delete(Class> table, Object object) throws SQLException {
SPstmtMeta pstmt = deleteCache.get(table);
if (pstmt == null) {
throw new MessageException(MysqlErrno.SOURCE, MysqlErrno.META_INFO_NOT_FOUND, "Not found table: " + table);
}
return executeUpdate(pstmt, object);
}
@Override
public int deleteByKey(Class> table, Object keys) throws SQLException {
SPstmtMeta pstmt = deleteCache.get(table);
if (pstmt == null) {
throw new MessageException(MysqlErrno.SOURCE, MysqlErrno.META_INFO_NOT_FOUND, "Not found table: " + table);
}
return executeUpdate(pstmt, keys);
}
@Override
public int deleteByKeys(Class> table, Object... keys) throws SQLException {
SPstmtMeta pstmt = deleteCache.get(table);
if (pstmt == null) {
throw new MessageException(MysqlErrno.SOURCE, MysqlErrno.META_INFO_NOT_FOUND, "Not found table: " + table);
}
return executeUpdate(pstmt, keys);
}
@Override
public int[] batchInsert(Class> table, List objects) throws SQLException {
SPstmtMeta pstmt = insertCache.get(table);
if (pstmt == null) {
throw new MessageException(MysqlErrno.SOURCE, MysqlErrno.META_INFO_NOT_FOUND, "Not found table: " + table);
}
return executeBatch(pstmt, objects);
}
@Override
public List batchInsert(Class> table, Class generatedKeyType, List objects) throws SQLException {
SPstmtMeta pstmt = insertCache.get(table);
if (pstmt == null) {
throw new MessageException(MysqlErrno.SOURCE, MysqlErrno.META_INFO_NOT_FOUND, "Not found table: " + table);
}
return executeBatch(pstmt, generatedKeyType, objects);
}
@Override
public int[] batchUpdate(Class> table, List objects) throws SQLException {
SPstmtMeta pstmt = updateCache.get(table);
if (pstmt == null) {
throw new MessageException(MysqlErrno.SOURCE, MysqlErrno.META_INFO_NOT_FOUND, "Not found table: " + table);
}
return executeBatch(pstmt, objects);
}
@Override
public int[] batchInsertIgnore(Class> table, List objects) throws SQLException {
SPstmtMeta pstmt = insertIgnoreCache.get(table);
if (pstmt == null) {
throw new MessageException(MysqlErrno.SOURCE, MysqlErrno.META_INFO_NOT_FOUND, "Not found table: " + table);
}
return executeBatch(pstmt, objects);
}
@Override
public List batchInsertIgnore(Class> table, Class generatedKeyType, List objects) throws SQLException {
SPstmtMeta pstmt = insertIgnoreCache.get(table);
if (pstmt == null) {
throw new MessageException(MysqlErrno.SOURCE, MysqlErrno.META_INFO_NOT_FOUND, "Not found table: " + table);
}
return executeBatch(pstmt, generatedKeyType, objects);
}
@Override
public int[] batchReplace(Class> table, List objects) throws SQLException {
SPstmtMeta pstmt = replaceCache.get(table);
if (pstmt == null) {
throw new MessageException(MysqlErrno.SOURCE, MysqlErrno.META_INFO_NOT_FOUND, "Not found table: " + table);
}
return executeBatch(pstmt, objects);
}
@Override
public List batchReplace(Class> table, Class generatedKeyType, List objects) throws SQLException {
SPstmtMeta pstmt = replaceCache.get(table);
if (pstmt == null) {
throw new MessageException(MysqlErrno.SOURCE, MysqlErrno.META_INFO_NOT_FOUND, "Not found table: " + table);
}
return executeBatch(pstmt, generatedKeyType, objects);
}
@Override
public int[] batchMerge(Class> table, List objects) throws SQLException {
SPstmtMeta pstmt = mergeCache.get(table);
if (pstmt == null) {
throw new MessageException(MysqlErrno.SOURCE, MysqlErrno.META_INFO_NOT_FOUND, "Not found table: " + table);
}
return executeBatch(pstmt, objects);
}
@Override
public List batchMerge(Class> table, Class generatedKeyType, List objects) throws SQLException {
SPstmtMeta pstmt = mergeCache.get(table);
if (pstmt == null) {
throw new MessageException(MysqlErrno.SOURCE, MysqlErrno.META_INFO_NOT_FOUND, "Not found table: " + table);
}
return executeBatch(pstmt, generatedKeyType, objects);
}
@Override
public int[] batchDelete(Class> table, List objects) throws SQLException {
SPstmtMeta pstmt = deleteCache.get(table);
if (pstmt == null) {
throw new MessageException(MysqlErrno.SOURCE, MysqlErrno.META_INFO_NOT_FOUND, "Not found table: " + table);
}
return executeBatch(pstmt, objects);
}
@Override
public int[] batchDeleteByKey(Class> table, List keys) throws SQLException {
SPstmtMeta pstmt = deleteCache.get(table);
if (pstmt == null) {
throw new MessageException(MysqlErrno.SOURCE, MysqlErrno.META_INFO_NOT_FOUND, "Not found table: " + table);
}
return executeBatch(pstmt, keys);
}
@Override
public List query(String queryId, Class elemType, Object params) throws SQLException {
Statement xstmt = statementCache.get(queryId);
if (xstmt == null) {
throw new MessageException(MysqlErrno.SOURCE, MysqlErrno.META_INFO_NOT_FOUND, "Not found statement: " + queryId);
}
return query(xstmt.staticPstmtMeta != null ? xstmt.staticPstmtMeta : xstmt.dynamicPstmtMeta(JdbcMeta.getByObj(params), params), elemType, params);
}
@Override
public List queryRange(String queryId, Class elemType, int offset, int count, Object params) throws SQLException {
Statement xstmt = statementCache.get(queryId);
if (xstmt == null) {
throw new MessageException(MysqlErrno.SOURCE, MysqlErrno.META_INFO_NOT_FOUND, "Not found statement: " + queryId);
}
return queryRange(xstmt.staticPstmtMeta != null ? xstmt.staticPstmtMeta : xstmt.dynamicPstmtMeta(JdbcMeta.getByObj(params), params), elemType, offset, count, params);
}
@Override
public T queryFirst(String queryId, Class elemType, Object params) throws SQLException {
Statement xstmt = statementCache.get(queryId);
if (xstmt == null) {
throw new MessageException(MysqlErrno.SOURCE, MysqlErrno.META_INFO_NOT_FOUND, "Not found statement: " + queryId);
}
return queryFirst(xstmt.staticPstmtMeta != null ? xstmt.staticPstmtMeta : xstmt.dynamicPstmtMeta(JdbcMeta.getByObj(params), params), elemType, params);
}
@Override
public boolean queryFirst2(String queryId, Object params) throws SQLException {
Statement xstmt = statementCache.get(queryId);
if (xstmt == null) {
throw new MessageException(MysqlErrno.SOURCE, MysqlErrno.META_INFO_NOT_FOUND, "Not found statement: " + queryId);
}
return queryFirst2(xstmt.staticPstmtMeta != null ? xstmt.staticPstmtMeta : xstmt.dynamicPstmtMeta(JdbcMeta.getByObj(params), params), params);
}
@Override
public void queryPage(String queryId, Class elemType, Page page, Object params) throws SQLException {
Statement xstmt = statementCache.get(queryId);
if (xstmt == null) {
throw new MessageException(MysqlErrno.SOURCE, MysqlErrno.META_INFO_NOT_FOUND, "Not found statement: " + queryId);
}
queryPage(xstmt.staticPstmtMeta != null ? xstmt.staticPstmtMeta : xstmt.dynamicPstmtMeta(JdbcMeta.getByObj(params), params), elemType, page, params);
}
@Override
public int execute(String updateId, Object params) throws SQLException {
Statement xstmt = statementCache.get(updateId);
if (xstmt == null) {
throw new MessageException(MysqlErrno.SOURCE, MysqlErrno.META_INFO_NOT_FOUND, "Not found statement: " + updateId);
}
return executeUpdate(xstmt.staticPstmtMeta != null ? xstmt.staticPstmtMeta : xstmt.dynamicPstmtMeta(JdbcMeta.getByObj(params), params), params);
}
@Override
public R execute(String updateId, Class generateKeyType, Object params) throws SQLException {
Statement xstmt = statementCache.get(updateId);
if (xstmt == null) {
throw new MessageException(MysqlErrno.SOURCE, MysqlErrno.META_INFO_NOT_FOUND, "Not found statement: " + updateId);
}
return executeUpdate(xstmt.staticPstmtMeta != null ? xstmt.staticPstmtMeta : xstmt.dynamicPstmtMeta(JdbcMeta.getByObj(params), params), generateKeyType, params);
}
@Override
public int[] batchExecute(String updateId, List params) throws SQLException {
Statement xstmt = statementCache.get(updateId);
if (xstmt == null) {
throw new MessageException(MysqlErrno.SOURCE, MysqlErrno.META_INFO_NOT_FOUND, "Not found statement: " + updateId);
}
if (xstmt.staticPstmtMeta != null) {
return executeBatch(xstmt.staticPstmtMeta != null ? xstmt.staticPstmtMeta : xstmt.dynamicPstmtMeta(JdbcMeta.getByObj(params), params), params);
}
throw new MessageException(MysqlErrno.SOURCE, MysqlErrno.SQL_DYNAMIC_NOT_SUPPORT, "executeBatch don't support dynamic statement: " + xstmt.id);
}
@Override
public List batchExecute(String updateId, Class generateKeyType, List params) throws SQLException {
Statement xstmt = statementCache.get(updateId);
if (xstmt == null) {
throw new MessageException(MysqlErrno.SOURCE, MysqlErrno.META_INFO_NOT_FOUND, "Not found statement: " + updateId);
}
if (xstmt.staticPstmtMeta != null) {
return executeBatch(xstmt.staticPstmtMeta, generateKeyType, params);
}
throw new MessageException(MysqlErrno.SOURCE, MysqlErrno.SQL_DYNAMIC_NOT_SUPPORT, "executeBatch don't support dynamic statement: " + xstmt.id);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy