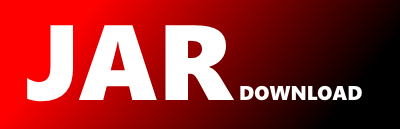
com.github.os72.protocjar.ProtocProxy.proxy Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of protoc-jar Show documentation
Show all versions of protoc-jar Show documentation
Protocol Buffers compiler - executable JAR and API
package com.github.os72.protocjar;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import java.io.UnsupportedEncodingException;
import java.net.URLEncoder;
import java.nio.file.Files;
import java.nio.file.StandardCopyOption;
import java.util.ArrayList;
import java.util.List;
import net.lingala.zip4j.core.ZipFile;
import net.lingala.zip4j.exception.ZipException;
import net.lingala.zip4j.model.ZipParameters;
import org.apache.commons.io.FileUtils;
import org.apache.http.client.methods.CloseableHttpResponse;
import org.apache.http.client.methods.HttpPost;
import org.apache.http.entity.ContentType;
import org.apache.http.entity.FileEntity;
import org.apache.http.impl.client.CloseableHttpClient;
import org.apache.http.impl.client.HttpClients;
public class ProtocProxy
{
public static int runRemote(List argList, OutputStream out, OutputStream err) throws IOException, InterruptedException {
File tmp = File.createTempFile("protocjar", "");
tmp.delete(); tmp.mkdirs();
String tempRoot = tmp.getAbsolutePath() + "/";
List inDirList = new ArrayList();
List protocCmd = new ArrayList();
for (String arg : argList) {
if (arg.contains("_out=")) {
String[] argSplit = arg.split("_out=");
File outDir = new File(argSplit[1]);
outDir.mkdirs();
protocCmd.add(argSplit[0] + "_out=" + getNormAbsolutePath(outDir));
}
else if (arg.startsWith("-I")) {
File inDir = new File(arg.substring(2));
inDirList.add(inDir.getAbsolutePath());
String inDirTemp = tempRoot + normalizePath(inDir.getAbsolutePath());
new File(inDirTemp).mkdirs();
for (File file : inDir.listFiles()) {
Files.copy(file.toPath(), new File(inDirTemp + "/" + file.getName()).toPath(), StandardCopyOption.REPLACE_EXISTING);
}
protocCmd.add("-I" + inDirTemp);
}
else if (!arg.startsWith("-")) {
File inFile = new File(arg);
protocCmd.add(getNormAbsolutePath(inFile));
}
else {
protocCmd.add(arg);
}
}
log("*** inDirList: " + inDirList);
log("*** " + protocCmd);
return 0;
}
public static int runRemote1(List argList, OutputStream out, OutputStream err) throws IOException, InterruptedException {
String inDir = "src/test/resources";
String inFile = "src/test/resources/Schema1.proto";
String outDir = "target/test-protoc";
String inDirAbsolute = new File(inDir).getAbsolutePath();
String inFileAbsolute = new File(inFile).getAbsolutePath();
String outDirAbsolute = new File(outDir).getAbsolutePath();
System.out.println(inDirAbsolute);
System.out.println(inFileAbsolute);
System.out.println(outDirAbsolute);
File tmp = File.createTempFile("protocjar", "");
tmp.delete();
tmp.mkdirs();
String tempRoot = tmp.getAbsolutePath() + "/";
String inDirTemp = tempRoot + normalizePath(inDirAbsolute);
String inFileTemp = tempRoot + normalizePath(inFileAbsolute);
String outDirTemp = tempRoot + normalizePath(outDirAbsolute);
log(inDirTemp);
log(inFileTemp);
log(outDirTemp);
File zipFile = null;
try {
new File(outDir).mkdirs();
String[] args = {"-v2.4.1", "-I"+inDir, "--java_out="+outDir, inFile};
//Protoc.runProtoc(args);
new File(inDirTemp).mkdirs();
new File(outDirTemp).mkdirs();
Files.copy(new File(inFileAbsolute).toPath(), new File(inFileTemp).toPath(), StandardCopyOption.REPLACE_EXISTING);
for (File file : new File(inDirAbsolute).listFiles()) {
Files.copy(file.toPath(), new File(inDirTemp + "/" + file.getName()).toPath(), StandardCopyOption.REPLACE_EXISTING);
}
String[] args1 = {"-v2.4.1", "-I"+inDirTemp, "--java_out="+outDirTemp, inFileTemp};
//Protoc.runProtoc(args1);
ZipFile zip = new ZipFile(tmp.getAbsolutePath() + ".req.zip");
zip.addFolder(tempRoot, new ZipParameters());
zipFile = zip.getFile();
}
catch (Exception e) {
e.printStackTrace();
}
CloseableHttpClient httpclient = HttpClients.createDefault();
try {
//HttpPost httppost = new HttpPost("http://httpbin.org/post");
HttpPost httppost = new HttpPost("http://localhost:8080/protoc" +
"?root=" + normalizePathForURL(tmp.getName()) +
"&inDir=" + normalizePathForURL(inDirAbsolute) +
"&inFile=" + normalizePathForURL(inFileAbsolute) +
"&outDir=" + normalizePathForURL(outDirAbsolute)
);
File file = new File(inFile);
file = zipFile;
FileEntity reqEntity = new FileEntity(file, ContentType.APPLICATION_OCTET_STREAM);
//InputStreamEntity reqEntity = new InputStreamEntity(new FileInputStream(file), -1, ContentType.APPLICATION_OCTET_STREAM);
reqEntity.setChunked(true);
httppost.setEntity(reqEntity);
System.out.println("Executing request: " + httppost.getRequestLine());
CloseableHttpResponse response = httpclient.execute(httppost);
try {
System.out.println("----------------------------------------");
System.out.println(response.getStatusLine());
//System.out.println(EntityUtils.toString(response.getEntity()));
InputStream respInStream = response.getEntity().getContent();
FileOutputStream fileOutStream = new FileOutputStream(tmp.getAbsolutePath() + ".resp.zip");
int len;
byte[] buf = new byte[4096];
while ((len = respInStream.read(buf)) > 0) fileOutStream.write(buf, 0, len);
fileOutStream.close();
respInStream.close();
}
finally {
response.close();
}
}
finally {
httpclient.close();
}
try {
ZipFile zip = new ZipFile(tmp.getAbsolutePath() + ".resp.zip");
zip.extractAll(tmp.getParentFile().getAbsolutePath());
}
catch (ZipException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
FileUtils.copyDirectory(new File(outDirTemp), new File(outDir));
FileInputStream protocInStream = new FileInputStream(tempRoot + "/protoc.log");
Protoc.streamCopy(protocInStream, System.out);
protocInStream.close();
return 0;
}
static String getNormAbsolutePath(File f) {
return normalizePath(f.getAbsolutePath());
}
static String normalizePathForURL(String path) throws UnsupportedEncodingException {
return URLEncoder.encode(normalizePath(path), "UTF-8");
}
static String normalizePath(String path) {
return path.replace(":", "").replace("\\", "/");
}
static void log(Object msg) {
Protoc.log(msg);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy