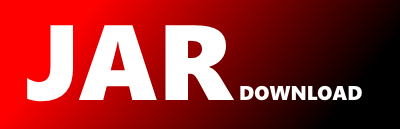
com.aerospike.client.async.AsyncMultiCommand Maven / Gradle / Ivy
/*******************************************************************************
* Copyright 2012-2014 by Aerospike.
*
* Permission is hereby granted, free of charge, to any person obtaining a copy
* of this software and associated documentation files (the "Software"), to
* deal in the Software without restriction, including without limitation the
* rights to use, copy, modify, merge, publish, distribute, sublicense, and/or
* sell copies of the Software, and to permit persons to whom the Software is
* furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included in
* all copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING
* FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS
* IN THE SOFTWARE.
******************************************************************************/
package com.aerospike.client.async;
import java.io.IOException;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.HashSet;
import java.util.Map;
import com.aerospike.client.AerospikeException;
import com.aerospike.client.Key;
import com.aerospike.client.Record;
import com.aerospike.client.ResultCode;
import com.aerospike.client.command.Buffer;
import com.aerospike.client.command.Command;
import com.aerospike.client.command.FieldType;
public abstract class AsyncMultiCommand extends AsyncCommand {
private final AsyncMultiExecutor parent;
private final AsyncNode node;
protected final HashSet binNames;
protected byte[] receiveBuffer;
protected int receiveSize;
protected int receiveOffset;
protected int resultCode;
protected int generation;
protected int expiration;
protected int fieldCount;
protected int opCount;
private final boolean stopOnNotFound;
public AsyncMultiCommand(AsyncMultiExecutor parent, AsyncCluster cluster, AsyncNode node, boolean stopOnNotFound) {
super(cluster);
this.parent = parent;
this.node = node;
this.stopOnNotFound = stopOnNotFound;
this.binNames = null;
}
public AsyncMultiCommand(AsyncMultiExecutor parent, AsyncCluster cluster, AsyncNode node, boolean stopOnNotFound, HashSet binNames) {
super(cluster);
this.parent = parent;
this.node = node;
this.stopOnNotFound = stopOnNotFound;
this.binNames = binNames;
}
protected final AsyncNode getNode() {
return node;
}
protected final void read() throws AerospikeException, IOException {
while (true) {
if (inHeader) {
if (! conn.read(byteBuffer)) {
return;
}
byteBuffer.position(0);
receiveSize = ((int) (byteBuffer.getLong() & 0xFFFFFFFFFFFFL));
if (receiveSize <= 0) {
finish();
return;
}
if (receiveBuffer == null || receiveSize > receiveBuffer.length) {
receiveBuffer = new byte[receiveSize];
}
byteBuffer.clear();
if (receiveSize < byteBuffer.capacity()) {
byteBuffer.limit(receiveSize);
}
inHeader = false;
}
if (! conn.read(byteBuffer)) {
return;
}
// Copy byteBuffer to byte[].
byteBuffer.position(0);
byteBuffer.get(receiveBuffer, receiveOffset, byteBuffer.limit());
receiveOffset += byteBuffer.limit();
byteBuffer.clear();
if (receiveOffset >= receiveSize) {
if (parseGroup()) {
finish();
return;
}
// Prepare for next group.
byteBuffer.limit(8);
receiveOffset = 0;
inHeader = true;
}
else {
int remaining = receiveSize - receiveOffset;
if (remaining < byteBuffer.capacity()) {
byteBuffer.limit(remaining);
}
}
}
}
private final boolean parseGroup() throws AerospikeException {
// Parse each message response and add it to the result array
receiveOffset = 0;
while (receiveOffset < receiveSize) {
resultCode = receiveBuffer[receiveOffset + 5] & 0xFF;
if (resultCode != 0) {
if (resultCode == ResultCode.KEY_NOT_FOUND_ERROR) {
if (stopOnNotFound) {
return true;
}
}
else {
throw new AerospikeException(resultCode);
}
}
// If this is the end marker of the response, do not proceed further
if ((receiveBuffer[receiveOffset + 3] & Command.INFO3_LAST) != 0) {
return true;
}
generation = Buffer.bytesToInt(receiveBuffer, receiveOffset + 6);
expiration = Buffer.bytesToInt(receiveBuffer, receiveOffset + 10);
fieldCount = Buffer.bytesToShort(receiveBuffer, receiveOffset + 18);
opCount = Buffer.bytesToShort(receiveBuffer, receiveOffset + 20);
receiveOffset += Command.MSG_REMAINING_HEADER_SIZE;
Key key = parseKey();
parseRow(key);
}
return false;
}
protected final Key parseKey() {
byte[] digest = null;
String namespace = null;
String setName = null;
for (int i = 0; i < fieldCount; i++) {
int fieldlen = Buffer.bytesToInt(receiveBuffer, receiveOffset);
receiveOffset += 4;
int fieldtype = receiveBuffer[receiveOffset++];
int size = fieldlen - 1;
if (fieldtype == FieldType.DIGEST_RIPE) {
digest = new byte[size];
System.arraycopy(receiveBuffer, receiveOffset, digest, 0, size);
receiveOffset += size;
}
else if (fieldtype == FieldType.NAMESPACE) {
namespace = new String(receiveBuffer, receiveOffset, size);
receiveOffset += size;
}
else if (fieldtype == FieldType.TABLE) {
setName = new String(receiveBuffer, receiveOffset, size);
receiveOffset += size;
}
}
return new Key(namespace, digest, setName);
}
protected Record parseRecordWithDuplicates() throws AerospikeException {
Map bins = null;
ArrayList
© 2015 - 2025 Weber Informatics LLC | Privacy Policy