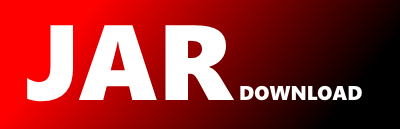
indi.atlantis.framework.chaconne.JobQueryDao Maven / Gradle / Ivy
/**
* Copyright 2017-2021 Fred Feng ([email protected])
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package indi.atlantis.framework.chaconne;
import java.util.Date;
import java.util.List;
import java.util.Map;
import com.github.paganini2008.devtools.jdbc.ResultSetSlice;
import com.github.paganini2008.springdesert.fastjdbc.annotations.Arg;
import com.github.paganini2008.springdesert.fastjdbc.annotations.Dao;
import com.github.paganini2008.springdesert.fastjdbc.annotations.Example;
import com.github.paganini2008.springdesert.fastjdbc.annotations.Get;
import com.github.paganini2008.springdesert.fastjdbc.annotations.Query;
import com.github.paganini2008.springdesert.fastjdbc.annotations.Select;
import com.github.paganini2008.springdesert.fastjdbc.annotations.Sql;
/**
*
* JobQueryDao
*
* @author Fred Feng
*
* @since 2.0.1
*/
@Dao
public interface JobQueryDao {
public static final String DEF_SELECT_CLUSTER_NAME = "select distinct cluster_name from chac_job_server_detail";
public static final String DEF_SELECT_JOB_SERVER_DETAIL = "select * from chac_job_server_detail where cluster_name=:clusterName @sql";
public static final String DEF_SELECT_JOB_SERVER_EXISTS = "select count(*) from chac_job_server_detail where cluster_name=:clusterName and group_name=:groupName and context_path=:contextPath";
public static final String DEF_SELECT_CONTEXT_PATH = "select distinct context_path from chac_job_server_detail where cluster_name=:clusterName order by start_date asc";
public static final String DEF_SELECT_ALL_JOB_DETAIL = "select * from chac_job_detail";
public static final String DEF_SELECT_JOB_DETAIL_BY_CLUSTER_NAMES = "select * from chac_job_detail where cluster_name in (:clusterNames)";
public static final String DEF_SELECT_JOB_DETAIL_BY_GROUP_NAMES = "select * from chac_job_detail where group_name in (:groupNames)";
public static final String DEF_SELECT_AVAILABLE_JOB_DETAIL = "select a.* from chac_job_detail a join chac_job_runtime_detail b on a.job_id=b.job_id where b.job_state<4";
public static final String DEF_SELECT_JOB_ID = "select job_id from chac_job_detail where cluster_name=:clusterName and group_name=:groupName and job_name=:jobName and job_class_name=:jobClassName";
public static final String DEF_SELECT_JOB_TRIGGER_DEADLINE = "select c.cluster_name,c.group_name,c.job_name,c.job_class_name,a.* from chac_job_trigger_detail a join chac_job_runtime_detail b on a.job_id=b.job_id join chac_job_detail c on c.job_id=b.job_id where a.end_date is not null and b.job_state<4";
public static final String DEF_SELECT_JOB_KEYS_BY_TRIGGER_TYPE = "select * from chac_job_detail a where 1=1 @sql and exists (select job_id from chac_job_trigger_detail where job_id=a.job_id and trigger_type=:triggerType)";
public static final String DEF_SELECT_JOB_DETAIL_BY_GROUP_NAME = "select a.* from chac_job_detail a join chac_job_runtime_detail b on a.job_id=b.job_id where a.cluster_name=:clusterName and a.group_name=:groupName and b.job_state<4";
public static final String DEF_SELECT_JOB_DETAIL_BY_OTHER_GROUP_NAME = "select a.* from chac_job_detail a join chac_job_runtime_detail b on a.job_id=b.job_id where a.cluster_name=:clusterName and a.group_name!=:groupName and b.job_state<4";
public static final String DEF_SELECT_JOB_EXISTS = "select count(*) from chac_job_detail where cluster_name=:clusterName and group_name=:groupName and job_name=:jobName and job_class_name=:jobClassName";
public static final String DEF_SELECT_JOB_TRIGGER = "select * from chac_job_trigger_detail where job_id=:jobId";
public static final String DEF_SELECT_LATEST_EXECUTION_TIME = "select min(next_execution_time) from chac_job_runtime_detail where next_execution_time is not null and job_id in (:jobIds)";
public static final String DEF_SELECT_JOB_RUNTIME = "select * from chac_job_runtime_detail where job_id=:jobId";
public static final String DEF_SELECT_JOB_RUNTIME_BY_JOB_STATE = "select a.*,b.* from chac_job_detail a join chac_job_runtime_detail b on a.job_id=b.job_id where 1=1 @sql and b.job_state=:jobState";
public static final String DEF_SELECT_DEPENDENT_JOB_DETAIL = "select * from chac_job_detail where job_id in (select dependent_job_id from chac_job_dependency where job_id=:jobId and dependency_type=:dependencyType)";
public static final String DEF_SELECT_JOB_HAS_RELATIONS = "select count(*) from chac_job_dependency where dependent_job_id=:dependentJobId and dependency_type=:dependencyType";
public static final String DEF_SELECT_JOB_RELATIONS = "select * from chac_job_detail where job_id in (select job_id from chac_job_dependency where dependent_job_id=:dependentJobId and dependency_type=:dependencyType)";
public static final String DEF_SELECT_JOB_TRACE = "select * from chac_job_trace where job_id=:jobId and execution_time between :startDate and :endDate";
public static final String DEF_SELECT_JOB_RUNNING_COUNT = "select count(1) from chac_job_trace where job_id=:jobId and running_state=1";
public static final String DEF_SELECT_JOB_INFO = "select a.*,b.job_state,b.last_running_state,b.last_execution_time,b.last_completion_time,b.next_execution_time,c.trigger_type,c.trigger_description,c.start_date,c.end_date from chac_job_detail a join chac_job_runtime_detail b on b.job_id=a.job_id join chac_job_trigger_detail c on c.job_id=b.job_id where a.cluster_name=:clusterName order by create_date desc";
public static final String DEF_SELECT_JOB_DETAIL = "select * from chac_job_detail where cluster_name=:clusterName and group_name=:groupName and job_name=:jobName and job_class_name=:jobClassName limit 1";
public static final String DEF_SELECT_JOB_LOG = "select * from chac_job_log where job_id=:jobId and trace_id=:traceId";
public static final String DEF_SELECT_JOB_EXCEPTION = "select * from chac_job_exception where job_id=:jobId and trace_id=:traceId";
public static final String DEF_SELECT_JOB_STAT_BY_DAY = "select date_format(execution_time,'%M %d,%Y') as executionDate, cluster_name as clusterName, group_name as groupName, sum(completed) as completedCount, sum(failed) as failedCount, sum(skipped) as skippedCount, sum(finished) as finishedCount, sum(retries) as retryCount from chac_job_trace where 1=1 @sql group by 1,2,3 limit :days";
public static final String DEF_SELECT_JOB_STAT_BY_MONTH = "select date_format(execution_time,'%M,%Y') as executionDate, cluster_name as clusterName, group_name as groupName, sum(completed) as completedCount, sum(failed) as failedCount, sum(skipped) as skippedCount, sum(finished) as finishedCount, sum(retries) as retryCount from chac_job_trace where 1=1 @sql group by 1,2,3 limit 3";
public static final String DEF_SELECT_JOB_STAT_BY_ID = "select a.cluster_name as clusterName, a.group_name as groupName, b.job_name as jobName, b.job_class_name as jobClassName, a.job_id as jobId, date_format(max(a.execution_time),'%M %d,%Y %H:%i:%s') as executionDate, sum(a.completed) as completedCount, sum(a.failed) as failedCount, SUM(a.skipped) AS skippedCount, sum(a.finished) as finishedCount, sum(a.retries) as retryCount from chac_job_trace a join chac_job_detail b on a.job_id=b.job_id where 1=1 @sql group by 1,2,3,4 order by b.create_date desc";
public static final String DEF_SELECT_JOB_STATE_COUNT = "select a.job_state as jobState, count(a.job_state) as jobCount from chac_job_runtime_detail a join chac_job_detail b on a.job_id=b.job_id where b.cluster_name=:clusterName group by a.job_state";
public static final String DEF_SELECT_JOB_STAT = "select sum(completed) as completedCount, sum(failed) as failedCount, sum(skipped) as skippedCount, sum(finished) as finishedCount, sum(retries) as retryCount, max(execution_time) as executionDate from chac_job_trace where 1=1 @sql";
@Query(value = DEF_SELECT_CLUSTER_NAME, singleColumn = true)
List selectClusterNames();
@Query(DEF_SELECT_JOB_SERVER_DETAIL)
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy