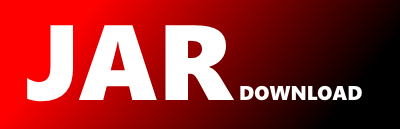
com.github.paganini2008.devtools.db4j.ParsedSqlRunner Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of devtools-db4j Show documentation
Show all versions of devtools-db4j Show documentation
a concise jdbc tools for quick developing
/**
* Copyright 2021 Fred Feng ([email protected])
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.github.paganini2008.devtools.db4j;
import java.sql.Connection;
import java.sql.SQLException;
import java.util.ArrayList;
import java.util.List;
import com.github.paganini2008.devtools.collection.Tuple;
import com.github.paganini2008.devtools.db4j.mapper.ColumnIndexRowMapper;
import com.github.paganini2008.devtools.db4j.mapper.RowMapper;
import com.github.paganini2008.devtools.db4j.mapper.TupleRowMapper;
import com.github.paganini2008.devtools.jdbc.ConnectionFactory;
import com.github.paganini2008.devtools.jdbc.Cursor;
import com.github.paganini2008.devtools.jdbc.DefaultPageableSql;
import com.github.paganini2008.devtools.jdbc.JdbcUtils;
import com.github.paganini2008.devtools.jdbc.PageableException;
import com.github.paganini2008.devtools.jdbc.PageableQuery;
import com.github.paganini2008.devtools.jdbc.PageableResultSetSlice;
import com.github.paganini2008.devtools.jdbc.PageableSql;
/**
*
* ParsedSqlRunner
*
* @author Fred Feng
* @version 1.0
*/
public class ParsedSqlRunner {
private final SqlRunner sqlRunner;
public ParsedSqlRunner() {
this.sqlRunner = new SqlRunner();
}
public T query(Connection connection, String sql, Object[] args, ResultSetExtractor extractor) throws SQLException {
return query(connection, sql, new ArraySqlParameter(args), extractor);
}
public T query(Connection connection, String sql, SqlParameter sqlParameter, ResultSetExtractor extractor) throws SQLException {
ParsedSql parsedSql = getParsedSql(sql);
String rawSql = parsedSql.toString();
Object[] parameters = sqlParameter != null ? getArguments(parsedSql, sqlParameter) : null;
JdbcType[] jdbcTypes = sqlParameter != null ? getJdbcTypes(parsedSql, sqlParameter) : null;
return sqlRunner.query(connection, rawSql, parameters, jdbcTypes, extractor);
}
public List queryForList(Connection connection, String sql, Object[] args) throws SQLException {
return queryForList(connection, sql, new ArraySqlParameter(args));
}
public List queryForList(Connection connection, String sql, SqlParameter sqlParameter) throws SQLException {
ParsedSql parsedSql = getParsedSql(sql);
String rawSql = parsedSql.toString();
Object[] parameters = sqlParameter != null ? getArguments(parsedSql, sqlParameter) : null;
JdbcType[] jdbcTypes = sqlParameter != null ? getJdbcTypes(parsedSql, sqlParameter) : null;
return sqlRunner.queryForList(connection, rawSql, parameters, jdbcTypes);
}
public Cursor queryForCursor(Connection connection, String sql, Object[] args) throws SQLException {
return queryForCursor(connection, sql, new ArraySqlParameter(args));
}
public Cursor queryForCursor(Connection connection, String sql, SqlParameter sqlParameter) throws SQLException {
ParsedSql parsedSql = getParsedSql(sql);
String rawSql = parsedSql.toString();
Object[] parameters = sqlParameter != null ? getArguments(parsedSql, sqlParameter) : null;
JdbcType[] jdbcTypes = sqlParameter != null ? getJdbcTypes(parsedSql, sqlParameter) : null;
return sqlRunner.queryForCursor(connection, rawSql, parameters, jdbcTypes);
}
public Cursor queryForCursor(Connection connection, String sql) throws SQLException {
ParsedSql parsedSql = getParsedSql(sql);
String rawSql = parsedSql.toString();
return sqlRunner.queryForCursor(connection, rawSql);
}
public List queryForList(Connection connection, String sql, SqlParameter sqlParameter, RowMapper rowMapper)
throws SQLException {
ParsedSql parsedSql = getParsedSql(sql);
String rawSql = parsedSql.toString();
Object[] parameters = sqlParameter != null ? getArguments(parsedSql, sqlParameter) : null;
JdbcType[] jdbcTypes = sqlParameter != null ? getJdbcTypes(parsedSql, sqlParameter) : null;
return sqlRunner.queryForList(connection, rawSql, parameters, jdbcTypes, rowMapper);
}
public Cursor queryForCursor(Connection connection, String sql, SqlParameter sqlParameter, RowMapper rowMapper)
throws SQLException {
ParsedSql parsedSql = getParsedSql(sql);
String rawSql = parsedSql.toString();
Object[] parameters = sqlParameter != null ? getArguments(parsedSql, sqlParameter) : null;
JdbcType[] jdbcTypes = sqlParameter != null ? getJdbcTypes(parsedSql, sqlParameter) : null;
return sqlRunner.queryForCursor(connection, rawSql, parameters, jdbcTypes, rowMapper);
}
public Cursor queryForCachedCursor(Connection connection, String sql, Object[] args, RowMapper rowMapper)
throws SQLException {
return queryForCachedCursor(connection, sql, new ArraySqlParameter(args), rowMapper);
}
public Cursor queryForCachedCursor(Connection connection, String sql, SqlParameter sqlParameter, RowMapper rowMapper)
throws SQLException {
ParsedSql parsedSql = getParsedSql(sql);
String rawSql = parsedSql.toString();
Object[] parameters = sqlParameter != null ? getArguments(parsedSql, sqlParameter) : null;
JdbcType[] jdbcTypes = sqlParameter != null ? getJdbcTypes(parsedSql, sqlParameter) : null;
return sqlRunner.queryForCachedCursor(connection, rawSql, parameters, jdbcTypes, rowMapper);
}
public Cursor queryForCachedCursor(Connection connection, String sql, Object[] args) throws SQLException {
return queryForCachedCursor(connection, sql, new ArraySqlParameter(args));
}
public Cursor queryForCachedCursor(Connection connection, String sql, SqlParameter sqlParameter) throws SQLException {
ParsedSql parsedSql = getParsedSql(sql);
String rawSql = parsedSql.toString();
Object[] parameters = sqlParameter != null ? getArguments(parsedSql, sqlParameter) : null;
JdbcType[] jdbcTypes = sqlParameter != null ? getJdbcTypes(parsedSql, sqlParameter) : null;
return sqlRunner.queryForCachedCursor(connection, rawSql, parameters, jdbcTypes);
}
public Cursor queryForCachedCursor(Connection connection, String sql) throws SQLException {
ParsedSql parsedSql = getParsedSql(sql);
String rawSql = parsedSql.toString();
return sqlRunner.queryForCachedCursor(connection, rawSql);
}
public PageableQuery queryForPage(ConnectionFactory connectionFactory, String sql, SqlParameter sqlParameter,
RowMapper rowMapper) {
return new PageableQueryImpl(connectionFactory, new DefaultPageableSql(sql), sqlParameter, rowMapper, this);
}
public PageableQuery queryForPage(ConnectionFactory connectionFactory, PageableSql pageableSql, SqlParameter sqlParameter,
RowMapper rowMapper) {
return new PageableQueryImpl(connectionFactory, pageableSql, sqlParameter, rowMapper, this);
}
public PageableQuery queryForPage(ConnectionFactory connectionFactory, String sql, SqlParameter sqlParameter) {
return queryForPage(connectionFactory, new DefaultPageableSql(sql), sqlParameter);
}
public PageableQuery queryForPage(ConnectionFactory connectionFactory, PageableSql pageableSql, SqlParameter sqlParameter) {
return queryForPage(connectionFactory, pageableSql, sqlParameter, new TupleRowMapper());
}
public T queryForObject(Connection connection, String sql, SqlParameter sqlParameter, Class requiredType) throws SQLException {
return queryForObject(connection, sql, sqlParameter, new ColumnIndexRowMapper(requiredType));
}
public T queryForObject(Connection connection, String sql, SqlParameter sqlParameter, RowMapper rowMapper) throws SQLException {
ParsedSql parsedSql = getParsedSql(sql);
String rawSql = parsedSql.toString();
Object[] parameters = sqlParameter != null ? getArguments(parsedSql, sqlParameter) : null;
JdbcType[] jdbcTypes = sqlParameter != null ? getJdbcTypes(parsedSql, sqlParameter) : null;
return sqlRunner.queryForObject(connection, rawSql, parameters, jdbcTypes, rowMapper);
}
public int[] batchUpdate(Connection connection, String sql, SqlParameters sqlParameters) throws SQLException {
ParsedSql parsedSql = getParsedSql(sql);
String rawSql = parsedSql.toString();
List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy