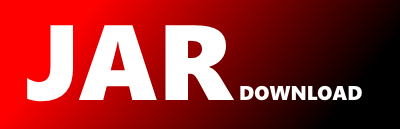
panda.net.echo.EchoUDPClient Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of panda-nets Show documentation
Show all versions of panda-nets Show documentation
Panda Nets is a client side library of many basic Internet protocols.
package panda.net.echo;
import java.io.IOException;
import java.net.DatagramPacket;
import java.net.InetAddress;
import panda.net.discard.DiscardUDPClient;
/***
* The EchoUDPClient class is a UDP implementation of a client for the Echo protocol described in
* RFC 862. To use the class, just open a local UDP port with
* {@link panda.net.DatagramSocketClient#open open } and call {@link #send send } to send datagrams
* to the server, then call {@link #receive receive } to receive echoes. After you're done echoing
* data, call {@link panda.net.DatagramSocketClient#close close() } to clean up properly.
*
* @see EchoTCPClient
* @see DiscardUDPClient
***/
public final class EchoUDPClient extends DiscardUDPClient {
/*** The default echo port. It is set to 7 according to RFC 862. ***/
public static final int DEFAULT_PORT = 7;
private final DatagramPacket __receivePacket = new DatagramPacket(new byte[0], 0);
/***
* Sends the specified data to the specified server at the default echo port.
*
* @param data The echo data to send.
* @param length The length of the data to send. Should be less than or equal to the length of
* the data byte array.
* @param host The address of the server.
* @exception IOException If an error occurs during the datagram send operation.
***/
@Override
public void send(byte[] data, int length, InetAddress host) throws IOException {
send(data, length, host, DEFAULT_PORT);
}
/*** Same as send(data, data.length, host)
***/
@Override
public void send(byte[] data, InetAddress host) throws IOException {
send(data, data.length, host, DEFAULT_PORT);
}
/***
* Receives echoed data and returns its length. The data may be divided up among multiple
* datagrams, requiring multiple calls to receive. Also, the UDP packets will not necessarily
* arrive in the same order they were sent.
*
* @param data the buffer to receive the input
* @param length of the buffer
* @return Length of actual data received.
* @exception IOException If an error occurs while receiving the data.
***/
public int receive(byte[] data, int length) throws IOException {
__receivePacket.setData(data);
__receivePacket.setLength(length);
_socket_.receive(__receivePacket);
return __receivePacket.getLength();
}
/***
* Same as receive(data, data.length)
*
* @param data the buffer to receive the input
* @return the number of bytes
* @throws IOException on error
***/
public int receive(byte[] data) throws IOException {
return receive(data, data.length);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy