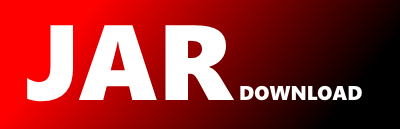
panda.net.pop3.POP3MessageInfo Maven / Gradle / Ivy
Show all versions of panda-nets Show documentation
package panda.net.pop3;
/***
* POP3MessageInfo is used to return information about messages stored on a POP3 server. Its fields
* are used to mean slightly different things depending on the information being returned.
*
* In response to a status command, number
contains the number of messages in the
* mailbox, size
contains the size of the mailbox in bytes, and
* identifier
is null.
*
* In response to a message listings, number
contains the message number,
* size
contains the size of the message in bytes, and identifier
is
* null.
*
* In response to unique identifier listings, number
contains the message number,
* size
is undefined, and identifier
contains the message's unique
* identifier.
***/
public final class POP3MessageInfo {
public int number;
public int size;
public String identifier;
/***
* Creates a POP3MessageInfo instance with number
and size
set to 0,
* and identifier
set to null.
***/
public POP3MessageInfo() {
this(0, null, 0);
}
/***
* Creates a POP3MessageInfo instance with number
set to num
,
* size
set to octets
, and identifier
set to null.
*
* @param num the number
* @param octets the size
***/
public POP3MessageInfo(int num, int octets) {
this(num, null, octets);
}
/***
* Creates a POP3MessageInfo instance with number
set to num
,
* size
undefined, and identifier
set to uid
.
*
* @param num the number
* @param uid the UID
***/
public POP3MessageInfo(int num, String uid) {
this(num, uid, -1);
}
private POP3MessageInfo(int num, String uid, int size) {
this.number = num;
this.size = size;
this.identifier = uid;
}
}