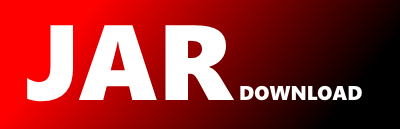
panda.tool.poi.xls.ESheet Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of panda-tool Show documentation
Show all versions of panda-tool Show documentation
Panda Tool contains some commonly used tools and source code generator for Panda Mvc. Can generate Entity/Query/Dao/Action class, Freemarker (HTML) template file.
package panda.tool.poi.xls;
import java.util.LinkedHashMap;
import java.util.Map;
import org.apache.poi.ss.usermodel.HeaderFooter;
public class ESheet {
private int index;
private String name;
private HeaderFooter header;
private HeaderFooter footer;
private Map strings = new LinkedHashMap();
private Map comments = new LinkedHashMap();
private Map textboxs = new LinkedHashMap();
/**
* @return the index
*/
public int getIndex() {
return index;
}
/**
* @param index the index to set
*/
public void setIndex(int index) {
this.index = index;
}
/**
* @return the name
*/
public String getName() {
return name;
}
/**
* @param name the name to set
*/
public void setName(String name) {
this.name = name;
}
/**
* @return the header
*/
public HeaderFooter getHeader() {
return header;
}
/**
* @param header the header to set
*/
public void setHeader(HeaderFooter header) {
this.header = header;
}
/**
* @return the footer
*/
public HeaderFooter getFooter() {
return footer;
}
/**
* @param footer the footer to set
*/
public void setFooter(HeaderFooter footer) {
this.footer = footer;
}
/**
* @return the strings
*/
public Map getStrings() {
return strings;
}
/**
* @param strings the strings to set
*/
public void setStrings(Map strings) {
this.strings = strings;
}
/**
* @return the comments
*/
public Map getComments() {
return comments;
}
/**
* @param comments the comments to set
*/
public void setComments(Map comments) {
this.comments = comments;
}
/**
* @return the textboxs
*/
public Map getTextboxs() {
return textboxs;
}
/**
* @param textboxs the textboxs to set
*/
public void setTextboxs(Map textboxs) {
this.textboxs = textboxs;
}
public String toString() {
return index + ": " + name;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy