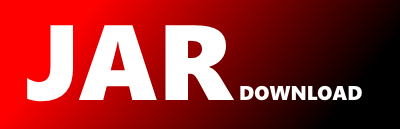
org.springframework.content.commons.utils.DomainObjectUtils Maven / Gradle / Ivy
package org.springframework.content.commons.utils;
import java.beans.PropertyDescriptor;
import java.lang.reflect.Field;
import org.springframework.beans.BeanWrapperImpl;
import org.springframework.core.annotation.AnnotationUtils;
public final class DomainObjectUtils {
private static boolean JAVAX_PERSISTENCE_ID_CLASS_PRESENT = false;
static {
try {
JAVAX_PERSISTENCE_ID_CLASS_PRESENT = DomainObjectUtils.class.getClassLoader().loadClass("javax.persistence.Id") != null;
} catch (ClassNotFoundException e) {}
}
private DomainObjectUtils() {}
public static final Object getId(Object entity) {
Object id = null;
if (JAVAX_PERSISTENCE_ID_CLASS_PRESENT && BeanUtils.hasFieldWithAnnotation(entity, javax.persistence.Id.class)) {
id = BeanUtils.getFieldWithAnnotation(entity, javax.persistence.Id.class);
if (id == null) {
PropertyDescriptor[] propertyDescriptors = new BeanWrapperImpl(entity).getPropertyDescriptors();
for (PropertyDescriptor propertyDescriptor : propertyDescriptors) {
if (AnnotationUtils.findAnnotation(propertyDescriptor.getReadMethod(), javax.persistence.Id.class) != null) {
return new BeanWrapperImpl(entity).getPropertyValue(propertyDescriptor.getName());
}
}
}
} else if (BeanUtils.hasFieldWithAnnotation(entity, org.springframework.data.annotation.Id.class)) {
id = BeanUtils.getFieldWithAnnotation(entity, org.springframework.data.annotation.Id.class);
if (id == null) {
PropertyDescriptor[] propertyDescriptors = new BeanWrapperImpl(entity).getPropertyDescriptors();
for (PropertyDescriptor propertyDescriptor : propertyDescriptors) {
if (AnnotationUtils.findAnnotation(propertyDescriptor.getReadMethod(), org.springframework.data.annotation.Id.class) != null) {
return new BeanWrapperImpl(entity).getPropertyValue(propertyDescriptor.getName());
}
}
}
}
return id;
}
public static final Field getIdField(Class> domainClass) {
if (JAVAX_PERSISTENCE_ID_CLASS_PRESENT && BeanUtils.findFieldWithAnnotation(domainClass, javax.persistence.Id.class) != null) {
return BeanUtils.findFieldWithAnnotation(domainClass, javax.persistence.Id.class);
} else if (BeanUtils.findFieldWithAnnotation(domainClass, org.springframework.data.annotation.Id.class) != null) {
return BeanUtils.findFieldWithAnnotation(domainClass, org.springframework.data.annotation.Id.class);
}
return null;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy