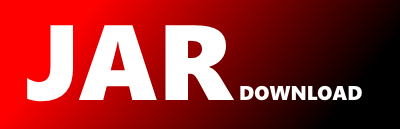
gov.nasa.worldwind.ogc.collada.ColladaMesh Maven / Gradle / Ivy
The newest version!
/*
* Copyright (C) 2012 United States Government as represented by the Administrator of the
* National Aeronautics and Space Administration.
* All Rights Reserved.
*/
package gov.nasa.worldwind.ogc.collada;
import java.util.*;
/**
* Represents the COLLADA mesh element and provides access to its contents.
*
* @author pabercrombie
* @version $Id: ColladaMesh.java 654 2012-06-25 04:15:52Z pabercrombie $
*/
public class ColladaMesh extends ColladaAbstractObject
{
protected List sources = new ArrayList();
protected List vertices = new ArrayList();
// Most meshes contain either triangles or lines. Lazily allocate these lists.
protected List triangles;
protected List lines;
public ColladaMesh(String ns)
{
super(ns);
}
public List getSources()
{
return this.sources;
}
public List getTriangles()
{
return this.triangles != null ? this.triangles : Collections.emptyList();
}
public List getLines()
{
return this.lines != null ? this.lines : Collections.emptyList();
}
public List getVertices()
{
return this.vertices;
}
@Override
public void setField(String keyName, Object value)
{
if (keyName.equals("vertices"))
{
this.vertices.add((ColladaVertices) value);
}
else if (keyName.equals("source"))
{
this.sources.add((ColladaSource) value);
}
else if (keyName.equals("triangles"))
{
if (this.triangles == null)
this.triangles = new ArrayList();
this.triangles.add((ColladaTriangles) value);
}
else if (keyName.equals("lines"))
{
if (this.lines == null)
this.lines = new ArrayList();
this.lines.add((ColladaLines) value);
}
else
{
super.setField(keyName, value);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy