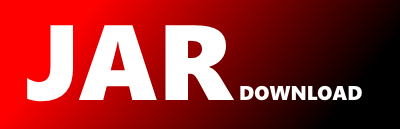
gov.nasa.worldwind.ogc.kml.impl.KMLTraversalContext Maven / Gradle / Ivy
The newest version!
/*
* Copyright (C) 2012 United States Government as represented by the Administrator of the
* National Aeronautics and Space Administration.
* All Rights Reserved.
*/
package gov.nasa.worldwind.ogc.kml.impl;
import gov.nasa.worldwind.ogc.kml.KMLRegion;
import gov.nasa.worldwind.util.Logging;
import java.util.*;
/**
* KMLTraversalContext
provides a suitcase of KML specific state used to render a hierarchy of KML
* features.
*
* @author tag
* @version $Id: KMLTraversalContext.java 1171 2013-02-11 21:45:02Z dcollins $
*/
public class KMLTraversalContext
{
/**
* The Deque
as this KML traversal context's Region stack. The region stack is used to implement
* Regions inheritance of from a KML containers to their descendant KML features.
*/
protected Deque regionStack = new ArrayDeque();
/**
* Indicates this KML traversal context's detail hint. Modifies the default relationship of KML scene resolution to
* screen resolution as viewing distance changes. Values greater than 0 increase the resolution. Values less than 0
* decrease the resolution. Initially 0.
*/
protected double detailHint;
/** Constructs a new KML traversal context in a default state, but otherwise does nothing. */
public KMLTraversalContext()
{
}
/**
* Initializes this KML traversal context to its default state. This should be called at the beginning of each frame
* to prepare this traversal context for the coming render pass.
*/
public void initialize()
{
this.regionStack.clear();
this.detailHint = 0.0;
}
/**
* Adds the specified region
to the top of this KML traversal context's Region stack. The specified
* region is returned by any subsequent calls to peekRegion
until either pushRegion
or
* popRegion
are called. The region
is removed from the stack by calling
* popRegion
.
*
* @param region the KML Region to add to the top of the stack.
*
* @throws IllegalArgumentException if region
is null
.
*/
public void pushRegion(KMLRegion region)
{
if (region == null)
{
String message = Logging.getMessage("nullValue.RegionIsNull");
Logging.logger().severe(message);
throw new IllegalArgumentException(message);
}
this.regionStack.push(region);
}
/**
* Returns the KML Region on the top of this KML traversal context's Region stack, or null
if the
* Region stack is empty. The Region on the top of the stack the last region added with a call to
* pushRegion
. This does not modify the contents of the stack.
*
* @return the Region on the top of this context's stack, or null
if the stack is empty.
*/
public KMLRegion peekRegion()
{
return this.regionStack.peek();
}
/**
* Removes the KML Region from the top of this KML traversal context's Region stack. This throws an exception if the
* Region stack is empty, otherwise this removes and returns the last Region added to the stack by a call to
* pushRegion
.
*
* @return the Region removed from the top of the stack.
*
* @throws NoSuchElementException if the Region stack is empty.
*/
public KMLRegion popRegion()
{
return this.regionStack.pop();
}
/**
* Indicates this KML traversal context's detail hint, which is described in {@link
* #setDetailHint(double)}
.
*
* @return the detail hint.
*
* @see #setDetailHint(double)
*/
public double getDetailHint()
{
return this.detailHint;
}
/**
* Specifies this KML traversal context's detail hint. The detail hint modifies the default relationship of KML
* scene resolution to screen resolution as the viewing distance changes. Values greater than 0 cause KML elements
* with a level of detail to appear at higher resolution at greater distances than normal, but at an increased
* performance cost. Values less than 0 decrease the default resolution at any given distance. The default value is
* 0. Values typically range between -0.5 and 0.5.
*
* @param detailHint the degree to modify the default relationship of KML scene resolution to screen resolution as
* viewing distance changes. Values greater than 0 increase the resolution. Values less than 0
* decrease the resolution. The default value is 0.
*/
public void setDetailHint(double detailHint)
{
this.detailHint = detailHint;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy