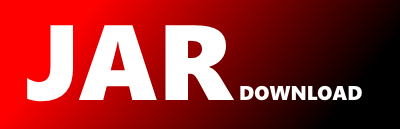
gov.nasa.worldwind.util.layertree.LayerTree Maven / Gradle / Ivy
The newest version!
/*
* Copyright (C) 2012 United States Government as represented by the Administrator of the
* National Aeronautics and Space Administration.
* All Rights Reserved.
*/
package gov.nasa.worldwind.util.layertree;
import gov.nasa.worldwind.avlist.AVKey;
import gov.nasa.worldwind.render.Offset;
import gov.nasa.worldwind.util.Logging;
import gov.nasa.worldwind.util.tree.*;
/**
* A Renderable
tree of {@link gov.nasa.worldwind.layers.Layer}
objects and their content. By
* default, a LayerTree
is created with a {@link LayerTreeModel}
, and a {@link
* gov.nasa.worldwind.util.tree.BasicTreeLayout}
that is configured for displaying a layer tree. Callers can
* specify the model to use either by specifying one during construction, or by calling {@link
* LayerTree#setModel(gov.nasa.worldwind.util.tree.TreeModel)}
. Once created, callers add layers to the tree
* using methods on LayerTreeModel
.
*
* @author dcollins
* @version $Id: LayerTree.java 1171 2013-02-11 21:45:02Z dcollins $
* @see LayerTreeModel
* @see LayerTreeNode
*/
public class LayerTree extends BasicTree
{
/** The default screen location: 20x140 pixels from the upper left screen corner. */
protected static final Offset DEFAULT_OFFSET = new Offset(20d, 140d, AVKey.PIXELS, AVKey.INSET_PIXELS);
/** The default frame image. Appears to the left of the frame title. */
protected static final String DEFAULT_FRAME_IMAGE = "images/layer-manager-64x64.png";
/** The default frame title: "Layers". */
protected static final String DEFAULT_FRAME_TITLE = "Layers";
/**
* Creates a new LayerTree
with an empty LayerTreeModel
and the default screen location.
* The tree's upper left corner is placed 20x140 pixels from the upper left screen corner.
*/
public LayerTree()
{
this.initialize(null, null);
}
/**
* Creates a new LayerTree
with the specified model
and the default screen location. The
* tree's upper left corner is placed 20x140 pixels from the upper left screen corner.
*
* @param model the tree model to use.
*
* @throws IllegalArgumentException if model
is null
.
*/
public LayerTree(LayerTreeModel model)
{
if (model == null)
{
String message = Logging.getMessage("nullValue.ModelIsNull");
Logging.logger().severe(message);
throw new IllegalArgumentException(message);
}
this.initialize(model, null);
}
/**
* Creates a new LayerTree
with an empty LayerTreeModel
and the specified screen
* location.
*
* @param offset the screen location of the tree's upper left corner, relative to the screen's upper left corner.
*
* @throws IllegalArgumentException if offset
is null
.
*/
public LayerTree(Offset offset)
{
if (offset == null)
{
String message = Logging.getMessage("nullValue.OffsetIsNull");
Logging.logger().severe(message);
throw new IllegalArgumentException(message);
}
this.initialize(null, offset);
}
/**
* Creates a new LayerTree
with the specified model
and the specified screen location.
*
* @param model the tree model to use.
* @param offset the screen location of the tree's upper left corner, relative to the screen's upper left corner.
*
* @throws IllegalArgumentException if model
is null
, or if offset
is
* null
.
*/
public LayerTree(LayerTreeModel model, Offset offset)
{
if (model == null)
{
String message = Logging.getMessage("nullValue.ModelIsNull");
Logging.logger().severe(message);
throw new IllegalArgumentException(message);
}
if (offset == null)
{
String message = Logging.getMessage("nullValue.OffsetIsNull");
Logging.logger().severe(message);
throw new IllegalArgumentException(message);
}
this.initialize(model, offset);
}
/**
* Initializes this tree with the specified model
and offset
. This configures the tree's
* model, its layout, and expands the path to the root node. If either parameter is null
this uses a
* suitable default.
*
* @param model this tree's model to use, or null
to create a new LayerTreeModel
.
* @param offset the screen location of this tree's upper left corner, or null
to use the default.
*/
protected void initialize(LayerTreeModel model, Offset offset)
{
if (model == null)
model = this.createTreeModel();
this.setModel(model);
this.setLayout(this.createTreeLayout(offset));
this.expandPath(this.getModel().getRoot().getPath());
}
/**
* Returns a new LayerTreeModel
. Called from initialize
when no model is specified.
*
* @return a new LayerTreeModel
.
*/
protected LayerTreeModel createTreeModel()
{
return new LayerTreeModel();
}
/**
* Returns a new TreeLayout
suitable for displaying the layer tree on a WorldWindow
. If
* the offset
is null
this the default value.
*
* @param offset the screen location of this tree's upper left corner, or null
to use the default.
*
* @return new TreeLayout
.
*/
protected TreeLayout createTreeLayout(Offset offset)
{
if (offset == null)
offset = DEFAULT_OFFSET;
BasicTreeLayout layout = new BasicTreeLayout(this, offset);
layout.getFrame().setFrameTitle(DEFAULT_FRAME_TITLE);
layout.getFrame().setIconImageSource(DEFAULT_FRAME_IMAGE);
BasicTreeAttributes attributes = new BasicTreeAttributes();
attributes.setRootVisible(false);
layout.setAttributes(attributes);
BasicFrameAttributes frameAttributes = new BasicFrameAttributes();
frameAttributes.setBackgroundOpacity(0.7);
layout.getFrame().setAttributes(frameAttributes);
BasicTreeAttributes highlightAttributes = new BasicTreeAttributes(attributes);
layout.setHighlightAttributes(highlightAttributes);
BasicFrameAttributes highlightFrameAttributes = new BasicFrameAttributes(frameAttributes);
highlightFrameAttributes.setForegroundOpacity(1.0);
highlightFrameAttributes.setBackgroundOpacity(1.0);
layout.getFrame().setHighlightAttributes(highlightFrameAttributes);
return layout;
}
/** {@inheritDoc} */
public LayerTreeModel getModel()
{
return (LayerTreeModel) super.getModel();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy